C#의 URL 인코딩
-
단순
ASCII
를 사용하여C#
의 문자 인코딩 -
C#
의 다양한 URL 인코딩 옵션 -
C#
의System.Web
에 있는 다른 인코딩 기능 -
Uri.HexEscape()
를 사용하여C#
에서 문자열의 단일 문자 수정 -
C#
에서 Temboo를 사용하여 URL 인코딩 - 결론
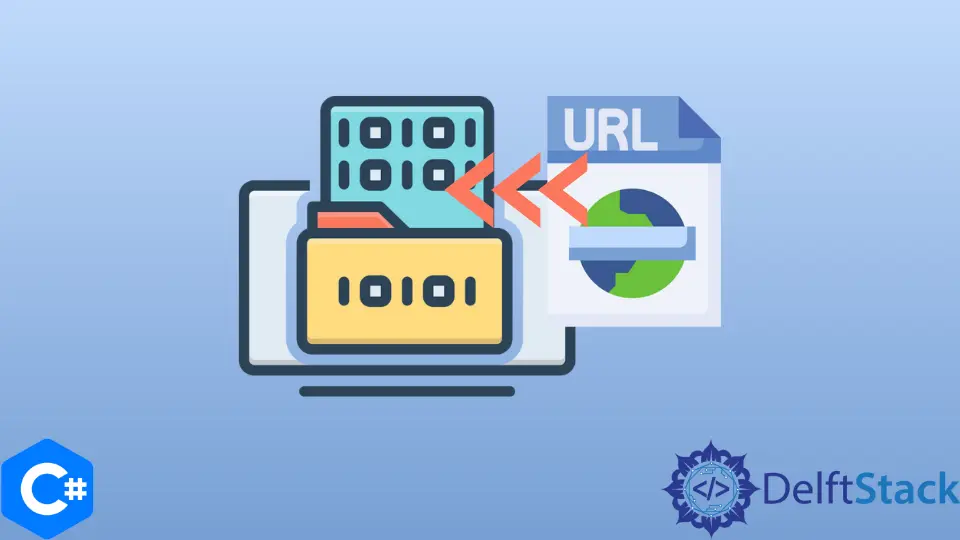
이 문서에서는 C#에서 URL을 인코딩하는 방법에 대해 설명합니다.
단순 ASCII
를 사용하여 C#
의 문자 인코딩
키보드에서 각 문자의 DECIMAL
값을 나타냅니다. 따라서 이 DECIMAL
값을 문자열의 문자 중 하나로 대체한 다음 효율적으로 인코딩할 수 있습니다.
using System;
class Program {
static void Main(string[] args) {
string s = "DelftStack";
string ascii_convert = "";
foreach (char c in s) {
ascii_convert += System.Convert.ToInt32(c);
}
Console.WriteLine("The converted ascii string is: " + ascii_convert);
}
}
System.Convert.ToInt32()
는 문자열에서 문자의 DECIMAL
표현을 나타내는 경향이 있습니다. 문자가 변환되면 해당 값이 이제 인코딩된 문자열을 나타내는 새 문자열 ASCII_CONVERT
에 추가됩니다.
DECIMAL
을 사용하는 대신 인코딩 요구 사항에 따라 BINARY
, OCTAL
또는 HEXADECIMAL
변환을 선택할 수도 있습니다.
C#
의 다양한 URL 인코딩 옵션
C#에는 다양한 인코딩 함수를 사용할 수 있습니다. 많은 사람들이 URL
, URI
, HEX
등과 같은 다른 표기법으로 문자를 변환합니다.
앞으로 나아가 간단한 URL을 이러한 다양한 표기법으로 변환하는 함수를 작성해 보겠습니다.
먼저 System.Web
네임스페이스를 코드 파일에 추가합니다. 그런 다음 함수를 호출하여 문자열을 URL 형식으로 인코딩하여 문자열의 /, ., "
를 ASCII(16진수)
표현으로 변환합니다.
using System;
using System.Web;
class Program {
static void Main(string[] args) {
Console.WriteLine(HttpUtility.UrlEncode("https://www.google.com"));
}
}
출력:
https%3a%2f%2fwww.google.com
이러한 표현은 다른 시스템에서 인식하는 데 문제를 일으키는 경우가 많습니다. 그다지 중요하지 않은 정보만 인코딩하도록 하는 것이 좋습니다.
위의 예에서 우리는 항상 Google에 액세스할 수 있기를 원합니다. 그러나 다른 형식으로 표현하면 무효화되고 인식할 수 없게 될 수 있습니다.
따라서 URL에 속하지만 사용자별로 다른 용어로 인코딩을 호출해야 합니다. 이제 Google에서 "세계"
를 검색한다고 가정해 보겠습니다.
URL은 다음과 같습니다.
https://www.google.com/search?q=World
여기서 q
는 쿼리를 나타냅니다. 다음 코드를 사용하여 인코딩하십시오.
예제 코드:
using System;
using System.Web;
class Program {
static void Main(string[] args) {
Console.WriteLine("https://www.google.com/search" + HttpUtility.UrlEncode("?q=World"));
}
}
출력:
https://www.google.com/search%3fq%3dWorld
C#
의 System.Web
에 있는 다른 인코딩 기능
예제 코드:
using System;
using System.Web;
class Program {
static void Main(string[] args) {
Console.WriteLine("URL ENCODE UNICODE: " +
HttpUtility.UrlEncodeUnicode("https://www.google.com/search?q=World"));
Console.WriteLine("URL PATH ENCODE: " +
HttpUtility.UrlPathEncode("https://www.google.com/search?q=World"));
Console.WriteLine("URI ESCAPE DATASTRING: " +
Uri.EscapeDataString("https://www.google.com/search?q=World"));
Console.WriteLine("URL ESCAPE URI STRING: " +
Uri.EscapeUriString("https://www.google.com/search?q=World"));
Console.WriteLine("HTML ENCODE: " +
HttpUtility.HtmlEncode("https://www.google.com/search?q=World"));
Console.WriteLine("HTML ATTRIBUTE ENCODE: " +
HttpUtility.HtmlAttributeEncode("https://www.google.com/search?q=World"));
}
}
출력:
URL ENCODE UNICODE: https%3a%2f%2fwww.google.com%2fsearch%3fq%3dWorld
URL PATH ENCODE: https://www.google.com/search?q=World
URI ESCAPE DATASTRING: https%3A%2F%2Fwww.google.com%2Fsearch%3Fq%3DWorld
URL ESCAPE URI STRING: https://www.google.com/search?q=World
HTML ENCODE: https://www.google.com/search?q=World
HTML ATTRIBUTE ENCODE: https://www.google.com/search?q=World
HttpUtility.UrlPathEncode()
, Uri.EscapeUriString()
, HttpUtility.HtmlEncode()
및 HttpUtility.HtmlAttributeEncode()
는 인코딩되지 않은 문자열과 동일한 결과를 나타내는 경향이 있습니다.
Uri.HexEscape()
를 사용하여 C#
에서 문자열의 단일 문자 수정
C#에서 URL 문자열의 문자를 임의로 수정한 다음 인코딩된 문자열을 반환하는 함수를 만들어 보겠습니다.
static string encoded_url(string param) {
Random rd = new Random();
int rand_num = rd.Next(0, param.Length - 1);
param = param.Remove(rand_num, 1);
param = param.Insert(rand_num, Uri.HexEscape(param[rand_num]));
return param;
}
다음과 같이 메인에서 호출합니다.
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 1: " +
encoded_url("https://www.google.com/search?q=World"));
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 2: " +
encoded_url("https://www.google.com/search?q=World"));
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 3: " +
encoded_url("https://www.google.com/search?q=World"));
완전한 소스 코드:
using System;
using System.Web;
class Program {
static string encoded_url(string param) {
Random rd = new Random();
int rand_num = rd.Next(0, param.Length - 1);
param = param.Remove(rand_num, 1); /// returning the
param = param.Insert(rand_num, Uri.HexEscape(param[rand_num]));
return param;
}
static void Main(string[] args) {
// string s = Console.ReadLine();
// string ascii_convert = "";
// foreach (char c in s)
//{
// ascii_convert += System.Convert.ToInt32(c);
// }
// Console.WriteLine("The converted ascii string is: " + ascii_convert);
// Console.ReadKey();
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 1: " +
encoded_url("https://www.google.com/search?q=World"));
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 2: " +
encoded_url("https://www.google.com/search?q=World"));
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 3: " +
encoded_url("https://www.google.com/search?q=World"));
}
}
출력:
URI HEX ESCAPE for 'GOOGLE', called at 1: https://www.%6Foogle.com/search?q=World
URI HEX ESCAPE for 'GOOGLE', called at 2: https://www.go%67gle.com/search?q=World
URI HEX ESCAPE for 'GOOGLE', called at 3: https://www.google%63com/search?q=World
임의의 위치에서 문자가 어떻게 HEX
표현으로 변환되는지 확인하십시오. 루프에서 이 URI.HexEscape()
함수를 사용하여 전체 문자열 또는 원하는 문자를 수정할 수도 있습니다.
URLENCODE
는 브라우저 호환성 및 사용에 가장 적합합니다. 또한 URI
및 HTTPUTILITY
의 사용을 명확히 하기 위해 URI
는 이미 클라이언트 C# 코드에 있는 SYSTEM
클래스에서 상속된다는 점을 기억하십시오.
SYSTEM.WEB
을 사용하지 않는 경우 URI
를 사용한 다음 인코딩 기능을 호출하는 것이 좋습니다.
그러나 SYSTEM.WEB
을 네임스페이스로 사용하는 것은 많은 경우에 문제가 되지 않습니다. 프로젝트가 .NET FRAMEWORK CLIENT PROFILE
에서 컴파일된 경우 코드 파일을 새 프로젝트에 복사하는 것 외에는 할 수 있는 일이 많지 않습니다.
.NET FRAMEWORK CLIENT PROFILE
은 SYSTEM.WEB
. SYSTEM.WEB
의 네이밍은 Simple .NET FRAMEWORK 4
를 활용해야 합니다.
C#
에서 Temboo를 사용하여 URL 인코딩
TEMBOO라는 API는 URL을 완벽하게 인코딩하는 경향이 있습니다.
예제 코드:
// the NAMESPACES TO USE BEFORE UTILIZING THEIR CODE
using Temboo.Core;
using Temboo.Library.Utilities.Encoding;
TembooSession session = new TembooSession("ACCOUNT_NAME", "APP_NAME", "APP_KEY");
URLEncode urlEncodeChoreo = new URLEncode(session);
urlEncodeChoreo.setText("https://www.google.com/search?q=");
URLEncodeResultSet urlEncodeResults = urlEncodeChoreo.execute();
Console.WriteLine(urlEncodeResults.URLEncodedText);
결론
인코딩하는 동안 변환될 불법 문자가 있는지 확인하십시오. 그렇지 않은 경우 첫 번째 솔루션으로 전환하고 SWITCH
사례를 사용하여 인코딩할 부분과 인코딩하지 않을 부분을 결정하는 혼합 함수를 만듭니다.
결국 인코딩은 사용자의 몫입니다. 잘못된 문자와 ASCII를 전환할 수 있는 함수는 반대쪽에 유사한 디코더가 필요합니다.
따라서 URL/문자열에 대한 전역 인코더/디코더가 있는 시스템에서 사용하는 것이 가장 좋습니다.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub