C#에서 데스크톱 및 활성 창 스크린샷 캡처
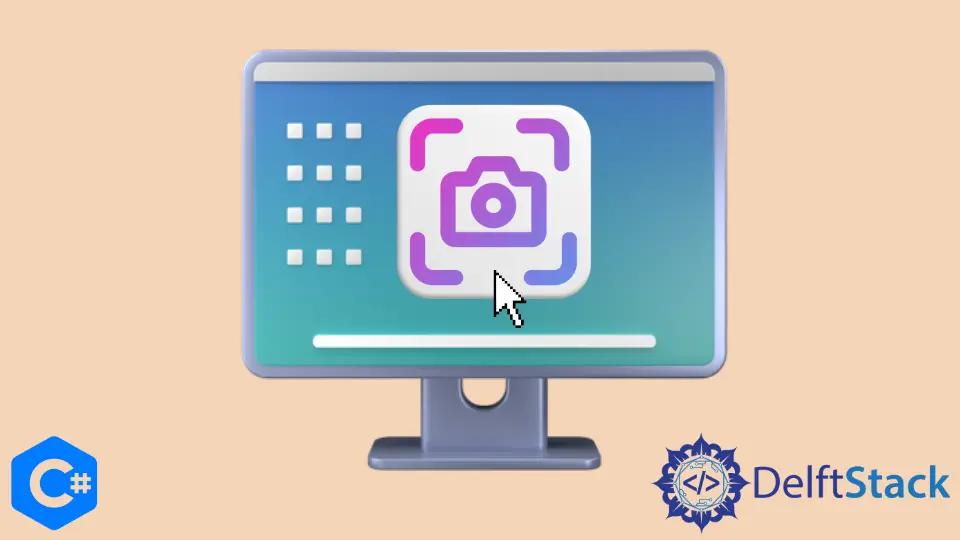
.Net
프레임워크는 스크립팅 언어를 사용하지 않고 몇 줄의 코드로 스크린샷을 캡처할 수 있습니다. 개발자가 전체 C# 코드를 이해하고 쉽게 수정할 수 있습니다.
이 자습서에서는 C#에서 디스플레이 또는 활성 창의 단일 이미지를 캡처하는 방법을 설명합니다.
Windows 10에서 Windows.Graphics.Capture
는 스크린샷 캡처를 위한 다양한 API를 제공하는 강력한 네임스페이스입니다. 데스크톱 및 활성 창 스크린샷은 최종 사용자를 위한 안전한 시스템 사용자 인터페이스를 만들기 위해 개발자를 호출합니다.
C#
에서 데스크톱 스크린샷 캡처
Visual Studio에서 C# 애플리케이션을 만들고 이름을 스크린샷
으로 지정합니다. 그런 다음 도구 상자로 이동하여 Form1.cs [Design]
에 button1
컨트롤을 추가하면 Form1.cs
에서 다음 코드를 실행할 수 있습니다.
// add the following directories
using System;
using System.Drawing;
using System.Drawing.Imaging;
namespace Screenshot {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
// create a clieck event for the `Screenshot` button
private void button1_Click(object sender, EventArgs e) {
Screenshot();
}
private void Screenshot() {
try {
// set the screenshot resolution based on your monitor's resolution
Bitmap captureBitmap = new Bitmap(1024, 768, PixelFormat.Format32bppArgb);
Rectangle captureRectangle = Screen.AllScreens[0].Bounds;
Graphics captureGraphics = Graphics.FromImage(captureBitmap);
captureGraphics.CopyFromScreen(captureRectangle.Left, captureRectangle.Top, 0, 0,
captureRectangle.Size);
// select the save location of the captured screenshot
captureBitmap.Save(@"E:\Capture.jpg", ImageFormat.Jpeg);
// show a message to let the user know that a screenshot has been captured
MessageBox.Show("Screenshot taken! Press `OK` to continue...");
}
catch (Exception ex) {
MessageBox.Show("Error! " + ex.Message);
}
}
}
}
Windows Form 애플리케이션을 구현하는 첫 번째 단계는 화면 캡처 기능을 C# 프로젝트에 추가하는 것입니다. Solution Explorer
에서 Package.appxmaanifest
를 열고 Capabilities
탭을 선택하여 Graphics Capture
를 확인합니다.
시스템 UI를 시작하기 전에 C# 애플리케이션이 스크린샷을 찍을 수 있는지 확인하는 것이 중요합니다. 하드웨어 요구 사항을 충족하지 않는 장치 또는 응용 프로그램에 대한 액세스 권한을 부여하지 않는 시스템은 C# 응용 프로그램이 스크린샷을 찍지 못하는 이유일 수 있습니다.
GraphicsCaptureSession
클래스의 IsSupported
메서드를 사용하여 C# 애플리케이션이 데스크톱 또는 활성 창 스크린샷을 캡처할 수 있는지 확인합니다.
public void OnInitialization() {
// check if the C# application supports screen capture
if (!GraphicsCaptureSession.IsSupported()) {
// this will hide the visibility of the `Screenshot` button if your C# application does not
// support the screenshot feature
button1.Visibility = Visibility.Collapsed;
}
}
C#의 Graphics
개체에서 CopyFromScreen()
메서드를 사용하여 열려 있는 모든 창을 포함하는 바탕 화면의 스크린샷을 캡처할 수 있습니다.
using System;
using System.Drawing;
using System.Drawing.Imaging;
namespace Screenshot {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
private void button1_Click(object sender, EventArgs e) {
CaptureMyScreen();
}
private void CaptureMyScreen() {
Rectangle rect = Screen.GetBounds(Point.Empty);
using (Bitmap bitmap = new Bitmap(rect.Width, rect.Height)) {
using (Graphics g = Graphics.FromImage(bitmap)) {
g.CopyFromScreen(Point.Empty, Point.Empty, rect.Size);
}
// select the save location of the captured screenshot
bitmap.Save(@"E://Capture.png", ImageFormat.Png);
// show a message to let the user know that a screenshot has been captured
MessageBox.Show("Screenshot taken! Press `OK` to continue...");
}
}
}
}
C#
에서 활성 창의 스크린샷 캡처
실행 중인 여러 애플리케이션으로 인해 많은 창이 표시되는 경우 활성 창만 캡처하는 것이 중요합니다. 활성 창은 현재 키보드 포커스를 유지하는 창입니다.
C#에서 Graphics
개체의 CopyFromScreen()
메서드는 열려 있는 모든 창을 캡처하는 데스크톱의 스크린샷을 캡처할 수 있지만 this.Bounds
를 사용하면 작업이 완료됩니다.
using System;
using System.Drawing;
using System.Drawing.Imaging;
namespace Screenshot {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
private void button1_Click(object sender, EventArgs e) {
CaptureMyScreen();
}
private void CaptureMyScreen() {
Rectangle rect = this.Bounds;
using (Bitmap bitmap = new Bitmap(rect.Width, rect.Height)) {
using (Graphics g = Graphics.FromImage(bitmap)) {
g.CopyFromScreen(new Point(rect.Left, rect.Top), Point.Empty, rect.Size);
}
// select the save location of the captured screenshot
bitmap.Save(@"E://Capture.png", ImageFormat.Png);
// show a message to let the user know that a screenshot has been captured
MessageBox.Show("Screenshot taken! Press `OK` to continue...");
}
}
}
}
이 자습서에서는 데스크톱 스크린샷을 캡처하는 세 가지 방법(C#에서 열려 있고 활성화된 모든 창)에 대해 설명했습니다. 취향에 따라 위의 C# 코드를 쉽게 사용자 정의할 수 있습니다.
그러나 스크린샷 캡처에 가장 최적화된 C# 코드입니다.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub