GoLang 文字列比較
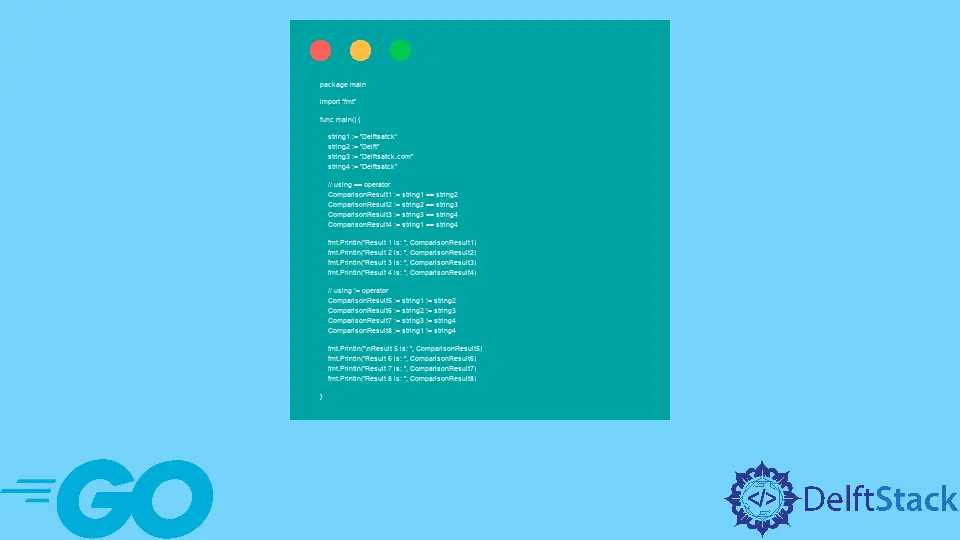
このチュートリアルでは、GoLang で文字列を比較する方法を示します。
GoLang には文字列を比較する 2つの方法があります。組み込みメソッド Compare()
と、もう 1つは GoLang 比較演算子による比較です。 このチュートリアルでは、GoLang で文字列を比較するこれら 2つの方法を示します。
GoLang で Compare()
メソッドを使用して文字列を比較する
GoLang の組み込みメソッド Compare()
は、2つの文字列を比較するために使用されます。 このメソッドは、辞書編集順序を使用して 2つの文字列を比較します。 このメソッドの構文は次のとおりです。
func Compare(a, b string) int
a
と b
は比較する 2つの文字列で、整数値を返します。 文字列の比較に基づく 3つの戻り値の型があります。
- a == b の場合、
compare()
メソッドは 0 を返します。 - a > b の場合、
compare()
メソッドは 1 を返します。 - a < b の場合、
compare()
メソッドは -1 を返します。
Compare()
メソッドを使用して文字列を比較する例を試してみましょう。
package main
import (
"fmt"
"strings"
)
func main() {
var string1 = "x"
var string2 = "y"
var string3 = "Delftstack"
var string4 = "Delftscope"
// string1 < string2 should return -1
fmt.Println(strings.Compare(string1, string2))
// string2 > string1 should return 1
fmt.Println(strings.Compare(string2, string1))
// string1 == string1 should return 0
fmt.Println(strings.Compare(string1, string1))
// string3 > string4 should return 1
fmt.Println(strings.Compare(string3, string4))
// string4 < string3 should return -1
fmt.Println(strings.Compare(string4, string3))
// Let's create a condition
string5 := "Hello this is delftstack.com!"
string6 := "Hello this is DELFTSTACK.COM!"
// using the Compare function
if strings.Compare(string5, string6) == 0 {
fmt.Println("The strings are a match.")
} else {
fmt.Println("The strings do not match.")
}
}
上記のコードは、Compare()
メソッドを使用して文字列を比較しようとし、上記の整数を返します。 このコードは、返された整数に基づいて条件も作成します。
出力を参照してください。
-1
1
0
1
-1
The strings do not match.
GoLang で比較演算子を使用して文字列を比較する
GoLang は、他の言語と同様に比較演算子もサポートしています。 これらの比較演算子には、==
、!=
、>=
、<=
、<
、>
が含まれます。 ==
と !=
は文字列の等価性をチェックするために使用され、他の 4つは辞書順で文字列を比較するために使用されます。
これらの比較演算子はブール演算子、つまり true
と false
を返します。 まず、==
および !=
演算子を使用して例を試してみましょう。
package main
import "fmt"
func main() {
string1 := "Delftsatck"
string2 := "Delft"
string3 := "Delftsatck.com"
string4 := "Delftsatck"
// using == operator
ComparisonResult1 := string1 == string2
ComparisonResult2 := string2 == string3
ComparisonResult3 := string3 == string4
ComparisonResult4 := string1 == string4
fmt.Println("Result 1 is: ", ComparisonResult1)
fmt.Println("Result 2 is: ", ComparisonResult2)
fmt.Println("Result 3 is: ", ComparisonResult3)
fmt.Println("Result 4 is: ", ComparisonResult4)
// using != operator
ComparisonResult5 := string1 != string2
ComparisonResult6 := string2 != string3
ComparisonResult7 := string3 != string4
ComparisonResult8 := string1 != string4
fmt.Println("\nResult 5 is: ", ComparisonResult5)
fmt.Println("Result 6 is: ", ComparisonResult6)
fmt.Println("Result 7 is: ", ComparisonResult7)
fmt.Println("Result 8 is: ", ComparisonResult8)
}
上記のコードは、指定された文字列を等しいかどうかに基づいて比較します。 出力を参照してください。
Result 1 is: false
Result 2 is: false
Result 3 is: false
Result 4 is: true
Result 5 is: true
Result 6 is: true
Result 7 is: true
Result 8 is: false
>=
、<=
、<
、>
演算子を使用して文字列を辞書順で比較する例を試してみましょう。 例を参照してください:
package main
import "fmt"
func main() {
string1 := "Delftsatck"
string2 := "Delft"
string3 := "Delftsatck.com"
string4 := "Delftsatck"
// using < operator
ComparisonResult1 := string1 < string2
ComparisonResult2 := string2 < string3
ComparisonResult3 := string3 < string4
ComparisonResult4 := string1 < string4
fmt.Println("Result 1 is: ", ComparisonResult1)
fmt.Println("Result 2 is: ", ComparisonResult2)
fmt.Println("Result 3 is: ", ComparisonResult3)
fmt.Println("Result 4 is: ", ComparisonResult4)
// using > operator
ComparisonResult5 := string1 > string2
ComparisonResult6 := string2 > string3
ComparisonResult7 := string3 > string4
ComparisonResult8 := string1 > string4
fmt.Println("\nResult 5 is: ", ComparisonResult5)
fmt.Println("Result 6 is: ", ComparisonResult6)
fmt.Println("Result 7 is: ", ComparisonResult7)
fmt.Println("Result 8 is: ", ComparisonResult8)
// using >= operator
ComparisonResult9 := string1 >= string2
ComparisonResult10 := string2 >= string3
ComparisonResult11 := string3 >= string4
ComparisonResult12 := string1 >= string4
fmt.Println("\nResult 9 is: ", ComparisonResult9)
fmt.Println("Result 10 is: ", ComparisonResult10)
fmt.Println("Result 11 is: ", ComparisonResult11)
fmt.Println("Result 12 is: ", ComparisonResult12)
// using <= operator
ComparisonResult13 := string1 <= string2
ComparisonResult14 := string2 <= string3
ComparisonResult15 := string3 <= string4
ComparisonResult16 := string1 <= string4
fmt.Println("\nResult 13 is: ", ComparisonResult13)
fmt.Println("Result 14 is: ", ComparisonResult14)
fmt.Println("Result 15 is: ", ComparisonResult15)
fmt.Println("Result 16 is: ", ComparisonResult16)
}
上記の文字列は、辞書編集順序に基づいて比較されます。 出力を参照してください。
Result 1 is: false
Result 2 is: true
Result 3 is: false
Result 4 is: false
Result 5 is: true
Result 6 is: false
Result 7 is: true
Result 8 is: false
Result 9 is: true
Result 10 is: false
Result 11 is: true
Result 12 is: true
Result 13 is: false
Result 14 is: true
Result 15 is: false
Result 16 is: true
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook