C# での URL エンコーディング
-
C#
で単純なASCII
を使用して文字をエンコードする -
C#
のさまざまな URL エンコード オプション -
C#
のSystem.Web
にあるその他のエンコード関数 -
C#
でUri.HexEscape()
を使用して文字列内の単一文字を変更する -
C#
で Temboo を使用して URL をエンコードする - まとめ
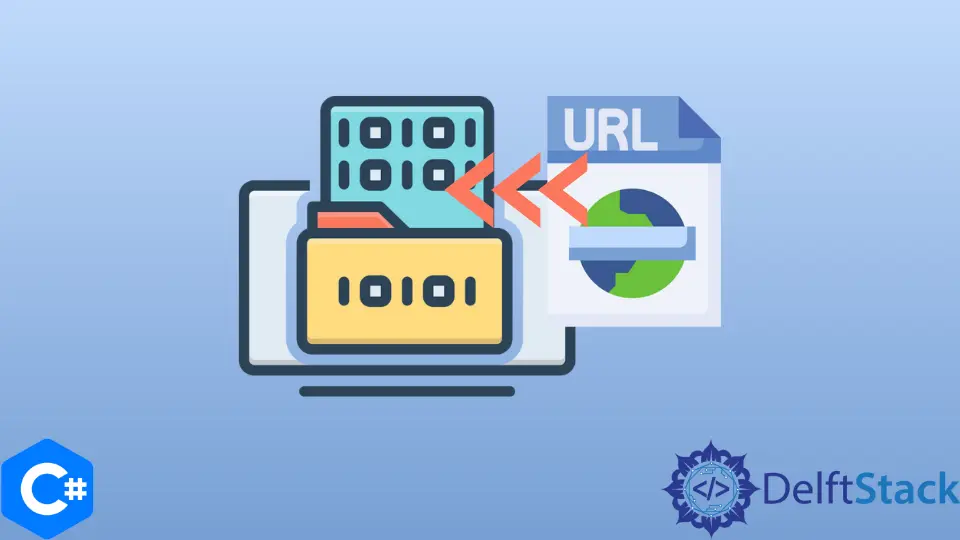
この記事では、C# で URL をエンコードする方法について説明します。
C#
で単純な ASCII
を使用して文字をエンコードする
キーボードの各文字の DECIMAL
値を表します。 したがって、この DECIMAL
値を文字列内の文字の 1つに置き換えて、効率的にエンコードすることができます。
using System;
class Program {
static void Main(string[] args) {
string s = "DelftStack";
string ascii_convert = "";
foreach (char c in s) {
ascii_convert += System.Convert.ToInt32(c);
}
Console.WriteLine("The converted ascii string is: " + ascii_convert);
}
}
System.Convert.ToInt32()
は、文字列内の文字の DECIMAL
表現を表す傾向があることに注意してください。 文字が変換されると、その値が新しい文字列 ASCII_CONVERT
に追加されます。これは、エンコードされた文字列を表します。
DECIMAL
を使用する代わりに、エンコードのニーズに合わせて BINARY
、OCTAL
、または HEXADECIMAL
変換を選択することもできます。
C#
のさまざまな URL エンコード オプション
C# では、さまざまなエンコーディング関数を使用できます。 多くの場合、URL
、URI
、HEX
など、文字をさまざまな表記法に変換します。
簡単な URL をこれらのさまざまな表記法に変換する関数を作成してみましょう。
まず、System.Web
名前空間をコード ファイルに追加します。 次に、関数を呼び出して文字列を URL 形式にエンコードし、文字列内の /, ., "
を ASCII (16 進数)
表現に変換します。
using System;
using System.Web;
class Program {
static void Main(string[] args) {
Console.WriteLine(HttpUtility.UrlEncode("https://www.google.com"));
}
}
出力:
https%3a%2f%2fwww.google.com
多くの場合、このような表現は、他のシステムによる認識で問題を引き起こします。 それほど重要でない情報のみをエンコードすることをお勧めします。
上記の例では、常に Google にアクセスできるようにしたいと考えています。 ただし、別の形式で表現すると、それが無効になり、認識できなくなる場合があります。
したがって、URL に属しているがユーザー固有の用語でエンコードを呼び出す必要があります。 たとえば、Google で "World"
を検索するとします。
URL は次のようになります。
https://www.google.com/search?q=World
ここでのq
はクエリを表します。 次のコードを使用してエンコードします。
コード例:
using System;
using System.Web;
class Program {
static void Main(string[] args) {
Console.WriteLine("https://www.google.com/search" + HttpUtility.UrlEncode("?q=World"));
}
}
出力:
https://www.google.com/search%3fq%3dWorld
C#
の System.Web
にあるその他のエンコード関数
コード例:
using System;
using System.Web;
class Program {
static void Main(string[] args) {
Console.WriteLine("URL ENCODE UNICODE: " +
HttpUtility.UrlEncodeUnicode("https://www.google.com/search?q=World"));
Console.WriteLine("URL PATH ENCODE: " +
HttpUtility.UrlPathEncode("https://www.google.com/search?q=World"));
Console.WriteLine("URI ESCAPE DATASTRING: " +
Uri.EscapeDataString("https://www.google.com/search?q=World"));
Console.WriteLine("URL ESCAPE URI STRING: " +
Uri.EscapeUriString("https://www.google.com/search?q=World"));
Console.WriteLine("HTML ENCODE: " +
HttpUtility.HtmlEncode("https://www.google.com/search?q=World"));
Console.WriteLine("HTML ATTRIBUTE ENCODE: " +
HttpUtility.HtmlAttributeEncode("https://www.google.com/search?q=World"));
}
}
出力:
URL ENCODE UNICODE: https%3a%2f%2fwww.google.com%2fsearch%3fq%3dWorld
URL PATH ENCODE: https://www.google.com/search?q=World
URI ESCAPE DATASTRING: https%3A%2F%2Fwww.google.com%2Fsearch%3Fq%3DWorld
URL ESCAPE URI STRING: https://www.google.com/search?q=World
HTML ENCODE: https://www.google.com/search?q=World
HTML ATTRIBUTE ENCODE: https://www.google.com/search?q=World
HttpUtility.UrlPathEncode()
、Uri.EscapeUriString()
、HttpUtility.HtmlEncode()
および HttpUtility.HtmlAttributeEncode()
は、エンコードされていない文字列と同じ結果を示す傾向があることに注意してください。
C#
で Uri.HexEscape()
を使用して文字列内の単一文字を変更する
C# で URL 文字列の文字をランダムに変更し、エンコードされた文字列を返す関数を作成してみましょう。
static string encoded_url(string param) {
Random rd = new Random();
int rand_num = rd.Next(0, param.Length - 1);
param = param.Remove(rand_num, 1);
param = param.Insert(rand_num, Uri.HexEscape(param[rand_num]));
return param;
}
次のようにメインで呼び出します。
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 1: " +
encoded_url("https://www.google.com/search?q=World"));
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 2: " +
encoded_url("https://www.google.com/search?q=World"));
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 3: " +
encoded_url("https://www.google.com/search?q=World"));
完全なソース コード:
using System;
using System.Web;
class Program {
static string encoded_url(string param) {
Random rd = new Random();
int rand_num = rd.Next(0, param.Length - 1);
param = param.Remove(rand_num, 1); /// returning the
param = param.Insert(rand_num, Uri.HexEscape(param[rand_num]));
return param;
}
static void Main(string[] args) {
// string s = Console.ReadLine();
// string ascii_convert = "";
// foreach (char c in s)
//{
// ascii_convert += System.Convert.ToInt32(c);
// }
// Console.WriteLine("The converted ascii string is: " + ascii_convert);
// Console.ReadKey();
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 1: " +
encoded_url("https://www.google.com/search?q=World"));
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 2: " +
encoded_url("https://www.google.com/search?q=World"));
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 3: " +
encoded_url("https://www.google.com/search?q=World"));
}
}
出力:
URI HEX ESCAPE for 'GOOGLE', called at 1: https://www.%6Foogle.com/search?q=World
URI HEX ESCAPE for 'GOOGLE', called at 2: https://www.go%67gle.com/search?q=World
URI HEX ESCAPE for 'GOOGLE', called at 3: https://www.google%63com/search?q=World
文字がランダムな位置で HEX
表現に変換される様子をご覧ください。 この URI.HexEscape()
関数をループで使用して、文字列全体または目的の文字を変更することもできます。
URLENCODE
はブラウザの互換性と使用に最適です。 また、URI
と HTTPUTILITY
の使用を明確にするために、URI
は SYSTEM
クラスから継承され、クライアント C# コード内に既に存在することに注意してください。
SYSTEM.WEB
を使用していない場合は、URI
を使用してからエンコーディング関数を呼び出したほうがよいでしょう。
ただし、SYSTEM.WEB
を名前空間として使用しても、多くの場合問題にはなりません。 プロジェクトが .NET FRAMEWORK CLIENT PROFILE
でコンパイルされている場合、コード ファイルを新しいプロジェクトにコピーする以外にできることはあまりありません。
.NET FRAMEWORK CLIENT PROFILE
は SYSTEM.WEB
。 SYSTEM.WEB
に名前を付けるときは、単純な .NET FRAMEWORK 4
を使用する必要があります。
C#
で Temboo を使用して URL をエンコードする
TEMBOO と呼ばれる API は、URL を完全にエンコードする傾向があります。
コード例:
// the NAMESPACES TO USE BEFORE UTILIZING THEIR CODE
using Temboo.Core;
using Temboo.Library.Utilities.Encoding;
TembooSession session = new TembooSession("ACCOUNT_NAME", "APP_NAME", "APP_KEY");
URLEncode urlEncodeChoreo = new URLEncode(session);
urlEncodeChoreo.setText("https://www.google.com/search?q=");
URLEncodeResultSet urlEncodeResults = urlEncodeChoreo.execute();
Console.WriteLine(urlEncodeResults.URLEncodedText);
まとめ
エンコード中に、変換される不正な文字があることを確認してください。 そうでない場合は、最初のソリューションに切り替えて、SWITCH
ケースを使用してエンコードする部分とエンコードしない部分を決定する混合関数を作成します。
最終的に、エンコーディングはユーザー次第です。 不正な文字と ASCII を切り替える機能には、反対側に同様のデコーダが必要です。
したがって、URL/文字列用のグローバル エンコーダー/デコーダーを備えたシステムで使用するのが最適です。
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub