Python でテキストから N-gram を作成する
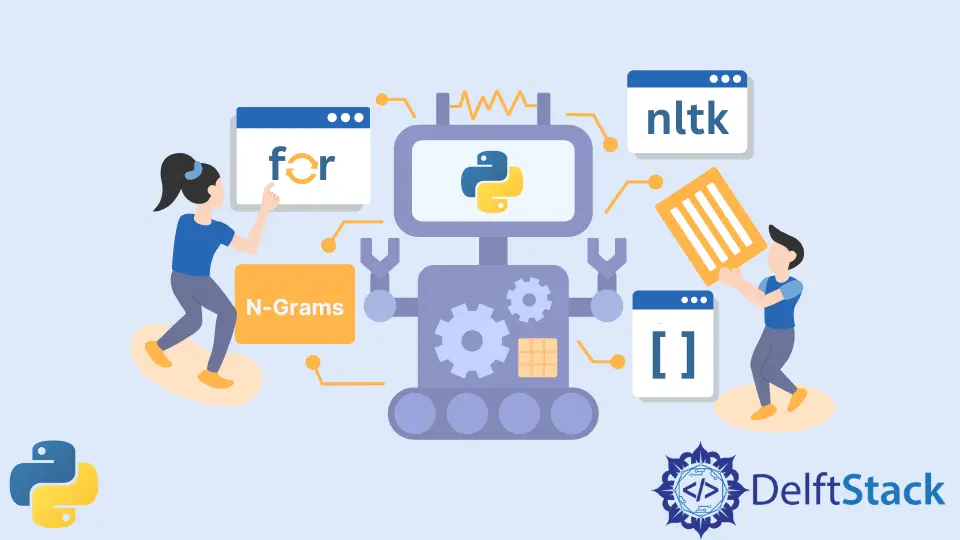
計算言語学では、n-gram は言語処理と文脈分析および意味分析にとって重要です。 それらは、トークンの文字列から互いに隣接する連続した連続した単語のシーケンスです。
人気のあるものは、ユニグラム、バイグラム、およびトリグラムであり、それらは効果的であり、n>3
の場合、データ スパース性 が存在する可能性があります。
この記事では、機能とライブラリを使用して Python で n-gram を作成する方法について説明します。
for
ループを使用して Python でテキストから N-gram を作成する
n-grams を含むリストを返す、テキストと n
値を取る ngrams
関数を効果的に作成できます。
関数を作成するには、テキストを分割し、n-gram を格納する空のリスト (output
) を作成します。 for
ループを使用して splitInput
リストをループし、すべての要素を調べます。
単語 (トークン) は output
リストに追加されます。
def ngrams(input, num):
splitInput = input.split(" ")
output = []
for i in range(len(splitInput) - num + 1):
output.append(splitInput[i : i + num])
return output
text = "Welcome to the abode, and more importantly, our in-house exceptional cooking service which is close to the Burj Khalifa"
print(ngrams(text, 3))
コードの出力
[['Welcome', 'to', 'the'], ['to', 'the', 'abode,'], ['the', 'abode,', 'and'], ['abode,', 'and', 'more'], ['and', 'more', 'importantly,'], ['more', 'importantly,', 'our'], ['importantly,', 'our', 'in-house'], ['our', 'in-house', 'exceptional'], ['in-house', 'exceptional', 'cooking'], ['exceptional', 'cooking', 'service'], ['cooking', 'service', 'which'], ['service', 'which', 'is'], ['which', 'is', 'close'], ['is', 'close', 'to'], ['close', 'to', 'the'], ['to', 'the', 'Burj'], ['the', 'Burj', 'Khalifa']]
nltk
を使用して Python でテキストから N-Grams を作成する
NLTK ライブラリ は、テキスト処理やトークン化などに重要なリソースへの使いやすいインターフェイスを提供する自然言語ツールキットです。 nltk
をインストールするには、以下の pip
コマンドを使用できます。
pip install nltk
潜在的な問題を示すために、word_tokenize()
メソッドを使用してみましょう。これは、より詳細なコードの記述に移る前に、NLTK が推奨する単語トークナイザーを使用して、渡したテキストのトークン化されたコピーを作成するのに役立ちます。
import nltk
text = "well the money has finally come"
tokens = nltk.word_tokenize(text)
コードの出力:
Traceback (most recent call last):
File "c:\Users\akinl\Documents\Python\SFTP\n-gram-two.py", line 4, in <module>
tokens = nltk.word_tokenize(text)
File "C:\Python310\lib\site-packages\nltk\tokenize\__init__.py", line 129, in word_tokenize
sentences = [text] if preserve_line else sent_tokenize(text, language)
File "C:\Python310\lib\site-packages\nltk\tokenize\__init__.py", line 106, in sent_tokenize
tokenizer = load(f"tokenizers/punkt/{language}.pickle")
File "C:\Python310\lib\site-packages\nltk\data.py", line 750, in load
opened_resource = _open(resource_url)
File "C:\Python310\lib\site-packages\nltk\data.py", line 876, in _open
return find(path_, path + [""]).open()
File "C:\Python310\lib\site-packages\nltk\data.py", line 583, in find
raise LookupError(resource_not_found)
LookupError:
**********************************************************************
Resource [93mpunkt[0m not found.
Please use the NLTK Downloader to obtain the resource:
[31m>>> import nltk
>>> nltk.download('punkt')
[0m
For more information see: https://www.nltk.org/data.html
Attempted to load [93mtokenizers/punkt/english.pickle[0m
Searched in:
- 'C:\\Users\\akinl/nltk_data'
- 'C:\\Python310\\nltk_data'
- 'C:\\Python310\\share\\nltk_data'
- 'C:\\Python310\\lib\\nltk_data'
- 'C:\\Users\\akinl\\AppData\\Roaming\\nltk_data'
- 'C:\\nltk_data'
- 'D:\\nltk_data'
- 'E:\\nltk_data'
- ''
**********************************************************************
上記のエラー メッセージと問題の理由は、NLTK ライブラリが一部のメソッドで 特定のデータ を必要とするためです。特にこれが初めての使用である場合は、データをダウンロードしていません。 したがって、2つのデータ モジュール punkt
と averaged_perceptron_tagger
をダウンロードするには、NLTK ダウンローダーが必要です。
データは、たとえば words()
などのメソッドを使用する場合に使用できます。 データをダウンロードするには、Python スクリプトでデータを実行する必要がある場合、download()
メソッドが必要です。
Python ファイルを作成し、以下のコードを実行して問題を解決できます。
import nltk
nltk.download("punkt")
nltk.download("averaged_perceptron_tagger")
または、コマンド ライン インターフェイスから次のコマンドを実行します。
python -m nltk.downloader punkt
python -m nltk.downloader averaged_perceptron_tagger
コード例:
import nltk
text = "well the money has finally come"
tokens = nltk.word_tokenize(text)
textBigGrams = nltk.bigrams(tokens)
textTriGrams = nltk.trigrams(tokens)
print(list(textBigGrams), list(textTriGrams))
コードの出力:
[('well', 'the'), ('the', 'money'), ('money', 'has'), ('has', 'finally'), ('finally', 'come')] [('well', 'the', 'money'), ('the', 'money', 'has'), ('money', 'has', 'finally'), ('has', 'finally', 'come')]
コード例:
import nltk
text = "well the money has finally come"
tokens = nltk.word_tokenize(text)
textBigGrams = nltk.bigrams(tokens)
textTriGrams = nltk.trigrams(tokens)
print("The Bigrams of the Text are")
print(*map(" ".join, textBigGrams), sep=", ")
print("The Trigrams of the Text are")
print(*map(" ".join, textTriGrams), sep=", ")
コードの出力:
The Bigrams of the Text are
well the, the money, money has, has finally, finally come
The Trigrams of the Text are
well the money, the money has, money has finally, has finally come
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn