C# でデスクトップとアクティブ ウィンドウのスクリーンショットをキャプチャする
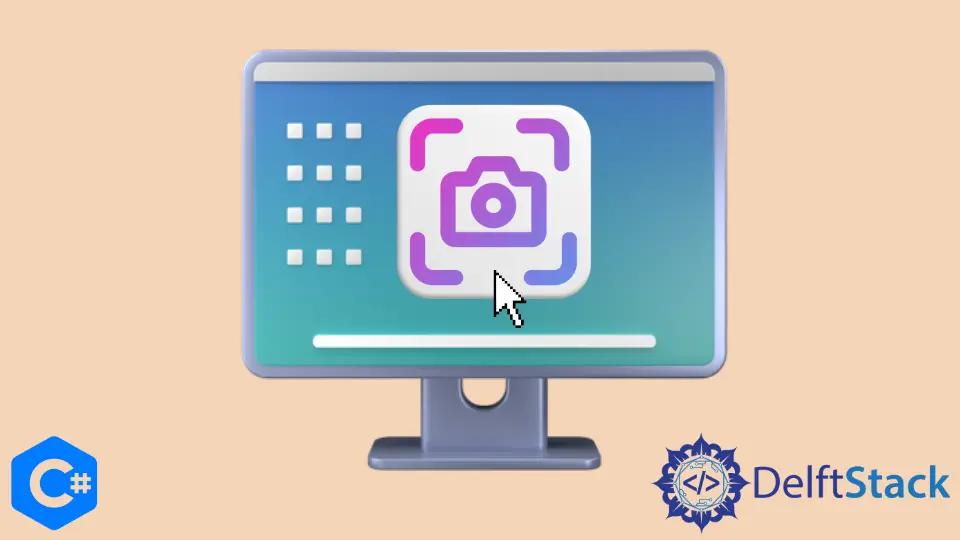
.Net
フレームワークは、スクリプト言語を使用せずに数行のコードでスクリーンショットをキャプチャできます。 これにより、完全な C# コードが開発者にとって理解しやすくなり、変更が容易になります。
このチュートリアルでは、C# でディスプレイまたはアクティブ ウィンドウの単一の画像をキャプチャする方法について説明します。
Windows 10 では、Windows.Graphics.Capture
は、スクリーンショットをキャプチャするためのさまざまな API を提供する強力な名前空間です。 デスクトップおよびアクティブ ウィンドウのスクリーンショットは、開発者にエンド ユーザー向けの安全なシステム ユーザー インターフェイスを作成するよう促します。
C#
でデスクトップのスクリーンショットをキャプチャする
Visual Studio で C# アプリケーションを作成し、Screenshot
という名前を付けます。 その後、ツールボックスに移動し、button1
コントロールを Form1.cs [Design]
に追加すると、次のコードが Form1.cs
で実行可能になります。
// add the following directories
using System;
using System.Drawing;
using System.Drawing.Imaging;
namespace Screenshot {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
// create a clieck event for the `Screenshot` button
private void button1_Click(object sender, EventArgs e) {
Screenshot();
}
private void Screenshot() {
try {
// set the screenshot resolution based on your monitor's resolution
Bitmap captureBitmap = new Bitmap(1024, 768, PixelFormat.Format32bppArgb);
Rectangle captureRectangle = Screen.AllScreens[0].Bounds;
Graphics captureGraphics = Graphics.FromImage(captureBitmap);
captureGraphics.CopyFromScreen(captureRectangle.Left, captureRectangle.Top, 0, 0,
captureRectangle.Size);
// select the save location of the captured screenshot
captureBitmap.Save(@"E:\Capture.jpg", ImageFormat.Jpeg);
// show a message to let the user know that a screenshot has been captured
MessageBox.Show("Screenshot taken! Press `OK` to continue...");
}
catch (Exception ex) {
MessageBox.Show("Error! " + ex.Message);
}
}
}
}
Windows フォーム アプリケーションを実装する最初の手順は、スクリーン キャプチャ機能を C# プロジェクトに追加することです。 Solution Explorer
で Package.appxmaanifest
を開き、Capabilities
タブを選択して Graphics Capture
を確認します。
システム UI を起動する前に、C# アプリケーションがスクリーンショットを取得できるかどうかを確認することが重要です。 デバイスがハードウェア要件を満たしていないか、システムがアプリケーションへのアクセスを許可していないことが、C# アプリケーションがスクリーンショットを撮れない理由として考えられます。
GraphicsCaptureSession
クラスの IsSupported
メソッドを使用して、C# アプリケーションがデスクトップまたはアクティブ ウィンドウのスクリーンショットをキャプチャできるかどうかを判断します。
public void OnInitialization() {
// check if the C# application supports screen capture
if (!GraphicsCaptureSession.IsSupported()) {
// this will hide the visibility of the `Screenshot` button if your C# application does not
// support the screenshot feature
button1.Visibility = Visibility.Collapsed;
}
}
C# の Graphics
オブジェクトから CopyFromScreen()
メソッドを使用して、開いているすべてのウィンドウを含むデスクトップのスクリーンショットをキャプチャすることができます。
using System;
using System.Drawing;
using System.Drawing.Imaging;
namespace Screenshot {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
private void button1_Click(object sender, EventArgs e) {
CaptureMyScreen();
}
private void CaptureMyScreen() {
Rectangle rect = Screen.GetBounds(Point.Empty);
using (Bitmap bitmap = new Bitmap(rect.Width, rect.Height)) {
using (Graphics g = Graphics.FromImage(bitmap)) {
g.CopyFromScreen(Point.Empty, Point.Empty, rect.Size);
}
// select the save location of the captured screenshot
bitmap.Save(@"E://Capture.png", ImageFormat.Png);
// show a message to let the user know that a screenshot has been captured
MessageBox.Show("Screenshot taken! Press `OK` to continue...");
}
}
}
}
C#
でアクティブ ウィンドウのスクリーンショットをキャプチャする
複数のアプリケーションを実行しているために多くのウィンドウが表示される場合は、アクティブなウィンドウのみをキャプチャすることが重要です。 アクティブなウィンドウは、現在キーボード フォーカスを保持しているウィンドウです。
C# の Graphics
オブジェクトの CopyFromScreen()
メソッドは、開いているすべてのウィンドウをキャプチャするデスクトップのスクリーンショットをキャプチャできますが、this.Bounds
を使用すると仕事が完了します。
using System;
using System.Drawing;
using System.Drawing.Imaging;
namespace Screenshot {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
private void button1_Click(object sender, EventArgs e) {
CaptureMyScreen();
}
private void CaptureMyScreen() {
Rectangle rect = this.Bounds;
using (Bitmap bitmap = new Bitmap(rect.Width, rect.Height)) {
using (Graphics g = Graphics.FromImage(bitmap)) {
g.CopyFromScreen(new Point(rect.Left, rect.Top), Point.Empty, rect.Size);
}
// select the save location of the captured screenshot
bitmap.Save(@"E://Capture.png", ImageFormat.Png);
// show a message to let the user know that a screenshot has been captured
MessageBox.Show("Screenshot taken! Press `OK` to continue...");
}
}
}
}
このチュートリアルでは、C# で開いているすべてのアクティブなウィンドウであるデスクトップのスクリーンショットをキャプチャする 3つの方法について説明しました。 上記の C# コードは好みに合わせて簡単にカスタマイズできます。
ただし、これはスクリーンショットをキャプチャするための最も最適化された C# コードです。
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub