TypeError: タプル(Int ではない) をタプルに連結することしかできません
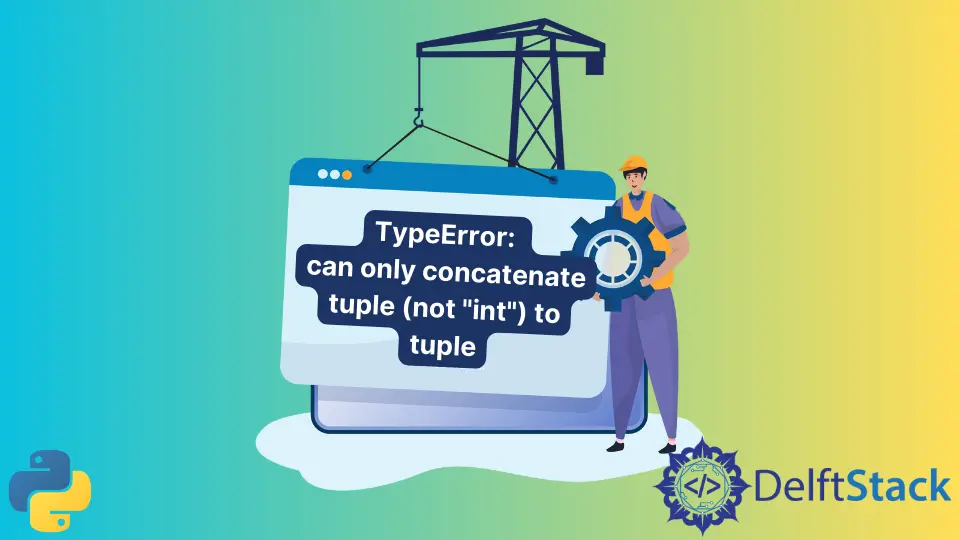
Python プログラミング言語では、tuple
はコンマ ,
で区切られたオブジェクトのコレクションを格納するために使用できるデータ構造です。 tuple
は不変です。つまり、そのオブジェクトを変更することはできません。
タプルを作成するには、タプルの名前と通常の括弧 ( )
が必要で、コンマ ,
で区切られたオブジェクトを追加します。
タプルの構文:
my_tpl = (1, 2, 3, 4, 5, 6)
print(type(my_tpl)) # print the type of my_tpl
print(my_tpl)
出力:
<class 'tuple'>
(1, 2, 3, 4, 5, 6)
単一のオブジェクトでタプルを作成する
上記のプログラムでタプルの作成についてはすでに理解しましたが、それは複数のオブジェクトを持つタプルでした。 タプルの作成は、他のものとは少し異なる場合があります。
コード例:
my_tpl = 1
print(type(my_tpl))
print(my_tpl)
出力:
<class 'int'>
1
これは tuple
ではなく int
クラスに属します。その理由は、int
と tuple
が異なるため、タプルのオブジェクトの後にカンマ ,
を使用します。
コード例:
my_tpl = (1,)
print(type(my_tpl))
print(my_tpl)
出力:
<class 'tuple'>
(1,)
単一のオブジェクトを含むタプルを定義しました。
Python の TypeError: Can Only Concatenate Tuple (Not "Int") To Tuple
を修正
この一般的なエラーは、タプル以外のデータ型の値を連結しようとしたときに発生します。 タプルに整数を追加すると、このエラーが発生する可能性があります。
このエラーが発生する理由と修正方法を見てみましょう。
コード例:
nums_tpl = (1, 2, 3, 4, 5) # Tuple
num_int = 6 # Integer
# Concatinating a tuple and an integer
concatinate = nums_tpl + num_int
print(concatinate)
出力:
TypeError: can only concatenate tuple (not "int") to tuple
整数をタプルに連結することは Python では許可されていないため、TypeError
が発生します。
TypeError: can only concatenate tuple (not "int") to tuple
を修正するには、2つのタプルを連結できますが、他のデータ型のタプルは連結できないため、整数の代わりにタプルを使用できます。
コード例:
nums_tpl = (1, 2, 3, 4, 5) # Tuple
num_int = (6,) # Tuple
# Concatinating two tuples
concatinate = nums_tpl + num_int
print(concatinate)
出力:
(1, 2, 3, 4, 5, 6)
ご覧のとおり、TypeError
は、タプルと整数の代わりに 2つのタプルを連結することで修正されます。
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn関連記事 - Python Error
- AttributeError の解決: 'list' オブジェクト属性 'append' は読み取り専用です
- AttributeError の解決: Python で 'Nonetype' オブジェクトに属性 'Group' がありません
- AttributeError: 'generator' オブジェクトに Python の 'next' 属性がありません
- AttributeError: 'numpy.ndarray' オブジェクトに Python の 'Append' 属性がありません
- AttributeError: Int オブジェクトに属性がありません
- AttributeError: Python で 'Dict' オブジェクトに属性 'Append' がありません