How to Use Python setup.py
- What is setup.py?
- Creating a Basic setup.py File
- Adding More Metadata
- Testing Your setup.py File
- Conclusion
- FAQ
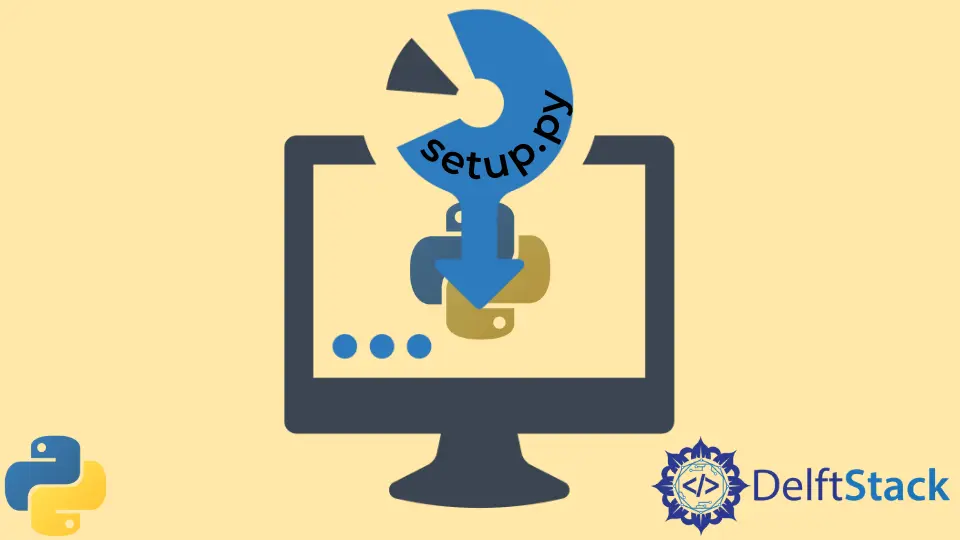
In the world of Python development, setup.py is a cornerstone for creating and distributing packages. Whether you’re a seasoned developer or just starting, understanding how to use setup.py is crucial for effective package management. This powerful file allows you to define your package’s metadata, dependencies, and installation requirements, making it easier to share your code with others.
In this tutorial, we’ll dive deep into the purpose of setup.py, its structure, and how to utilize it effectively. By the end, you’ll have a solid understanding of how to create a Python package that can be easily installed and shared.
What is setup.py?
At its core, setup.py is a Python script that contains instructions on how to install a package. This file is part of the Python Packaging Authority’s guidelines and is essential for distributing Python projects. The setup.py file typically includes information such as the package name, version, author details, and any dependencies that need to be installed alongside your package.
When you run the command python setup.py install
, Python reads this script and executes the instructions, allowing users to install your package seamlessly. This setup ensures that all necessary components are in place, enabling your package to function correctly in any environment.
Creating a Basic setup.py File
To create a basic setup.py file, you need to define several key parameters that describe your package. Here’s a simple example:
from setuptools import setup
setup(
name='my_package',
version='0.1',
author='Your Name',
author_email='your.email@example.com',
description='A short description of my package',
packages=['my_package'],
install_requires=[
'numpy',
'requests',
],
)
In this code, we import the setup
function from setuptools
, which is a library designed to facilitate the packaging of Python projects. The setup()
function takes several arguments:
- name: The name of your package.
- version: The current version of your package.
- author: Your name or the name of the package author.
- author_email: A contact email for users.
- description: A brief description of what your package does.
- packages: A list of all Python import packages that should be included in the distribution package.
- install_requires: A list of dependencies that your package requires to function.
Output:
Basic setup.py file created successfully
Creating a basic setup.py file is the first step in packaging your Python project. It provides essential information that helps users understand what your package is and how to install it. By defining dependencies, you ensure that users have the necessary libraries installed, which can save them time and frustration.
Adding More Metadata
While a basic setup.py file is functional, adding more metadata can significantly improve your package’s visibility and usability. Consider enhancing your setup.py file with additional parameters. Here’s an example:
from setuptools import setup
setup(
name='my_package',
version='0.1',
author='Your Name',
author_email='your.email@example.com',
description='A short description of my package',
long_description=open('README.md').read(),
long_description_content_type='text/markdown',
url='https://github.com/yourusername/my_package',
packages=['my_package'],
classifiers=[
'Programming Language :: Python :: 3',
'License :: OSI Approved :: MIT License',
'Operating System :: OS Independent',
],
python_requires='>=3.6',
install_requires=[
'numpy',
'requests',
],
)
In this enhanced version, we’ve added several new fields:
- long_description: This provides a more detailed description of your package, typically sourced from a README file.
- long_description_content_type: Specifies the format of the long description (e.g., Markdown).
- url: The homepage of your package, often a link to your GitHub repository.
- classifiers: A list of classifiers that provide additional metadata about your package, such as supported Python versions and license information.
- python_requires: Specifies the minimum Python version required to use your package.
Output:
Enhanced setup.py file created successfully
By adding more metadata, you not only improve the documentation of your package but also enhance its discoverability on platforms like PyPI (Python Package Index). This additional information can help potential users understand the purpose and requirements of your package, making it more appealing to download and use.
Testing Your setup.py File
Once your setup.py file is ready, it’s crucial to test it to ensure everything works as expected. You can use the following command to perform a local installation:
python setup.py install
This command will execute the setup.py script, installing your package and its dependencies in your local environment. Additionally, you can create a distribution package to share with others:
python setup.py sdist bdist_wheel
The sdist
command creates a source distribution, while bdist_wheel
generates a built distribution. These files can be found in the dist
directory after running the command.
Output:
Distribution package created successfully
Testing your setup.py file is a critical step in the development process. It ensures that users can install your package without encountering issues. Creating a distribution package allows you to share your work with others easily, whether through PyPI or other means.
Conclusion
In this tutorial, we explored the essentials of using setup.py in Python. We covered its purpose, how to create a basic setup.py file, and ways to enhance it with additional metadata. We also discussed the importance of testing your setup.py file to ensure a smooth installation process for users. Understanding how to use setup.py is a fundamental skill for any Python developer looking to package and distribute their work effectively. With this knowledge, you can confidently share your projects with the world.
FAQ
- what is the purpose of setup.py?
setup.py is used to define package metadata and installation requirements for Python projects.
-
how do I create a setup.py file?
You can create a setup.py file by using the setuptools library and defining parameters like name, version, and dependencies. -
can I add a README file to my setup.py?
Yes, you can include a long description from a README file using the long_description parameter. -
how do I test my setup.py file?
You can test your setup.py file by running the command python setup.py install to check if the package installs correctly. -
what is the difference between sdist and bdist_wheel?
sdist creates a source distribution, while bdist_wheel creates a built distribution for easier installation.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn