Comparators in Python
-
Use the
cmp
Argument With thesorted()
Function to Sort an Array in Python -
Use the
functools.cmp_to_key()
to Sort an Array in Python - Use the Custom Comparator Function to Sort an Array in Python
- Conclusion
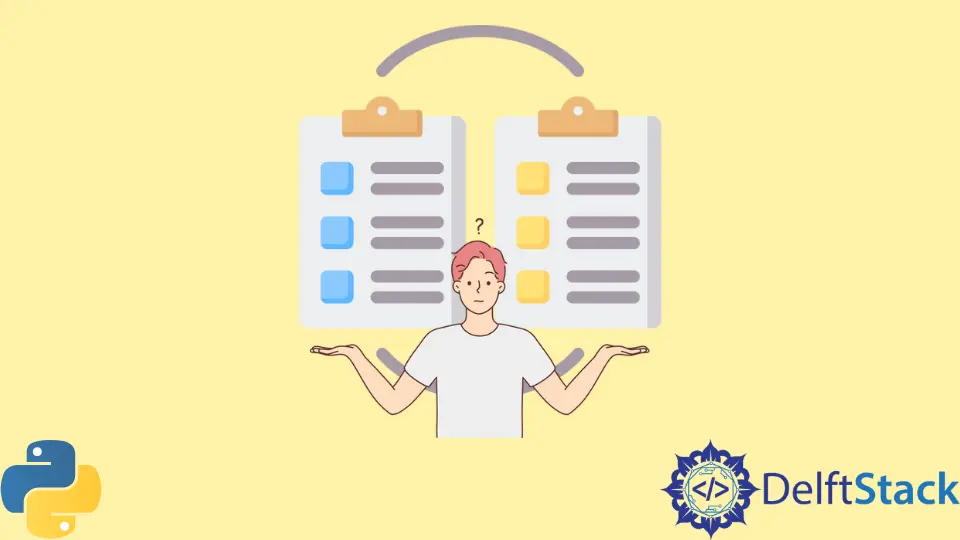
In this tutorial, we embark on a detailed exploration of comparators in Python, a critical tool for customizing the sorting of arrays. Comparators are essential in programming, as they allow for the comparison of two objects based on defined criteria.
This becomes particularly significant in sorting operations, where the default sorting behavior might not suffice. Our journey begins with the traditional use of the cmp
argument in Python 2, navigating through the evolution of sorting techniques to the more advanced and adaptable methods in Python 3, like the functools.cmp_to_key()
function and the use of custom comparator functions.
Use the cmp
Argument With the sorted()
Function to Sort an Array in Python
This method only works in Python 2 versions and is removed in the newer versions of Python released after the version Python 3.
Earlier, the sorted()
method had a cmp
argument that utilized comparators to sort a given data structure.
The following code uses the cmp
argument with the sorted()
function to sort an array in Python 2.
def compare_absolute(x, y):
return cmp(abs(x), abs(y))
numbers = [3, -1, -2, 4, 0, -3, 2]
sorted_numbers = sorted(numbers, cmp=compare_absolute)
print("Sorted Numbers:", sorted_numbers)
In our code, we first define the compare_absolute
function, which takes two parameters, x
and y
. Inside this function, we use the cmp
function to compare the absolute values of x
and y
.
The sorted
function then uses this comparator to sort the numbers
list. We pass the compare_absolute
function to sorted
using the cmp
parameter.
Finally, we print the sorted list, which is sorted based on the absolute values of its elements.
The above code provides the following output:
The output displays the list sorted by the absolute values of its elements. The use of cmp
in this context allows for a flexible comparison mechanism, tailoring the sorting process to our specific needs.
Use the functools.cmp_to_key()
to Sort an Array in Python
While we can use the cmp()
function provided by Python in Python 2, the function does not exist for the newer version in Python 3. The use of comparators
is restricted in the newer versions of Python.
A comparator
function is used to sort a given data structure along with the help of the sorted()
function. In Python 3, we use a key
function to carry out a custom sorting process.
Here, we use the functools.cmp_to_key()
function from the functools
library in order to convert the newly obsolete cmp
function to a key
function.
The following code uses a comparator
function to sort a given data structure.
import functools
def compare_length(x, y):
return len(x) - len(y)
strings = ["apple", "fig", "banana", "cherry"]
sorted_strings = sorted(strings, key=functools.cmp_to_key(compare_length))
print("Sorted Strings:", sorted_strings)
In this code snippet, we first import the functools
module. We then define a comparison function compare_length
that takes two strings, x
and y
, and returns the difference in their lengths.
This function serves as our custom comparator. In the sorted
function, we use functools.cmp_to_key(compare_length)
to convert our comparator into a key function.
This key function is then used to sort the list strings
. We conclude by printing the sorted list of strings.
The above code provides the following output:
The output demonstrates the strings sorted by their lengths, showcasing the effectiveness of using functools.cmp_to_key
for custom sorting operations. This approach is highly versatile, allowing Python programmers to define precise sorting criteria easily.
Use the Custom Comparator Function to Sort an Array in Python
In Python, sorting and comparison operations often require customization beyond the standard behavior. This is where custom comparator functions come into play.
They allow developers to define specific criteria for comparing elements, providing a high degree of control over the sorting process.
Let’s sort a list of strings based on their first character.
def custom_comparator(string):
return string[0]
strings = ["banana", "apple", "cherry"]
sorted_strings = sorted(strings, key=custom_comparator)
print("Sorted Strings:", sorted_strings)
In the above code, we define the custom_comparator
function that takes a string and returns its first character. We then use this function as the key for the sorted()
function.
When we sort the list strings
, the sorted()
function compares the strings based on the first character of each string. This method is straightforward and highly effective for custom sorting based on specific string characteristics.
The above code provides the following output:
The output displays the strings sorted by their first character. apple
appears first because its initial character a
comes alphabetically before b
(in banana
) and c
(in cherry
).
Conclusion
In conclusion, our exploration of array sorting in Python using comparators has unveiled the versatility and adaptability of Python in managing data. From the deprecated cmp
argument in Python 2 to the innovative use of functools.cmp_to_key()
and custom comparator functions in Python 3, we have seen how Python’s sorting capabilities have evolved to meet diverse programming needs.
These methods not only offer a deeper understanding of Python’s sorting mechanics but also empower developers with the flexibility to implement custom sorting criteria. This tutorial underscores Python’s commitment to providing robust tools for data manipulation, catering to both straightforward and complex sorting requirements.
Whether you are a beginner or an experienced developer, these sorting techniques in Python offer valuable insights and practical solutions for a wide range of programming challenges.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn