NumPy 配列を Pandas DataFrame に変換する
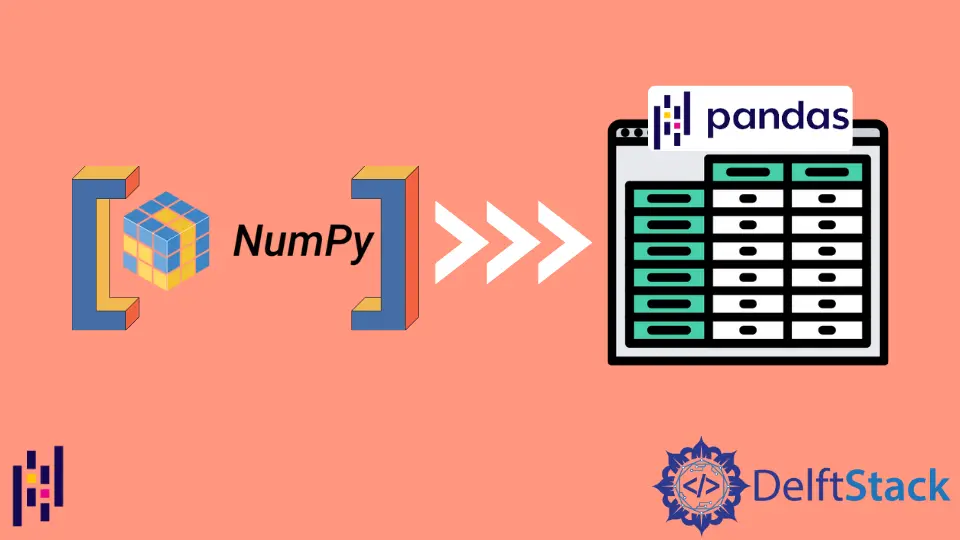
このチュートリアルでは、pandas.DataFrame()
メソッドを使って numpy 配列を Pandas DataFrame に変換する方法を説明します。
pandas.DataFrame()
メソッドに numpy 配列を渡して、NumPy 配列から Pandas DataFrame を生成します。また、DataFrame のカラム名と行のインデックスを指定することもできます。
pandas.DataFrame()
メソッドを使って NumPy 配列を Pandas DataFrame に変換する
NumPy 配列を pandas.DataFrame()
メソッドに渡して、NumPy 配列から DataFrame を生成します。
from numpy import random
import pandas as pd
random.seed(5)
random.randint(100, size=(3, 5))
data_array = random.randint(100, size=(4, 3))
print("NumPy Data Array is:")
print(data_array)
print("")
data_df = pd.DataFrame(data_array)
print("The DataFrame generated from the NumPy array is:")
print(data_df)
出力:
NumPy Data Array is:
[[27 44 77]
[75 65 47]
[30 84 86]
[18 9 41]]
The DataFrame generated from the NumPy array is:
0 1 2
0 27 44 77
1 75 65 47
2 30 84 86
3 18 9 41
まず、サイズ (4,3)
、4 行 3 列のランダムな配列を作成します。この配列を pandas.DataFrame()
メソッドに引数として渡します。デフォルトでは、pandas.DataFrame()
メソッドはデフォルトのカラム名と行インデックスを挿入します。
pandas.DataFrame()
メソッドの index
と columns
パラメータを用いて列名と行のインデックスを設定することもできます。
from numpy import random
import pandas as pd
random.seed(5)
random.randint(100, size=(3, 5))
data_array = random.randint(100, size=(4, 3))
row_indices = ["Row_1", "Row_2", "Row_3", "Row_4"]
column_names = ["Column_1", "Column_2", "Column_3"]
print("NumPy Data Array is:")
print(data_array)
print("")
data_df = pd.DataFrame(data_array, index=row_indices, columns=column_names)
print("The DataFrame generated from the NumPy array is:")
print(data_df)
出力:
NumPy Data Array is:
[[27 44 77]
[75 65 47]
[30 84 86]
[18 9 41]]
The DataFrame generated from the NumPy array is:
Column_1 Column_2 Column_3
Row_1 27 44 77
Row_2 75 65 47
Row_3 30 84 86
Row_4 18 9 41
ここでは、index
の値を row_indices
に設定します。同様に、columns
の値を各列の名前を含むリスト column_names
に設定することで、列名を代入します。
場合によっては、NumPy の配列自体が行のインデックスと列名を保持していることもあります。そこで、配列のスライスを利用して、配列からデータ、行インデックス、列名を抽出します。
import numpy as np
import pandas as pd
marks_array = np.array(
[["", "Mathematics", "Economics"], ["Sunny", 25, 23], ["Alice", 23, 24]]
)
print("NumPy Data Array is:")
print(marks_array)
print("")
row_indices = marks_array[1:, 0]
column_names = marks_array[0, 1:]
data_df = pd.DataFrame(
data=np.int_(marks_array[1:, 1:]), index=row_indices, columns=column_names
)
print("The DataFrame generated from the NumPy array is:")
print(data_df)
出力:
NumPy Data Array is:
[['' 'Mathematics' 'Economics']
['Sunny' '25' '23']
['Alice' '23' '24']]
The DataFrame generated from the NumPy array is:
Mathematics Economics
Sunny 25 23
Alice 23 24
NumPy 配列自体に行インデックスとカラム名があります。1 行目と 1 列目以降の値をすべて選択して data
引数として pandas.DataFrame()
関数に渡し、2 行目から 1 列目の値をすべて選択して index
引数として渡します。同様に、2 番目の列から最初の行の値をすべて選択して columns
引数として渡し、列名を設定します。
numpy.array()
は整数値を文字列に変換しながら NumPy 配列を作成し、配列と同じデータ形式であることを保証します。データの値を integer
型に変換するには numpy.int_()
関数を利用します。
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn