C#에서 키로 사전 값 가져 오기
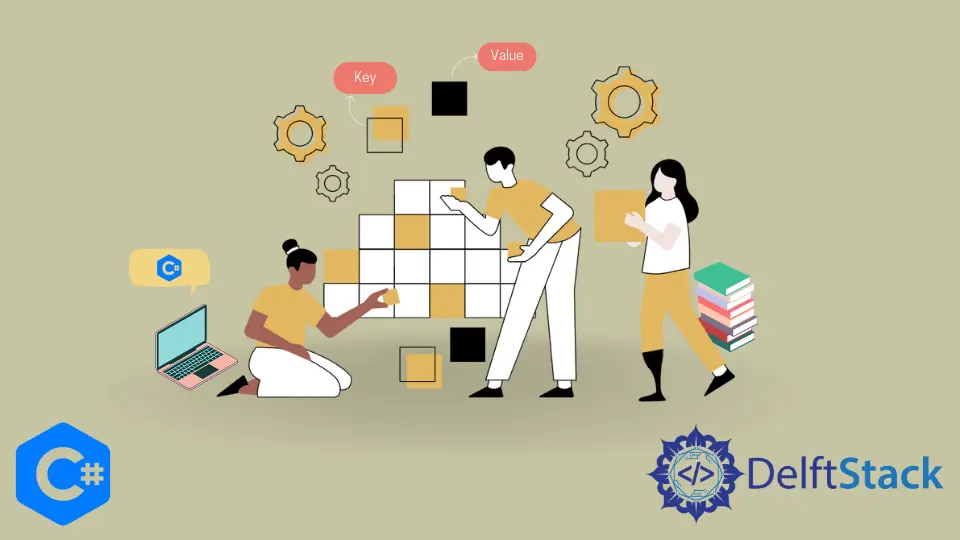
이 자습서에서는 C#의 키로 사전 값을 가져 오는 방법에 대해 설명합니다.
C#에서[]
메서드를 사용하여 키로 사전 값 가져 오기
Dictionary<T1,T2>
클래스를 사용할 수 있습니다. C#에서 사전을 선언합니다. 사전은 C#에서키-값
쌍의 형태로 데이터를 보유하는 데이터 구조입니다. C#에서[]
메소드와 함께 키를 사용하여 사전에있는 값을 가져올 수 있습니다.
using System;
using System.Collections.Generic;
namespace get_dictionary_value {
class Program {
static void Main(string[] args) {
Dictionary<string, string> mydictionary = new Dictionary<string, string>();
mydictionary.Add("Key 1", "Value 1");
mydictionary.Add("Key 2", "Value 2");
mydictionary.Add("Key 3", "Value 3");
Console.WriteLine(mydictionary["Key 3"]);
}
}
}
출력:
Value 3
Dictionary<string, string>
클래스를 사용하여mydictionary
사전을 만들었습니다. 그 후[]
메소드를 사용하여mydictionary
에서Key 3
키의 값을 검색했습니다. 이 방법의 유일한 결점은 키가 사전에서 발견되지 않으면 예외가 발생한다는 것입니다.
C#에서TryGetKey()
함수를 사용하여 키로 사전 값 가져 오기
TryGetKey()
함수는 키가 사전에 있거나 C#에 없습니다. TryGetKey()
함수는 부울 값을 반환합니다. 키가 사전에 있으면 함수는true
를 반환하고out
매개 변수의 값을 사전의 키 값으로 변경합니다. 키가 사전에 없으면 함수는false
를 반환합니다. TryGetKey()
함수는 키가 사전에없는 경우[]
메소드에서 발생한 예외를 처리합니다. 다음 코드 예제는 C#의TryGetkey()
함수를 사용하여 키로 사전의 값을 가져 오는 방법을 보여줍니다.
using System;
using System.Collections.Generic;
namespace get_dictionary_value {
class Program {
static void Main(string[] args) {
Dictionary<string, string> mydictionary = new Dictionary<string, string>();
mydictionary.Add("Key 1", "Value 1");
mydictionary.Add("Key 2", "Value 2");
mydictionary.Add("Key 3", "Value 3");
string value;
bool hasValue = mydictionary.TryGetValue("Key 3", out value);
if (hasValue) {
Console.WriteLine(value);
} else {
Console.WriteLine("Key not present");
}
}
}
}
출력:
Value 3
먼저 키가mydictionary
사전에 있는지 여부를 확인합니다. 그렇다면 값을 검색하여 인쇄합니다. 그렇지 않은 경우키 없음
을 인쇄합니다.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn