C# でキーで辞書の値を取得する
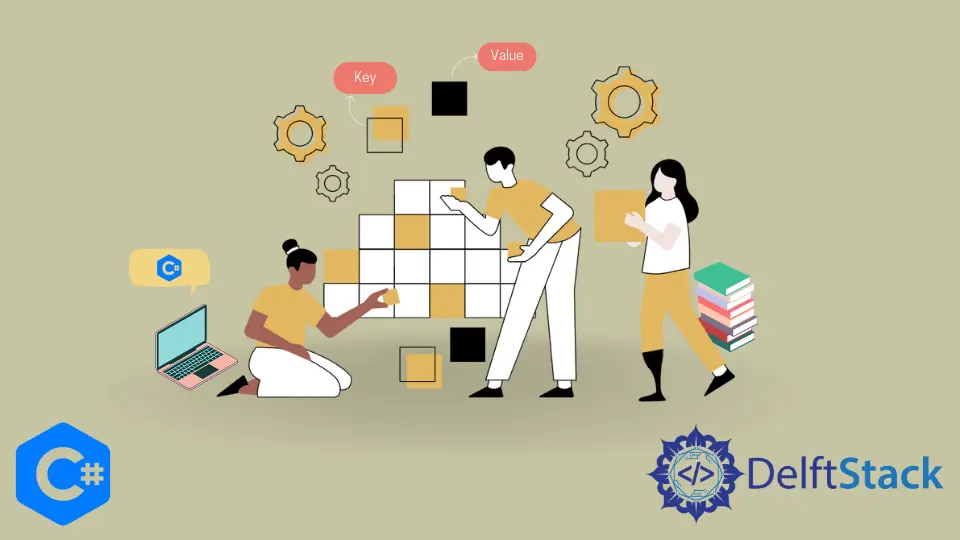
このチュートリアルでは、C# のキーで辞書の値を取得する方法について説明します。
C# の []
メソッドを使用してキーで辞書値を取得する
Dictionary<T1,T2>
クラスを使用して、C# で辞書を宣言できます。辞書は、キーと値
のペアの形式でデータを保持するデータ構造です。C# の []
メソッドでキーを使用すると、辞書の値を取得できます。
using System;
using System.Collections.Generic;
namespace get_dictionary_value {
class Program {
static void Main(string[] args) {
Dictionary<string, string> mydictionary = new Dictionary<string, string>();
mydictionary.Add("Key 1", "Value 1");
mydictionary.Add("Key 2", "Value 2");
mydictionary.Add("Key 3", "Value 3");
Console.WriteLine(mydictionary["Key 3"]);
}
}
}
出力:
Value 3
Dictionary<string, string>
クラスを使用して、辞書 mydictionary
を作成しました。その後、[]
メソッドを使用して、mydictionary
の Key 3
キーの値を取得しました。このメソッドの唯一の欠点は、キーが辞書に見つからない場合に例外が発生することです。
C# の TryGetKey()
関数を使用してキーごとに辞書値を取得する
TryGetKey()
関数は、キーは辞書に存在するか、C# に存在しません。TryGetKey()
関数はブール値を返します。キーがディクショナリに存在する場合、関数は true
を返し、out
パラメータの値をディクショナリ内のキーの値に変更します。キーが辞書に存在しない場合、関数は false
を返します。TryGetKey()
関数は、キーが辞書に存在しない場合に []
メソッドで発生した例外を処理します。次のコード例は、C# の TryGetkey()
関数を使用してキーで辞書の値を取得する方法を示しています。
using System;
using System.Collections.Generic;
namespace get_dictionary_value {
class Program {
static void Main(string[] args) {
Dictionary<string, string> mydictionary = new Dictionary<string, string>();
mydictionary.Add("Key 1", "Value 1");
mydictionary.Add("Key 2", "Value 2");
mydictionary.Add("Key 3", "Value 3");
string value;
bool hasValue = mydictionary.TryGetValue("Key 3", out value);
if (hasValue) {
Console.WriteLine(value);
} else {
Console.WriteLine("Key not present");
}
}
}
}
出力:
Value 3
まず、キーが mydictionary
辞書に存在するかどうかを確認します。その場合、値を取得して出力します。そうでない場合は、キーが存在しません
と出力します。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn