Python의 텍스트에서 N-그램 만들기
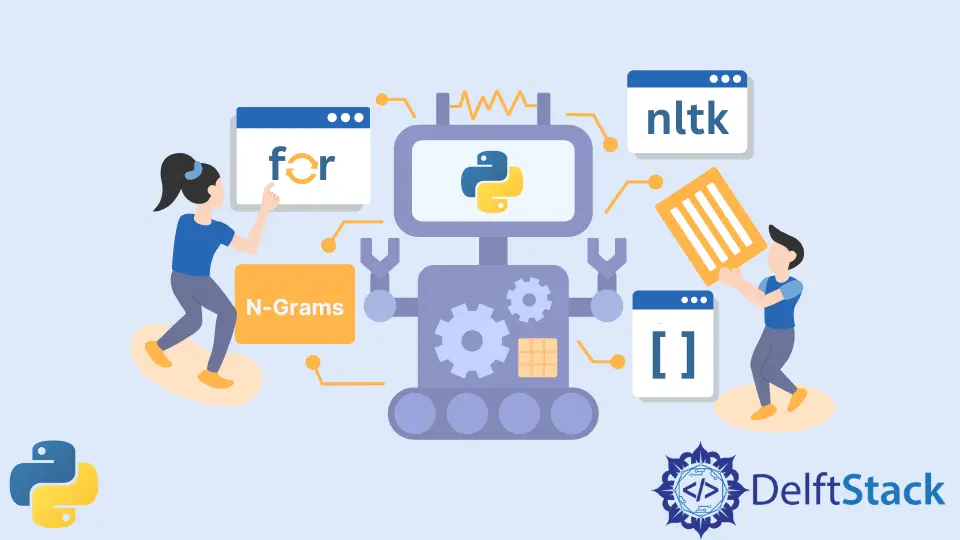
전산 언어학에서 n-gram은 언어 처리와 문맥 및 의미 분석에 중요합니다. 일련의 토큰에서 서로 인접한 연속적이고 연속적인 단어 시퀀스입니다.
인기 있는 것은 unigram, bigrams 및 trigram이며 효과적이며 n>3
인 경우 데이터 희소성이 있을 수 있습니다.
이 기사에서는 기능 및 라이브러리를 사용하여 Python에서 n-gram을 만드는 방법에 대해 설명합니다.
for
루프를 사용하여 Python의 텍스트에서 N-그램 만들기
텍스트와 n-gram을 포함하는 목록을 반환하는 n
값을 사용하는 ngrams
함수를 효과적으로 만들 수 있습니다.
함수를 만들기 위해 텍스트를 분할하고 n-gram을 저장할 빈 목록(output
)을 만들 수 있습니다. for
루프를 사용하여 splitInput
목록을 반복하여 모든 요소를 살펴봅니다.
그러면 단어(토큰)가 output
목록에 추가됩니다.
def ngrams(input, num):
splitInput = input.split(" ")
output = []
for i in range(len(splitInput) - num + 1):
output.append(splitInput[i : i + num])
return output
text = "Welcome to the abode, and more importantly, our in-house exceptional cooking service which is close to the Burj Khalifa"
print(ngrams(text, 3))
코드의 출력
[['Welcome', 'to', 'the'], ['to', 'the', 'abode,'], ['the', 'abode,', 'and'], ['abode,', 'and', 'more'], ['and', 'more', 'importantly,'], ['more', 'importantly,', 'our'], ['importantly,', 'our', 'in-house'], ['our', 'in-house', 'exceptional'], ['in-house', 'exceptional', 'cooking'], ['exceptional', 'cooking', 'service'], ['cooking', 'service', 'which'], ['service', 'which', 'is'], ['which', 'is', 'close'], ['is', 'close', 'to'], ['close', 'to', 'the'], ['to', 'the', 'Burj'], ['the', 'Burj', 'Khalifa']]
nltk
를 사용하여 Python의 텍스트에서 N-그램 만들기
NLTK 라이브러리는 특히 텍스트 처리 및 토큰화에 중요한 리소스에 대한 사용하기 쉬운 인터페이스를 제공하는 자연어 툴킷입니다. nltk
를 설치하려면 아래 pip
명령을 사용할 수 있습니다.
pip install nltk
잠재적인 문제를 보여주기 위해 word_tokenize()
메서드를 사용하겠습니다. 이 메서드는 더 자세한 코드 작성으로 이동하기 전에 NLTK의 권장 단어 토크나이저를 사용하여 전달하는 텍스트의 토큰화된 복사본을 만드는 데 도움이 됩니다.
import nltk
text = "well the money has finally come"
tokens = nltk.word_tokenize(text)
코드 출력:
Traceback (most recent call last):
File "c:\Users\akinl\Documents\Python\SFTP\n-gram-two.py", line 4, in <module>
tokens = nltk.word_tokenize(text)
File "C:\Python310\lib\site-packages\nltk\tokenize\__init__.py", line 129, in word_tokenize
sentences = [text] if preserve_line else sent_tokenize(text, language)
File "C:\Python310\lib\site-packages\nltk\tokenize\__init__.py", line 106, in sent_tokenize
tokenizer = load(f"tokenizers/punkt/{language}.pickle")
File "C:\Python310\lib\site-packages\nltk\data.py", line 750, in load
opened_resource = _open(resource_url)
File "C:\Python310\lib\site-packages\nltk\data.py", line 876, in _open
return find(path_, path + [""]).open()
File "C:\Python310\lib\site-packages\nltk\data.py", line 583, in find
raise LookupError(resource_not_found)
LookupError:
**********************************************************************
Resource [93mpunkt[0m not found.
Please use the NLTK Downloader to obtain the resource:
[31m>>> import nltk
>>> nltk.download('punkt')
[0m
For more information see: https://www.nltk.org/data.html
Attempted to load [93mtokenizers/punkt/english.pickle[0m
Searched in:
- 'C:\\Users\\akinl/nltk_data'
- 'C:\\Python310\\nltk_data'
- 'C:\\Python310\\share\\nltk_data'
- 'C:\\Python310\\lib\\nltk_data'
- 'C:\\Users\\akinl\\AppData\\Roaming\\nltk_data'
- 'C:\\nltk_data'
- 'D:\\nltk_data'
- 'E:\\nltk_data'
- ''
**********************************************************************
위의 오류 메시지 및 문제의 원인은 NLTK 라이브러리가 일부 메서드에 대해 특정 데이터를 필요로 하고 특히 이것이 처음 사용하는 경우 데이터를 다운로드하지 않았기 때문입니다. 따라서 punkt
및 averaged_perceptron_tagger
라는 두 개의 데이터 모듈을 다운로드하려면 NLTK 다운로더가 필요합니다.
예를 들어 words()
와 같은 메서드를 사용할 때 데이터를 사용할 수 있습니다. 데이터를 다운로드하려면 Python 스크립트를 통해 실행해야 하는 경우 download()
메서드가 필요합니다.
Python 파일을 만들고 아래 코드를 실행하여 문제를 해결할 수 있습니다.
import nltk
nltk.download("punkt")
nltk.download("averaged_perceptron_tagger")
또는 명령줄 인터페이스를 통해 다음 명령을 실행합니다.
python -m nltk.downloader punkt
python -m nltk.downloader averaged_perceptron_tagger
예제 코드:
import nltk
text = "well the money has finally come"
tokens = nltk.word_tokenize(text)
textBigGrams = nltk.bigrams(tokens)
textTriGrams = nltk.trigrams(tokens)
print(list(textBigGrams), list(textTriGrams))
코드 출력:
[('well', 'the'), ('the', 'money'), ('money', 'has'), ('has', 'finally'), ('finally', 'come')] [('well', 'the', 'money'), ('the', 'money', 'has'), ('money', 'has', 'finally'), ('has', 'finally', 'come')]
예제 코드:
import nltk
text = "well the money has finally come"
tokens = nltk.word_tokenize(text)
textBigGrams = nltk.bigrams(tokens)
textTriGrams = nltk.trigrams(tokens)
print("The Bigrams of the Text are")
print(*map(" ".join, textBigGrams), sep=", ")
print("The Trigrams of the Text are")
print(*map(" ".join, textTriGrams), sep=", ")
코드 출력:
The Bigrams of the Text are
well the, the money, money has, has finally, finally come
The Trigrams of the Text are
well the money, the money has, money has finally, has finally come
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn