Pandas DataFrame シリーズからリストを取得
Luqman Khan
2022年4月14日
Pandas
Pandas DataFrame
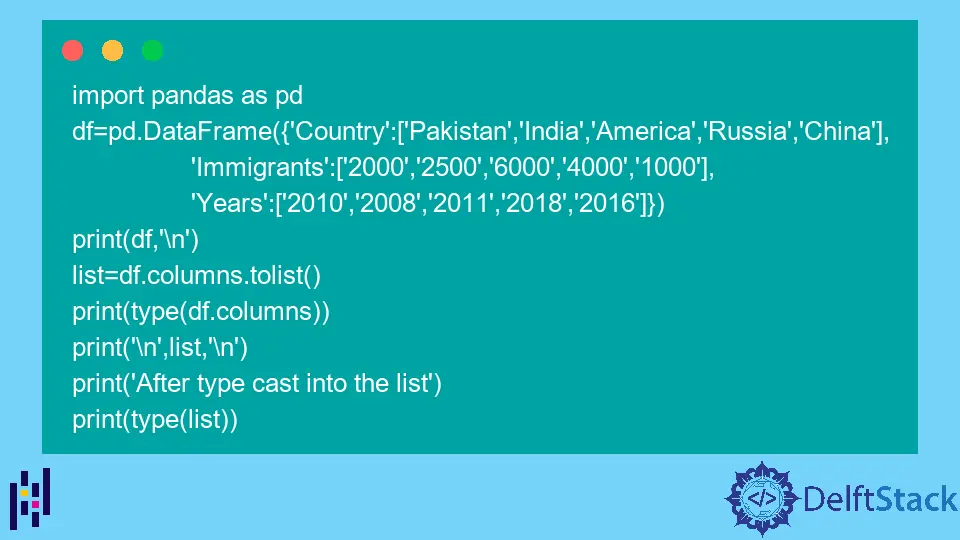
Python は、主に Python パッケージが原因で、データ分析でよく知られている言語です。Pandas は、データの分析をはるかに簡単にするのに役立つパッケージの 1つです。
Pandas の tolist()
メソッドは、シリーズを Python のシリーズまたは組み込みリストに変換します。デフォルトでは、シリーズは pandas.core.series.Series
データ型と tolist()
メソッドのタイプであり、データのリストに変換されます。
tolist()
メソッドを使用して、Pandas DataFrame シリーズからリストを取得する
この記事では、pandas DataFrame 列からリストを取得する方法について説明します。まず、CSV ファイルを Pandas DataFrame に読み込みます。
import pandas as pd
# read csv file
df = pd.read_csv("home_price.csv")
# display 3 rows
df = df.head(3)
print(df)
出力:
Area Home price
0 1000 10000
1 1200 12000
2 1300 13000
次に、tolist()
が役立つことがわかっているので、列から値を抽出してリストに変換します。
list1 = df["Home price"].values.tolist()
print("extract the value of series and converting into the list")
print(list1)
出力:
extract the value of series and converting into the list
[10000, 12000, 13000, 14000, 15000]
このリストは、Python で最も一般的なデータ構造の 1つである、順序付けられた柔軟な Python コンテナーです。要素は角かっこ []
に挿入され、コンマで区切られてリストが作成されます。リストには重複する値を含めることができます。そのため、主にデータセットでリストを使用しています。
import numpy as np
import pandas as pd
# read csv file
df = pd.read_csv("home_price.csv")
# extract the value of series and converting into the list
list1 = df["Home price"].values.tolist()
list1 = np.array(list1)
# type casting in list data type
updated = list(list1 * 1.5)
print("after include 1.5 % tax\n")
print(updated, "new home price")
df["Home price"] = updated
# create new csv
df.to_csv("home prices after 1 year.csv")
df2 = pd.read_csv("home prices after 1 year.csv")
print(df2)
この場合、現在の価格は 1.5
税で引き上げられます。次に、updated
list という名前のリストを作成し、既存の列を更新します。さらに、to_csv()
メソッドを使用して新しい CSV ファイルを作成します。
出力:
after include 1.5 % tax
[15000.0, 18000.0, 19500.0, 21000.0, 22500.0] new home price
Unnamed: 0 Area Home price
0 0 1000 15000.0
1 1 1200 18000.0
2 2 1300 19500.0
3 3 1400 21000.0
4 4 1500 22500.0
別の簡単な例を考えてみましょう。
import pandas as pd
df = pd.DataFrame(
{
"Country": ["Pakistan", "India", "America", "Russia", "China"],
"Immigrants": ["2000", "2500", "6000", "4000", "1000"],
"Years": ["2010", "2008", "2011", "2018", "2016"],
}
)
print(df, "\n")
list = df.columns.tolist()
print(type(df.columns))
print("\n", list, "\n")
print("After type cast into the list")
print(type(list))
系列のデータ型が tolist()
によって変更され、Dataframe のすべての列を含むリストが取得されたことに注意してください。
出力:
Country Immigrants Years
0 Pakistan 2000 2010
1 India 2500 2008
2 America 6000 2011
3 Russia 4000 2018
4 China 1000 2016
<class 'pandas.core.indexes.base.Index'>
['Country', 'Immigrants', 'Years']
After type cast into the list
<class 'list'>
すべてのコードはここにあります。
import numpy as np
import pandas as pd
# read csv file
df = pd.read_csv("home_price.csv")
# display 3 rows
df = df.head(3)
print(df)
list1 = df["Home price"].values.tolist()
print("extract the value of series and converting into the list")
print(list1)
# another example
# read csv file
df = pd.read_csv("home_price.csv")
# extract the value of series and converting into the list
list1 = df["Home price"].values.tolist()
list1 = np.array(list1)
# type casting in list data type
updated = list(list1 * 1.5)
print("after include 1.5 % tax\n")
print(updated, "new home price")
df["Home price"] = updated
# create new csv
df.to_csv("home prices after 1 year.csv")
df2 = pd.read_csv("home prices after 1 year.csv")
print(df2)
# another example
df = pd.DataFrame(
{
"Country": ["Pakistan", "India", "America", "Russia", "China"],
"Immigrants": ["2000", "2500", "6000", "4000", "1000"],
"Years": ["2010", "2008", "2011", "2018", "2016"],
}
)
print(df, "\n")
list = df.columns.tolist()
print(type(df.columns))
print("\n", list, "\n")
print("After type cast into the list")
print(type(list))
出力:
Area Home price
0 1000 10000
1 1200 12000
2 1300 13000
extract the value of series and converting into the list
[10000, 12000, 13000]
after include 1.5 % tax
[15000.0, 18000.0, 19500.0, 21000.0, 22500.0] new home price
Unnamed: 0 Area Home price
0 0 1000 15000.0
1 1 1200 18000.0
2 2 1300 19500.0
3 3 1400 21000.0
4 4 1500 22500.0
Country Immigrants Years
0 Pakistan 2000 2010
1 India 2500 2008
2 America 6000 2011
3 Russia 4000 2018
4 China 1000 2016
<class 'pandas.core.indexes.base.Index'>
['Country', 'Immigrants', 'Years']
After type cast into the list
<class 'list'>
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe