Usar variables globales en React Native
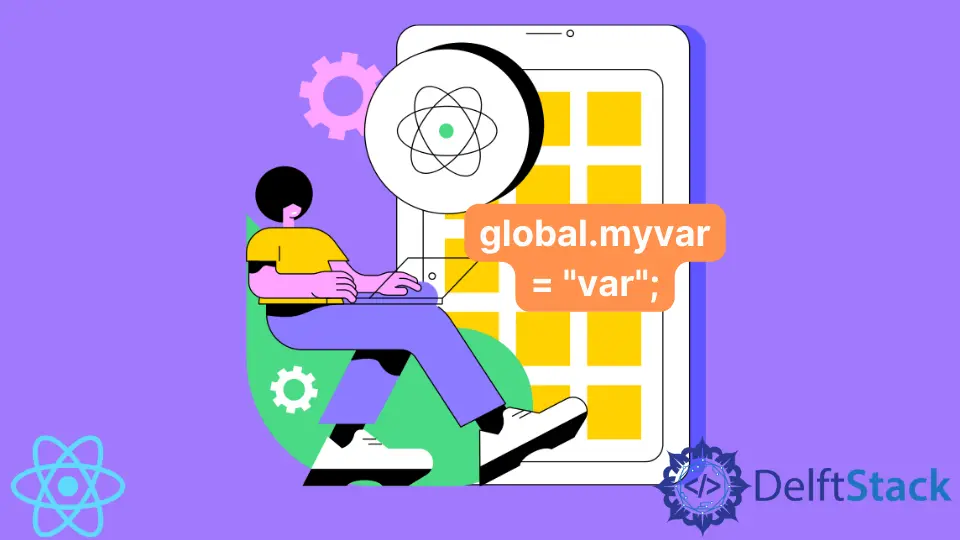
En este artículo, veremos las variables globales y cómo inicializarlas y usarlas en React Native.
React Native tiene dos variables diferentes por defecto: local y global. Si bien las variables globales se pueden usar en cualquier lugar, en cualquier actividad y se pueden cambiar desde cualquier lugar, las variables locales solo se utilizan dentro de un rango establecido.
Las variables de alcance global, que se pueden utilizar en muchas actividades, son compatibles con React Native. Por lo tanto, en este tutorial, crearemos variables de alcance global en React Native y las usaremos en muchas actividades.
Usar variables globales en React Native
Debemos utilizar el término integrado global
para construir variables globales. Para obtener más información, consulte el código a continuación.
Sintaxis:
global.myvar = 'Welcome to Upwork';
Si tenemos una variable local llamada myvar
, solo está disponible desde la misma clase. Y si tenemos global.myvar
, se vuelve global y accesible desde cualquier clase de aplicación.
El siguiente ejemplo le ayudará a comprenderlo. En la primera pantalla se inicializó una variable global.MyVar
, a la que se accede desde la primera y la segunda pantalla.
Primero, importe la biblioteca react-navigation
a su proyecto para crear una estructura de actividad, ya que no podemos utilizar muchas actividades en nuestro proyecto sin ella.
npm install --save react-navigation
Ahora, con cualquier editor de código, abra App.js
y reemplace el código con lo siguiente.
Código de ejemplo (App.js
):
import 'react-native-gesture-handler';
import {NavigationContainer} from '@react-navigation/native';
import {createStackNavigator} from '@react-navigation/stack';
import React from 'react';
import FirstPage from './pages/FirstPage';
import SecondPage from './pages/SecondPage';
const Stack = createStackNavigator();
function App() {
return (
<NavigationContainer>
<Stack.Navigator initialRouteName='FirstPage'>
<Stack.Screen
name = 'FirstPage'
component={FirstPage}
options={
{
title: 'First Page', headerStyle: {
backgroundColor: '#f4511e',
},
headerTintColor: '#fff', headerTitleStyle: {
fontWeight: 'bold',
},
}}
/>
<Stack.Screen
name="SecondPage"
component={SecondPage}
options={{
title: "Second Page",
headerStyle: {
backgroundColor: "#f4511e",
},
headerTintColor: "#fff",
headerTitleStyle: {
fontWeight: "bold",
},
}}
/>
</Stack.Navigator>
</NavigationContainer>
);
}
export default App;
Navigator
utilizado aquí le permite crear componentes y ayuda a navegar por diferentes componentes. Ayuda a navegar entre las pantallas, como si tuviéramos un elemento de la pantalla llamado “Primera página”, que tiene propiedades como título, color, estilo de encabezado, fuente, peso de fuente, etc.
Asimismo, la segunda pantalla se denomina Segunda página
, con propiedades como título, color, estilo de encabezado, fuente, grosor de fuente, etc.
Reemplace el código en FirstPage.js
con el siguiente código en cualquier editor de código.
import React from 'react';
import {Button, SafeAreaView, Text, View} from 'react-native';
const FirstPage = ({navigation}) => {
global.MyVar = 'Welcome To Upwork';
return (
<SafeAreaView style={{ flex: 1 }}>
<View style={{ flex: 1, padding: 16 }}>
<View
style={{
flex: 1,
alignItems: 'center',
justifyContent: 'center',
}}
>
<Text
style={{
fontSize: 25,
textAlign: 'center',
marginBottom: 16,
}}
>
Here is the First Page of the App
</Text>
<Text
style={{
fontSize: 18,
textAlign: "center",
marginBottom: 16,
color: "red",
}}
>
Value of Global Variable is: {global.MyVar}
</Text>
<Button
onPress={() => navigation.navigate('SecondPage')}
title='Go to Second Page.'
/>
</View>
</View>
</SafeAreaView>
);
};
export default FirstPage;
Una variable global se declara utilizando la palabra clave global
. Después de usar la palabra clave global
, tenemos que hacer que sus variables almacenen el valor global.
Como puedes ver, se declara globalmente la variable MyVar
, que almacena el valor Welcome To Upwork
. Solo tenemos que inicializarlo una vez.
Ahora podemos usar esa variable en diferentes páginas cuando sea necesario.
En cualquier editor de código, abra SecondPage.js
y reemplace el código con lo siguiente.
import React from 'react';
import {SafeAreaView, Text, View} from 'react-native';
const SecondPage = () => {
return (
<SafeAreaView style={{ flex: 1 }}>
<View style={{ flex: 1, padding: 16 }}>
<View
style={{
flex: 1,
alignItems: 'center',
justifyContent: 'center',
}}
>
<Text
style={{
fontSize: 25,
textAlign: 'center',
marginBottom: 16,
}}
>
Here is the Second Page of the App
</Text>
<Text
style={{
fontSize: 18,
textAlign: "center",
marginBottom: 16,
color: "red",
}}
>
Value of Global Variable is: {global.MyVar}
</Text>
</View>
</View>
</SafeAreaView>
);
};
export default SecondPage;
Producción:
En la captura de pantalla se puede ver el valor de la variable y el botón con el nombre IR A SEGUNDA PAGINA
. Representa la primera página en la segunda página con la ayuda de un navegador.
Aquí, en la captura de pantalla, puede ver que también se usa la variable global.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn