Pandas は他のカラムに基づいてカラムを作成する
Suraj Joshi
2023年1月30日
Pandas
Pandas DataFrame Column
- 要素別操作を使用して他のカラムの値に基づいて Pandas DataFrame に新しいカラムを作成する
-
DataFrame.apply()
メソッドを使って他のカラムの値を元に Pandas DataFrame に新しいカラムを作成する
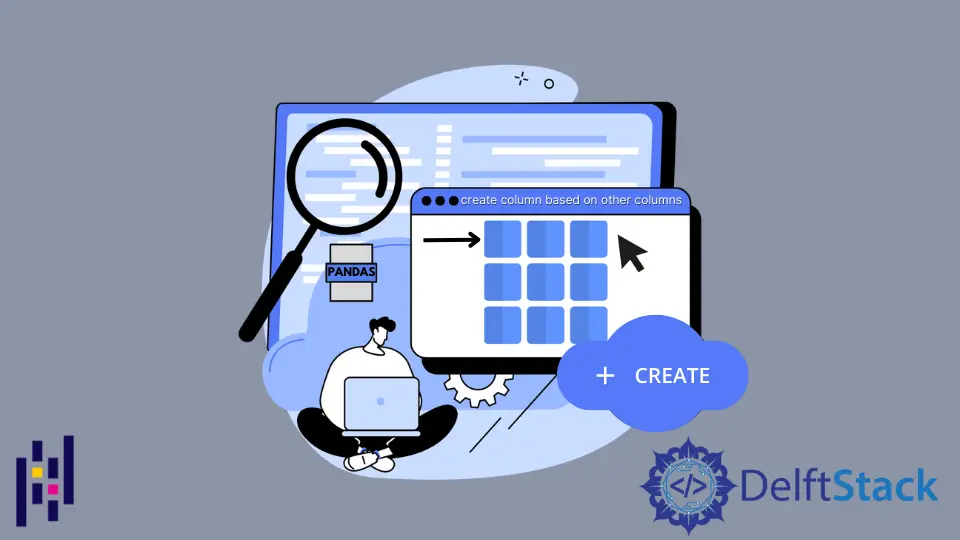
このチュートリアルでは、列の各要素に関数を適用したり、DataFrame.apply()
メソッドを使用して、DataFrame 内の他の列の値に基づいて Pandas DataFrame 内に新しい列を作成する方法を紹介します。
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": [300, 400, 350, 100, 1000, 400],
"Discount(%)": [10, 15, 5, 0, 2, 7],
}
)
print(items_df)
出力:
Id Name Cost Discount(%)
0 302 Watch 300 10
1 504 Camera 400 15
2 708 Phone 350 5
3 103 Shoes 100 0
4 343 Laptop 1000 2
5 565 Bed 400 7
上記のコードスニペットに表示されている DataFrame を使用して、DataFrame 内の他の列の値に基づいて Pandas DataFrame 内に新しい列を作成する方法を示します。
要素別操作を使用して他のカラムの値に基づいて Pandas DataFrame に新しいカラムを作成する
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Actual Price": [300, 400, 350, 100, 1000, 400],
"Discount(%)": [10, 15, 5, 0, 2, 7],
}
)
print("Initial DataFrame:")
print(items_df, "\n")
items_df["Final Price"] = items_df["Actual Price"] - (
(items_df["Discount(%)"] / 100) * items_df["Actual Price"]
)
print("DataFrame after addition of new column")
print(items_df, "\n")
出力:
Initial DataFrame:
Id Name Actual Price Discount(%)
0 302 Watch 300 10
1 504 Camera 400 15
2 708 Phone 350 5
3 103 Shoes 100 0
4 343 Laptop 1000 2
5 565 Bed 400 7
DataFrame after addition of new column
Id Name Actual Price Discount(%) Final Price
0 302 Watch 300 10 270.0
1 504 Camera 400 15 340.0
2 708 Phone 350 5 332.5
3 103 Shoes 100 0 100.0
4 343 Laptop 1000 2 980.0
5 565 Bed 400 7 372.0
DataFrame の Actual Price
カラムから割引額を差し引くことで、各商品の最終価格を計算します。そして、最終価格の値の Series
を DataFrame items_df
の Final Price
カラムに割り当てます。
DataFrame.apply()
メソッドを使って他のカラムの値を元に Pandas DataFrame に新しいカラムを作成する
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Actual_Price": [300, 400, 350, 100, 1000, 400],
"Discount_Percentage": [10, 15, 5, 0, 2, 7],
}
)
print("Initial DataFrame:")
print(items_df, "\n")
items_df["Final Price"] = items_df.apply(
lambda row: row.Actual_Price - ((row.Discount_Percentage / 100) * row.Actual_Price),
axis=1,
)
print("DataFrame after addition of new column")
print(items_df, "\n")
出力:
Initial DataFrame:
Id Name Actual_Price Discount_Percentage
0 302 Watch 300 10
1 504 Camera 400 15
2 708 Phone 350 5
3 103 Shoes 100 0
4 343 Laptop 1000 2
5 565 Bed 400 7
DataFrame after addition of new column
Id Name Actual_Price Discount_Percentage Final Price
0 302 Watch 300 10 270.0
1 504 Camera 400 15 340.0
2 708 Phone 350 5 332.5
3 103 Shoes 100 0 100.0
4 343 Laptop 1000 2 980.0
5 565 Bed 400 7 372.0
これは apply()
メソッドで定義されたラムダ関数を DataFrame items_df
の各行に適用し、最終的に結果の系列を DataFrame items_df
の Final Price
列に代入します。
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn