React Native에서 전역 변수 사용
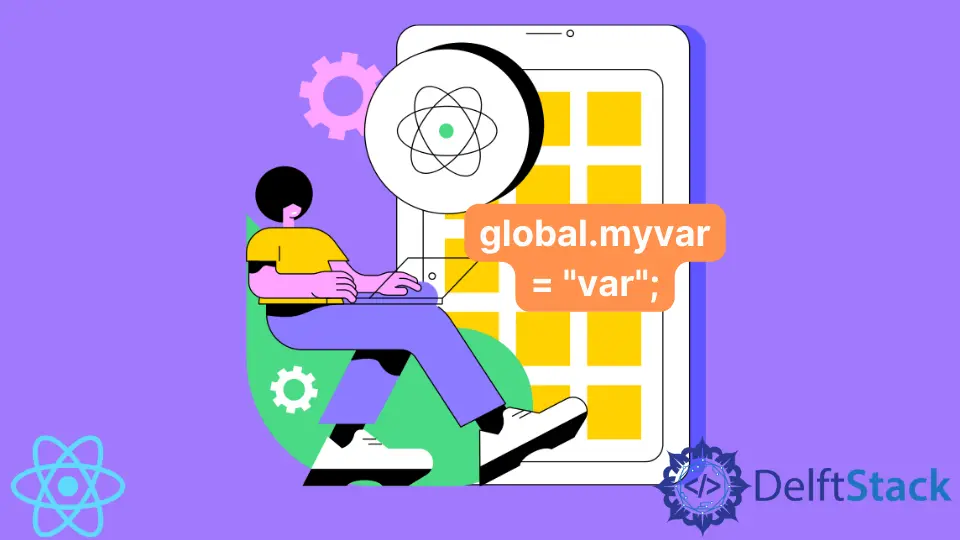
이번 글에서는 전역 변수에 대해 알아보고 초기화하는 방법과 React Native에서 사용하는 방법에 대해 알아보겠습니다.
React Native에는 기본적으로 로컬 및 글로벌이라는 두 가지 변수가 있습니다. 전역 변수는 어떤 활동에서나 어디서나 사용할 수 있고 어디에서나 변경할 수 있지만 지역 변수는 설정된 범위 내에서만 사용됩니다.
많은 활동에서 활용될 수 있는 전역 범위 변수는 React Native에서 지원됩니다. 따라서 이 튜토리얼에서는 React Native에서 전역 범위 변수를 생성하고 이를 여러 활동에서 사용합니다.
React Native에서 전역 변수 사용
전역 변수를 구성하려면 내장 용어 global
을 활용해야 합니다. 자세한 내용은 아래 코드를 참조하세요.
통사론:
global.myvar = 'Welcome to Upwork';
myvar
라는 로컬 변수가 있는 경우 동일한 클래스에서만 사용할 수 있습니다. 그리고 global.myvar
가 있으면 전역이 되고 모든 애플리케이션 클래스에서 액세스할 수 있습니다.
다음 예를 보면 이해하는 데 도움이 될 것입니다. 첫 번째 화면과 두 번째 화면에서 모두 액세스하는 global.MyVar
변수를 첫 번째 화면에서 초기화했습니다.
먼저 react-navigation
라이브러리를 프로젝트로 가져와 프로젝트에서 많은 활동을 활용할 수 없기 때문에 활동 구조를 만듭니다.
npm install --save react-navigation
이제 코드 편집기에서 App.js
를 열고 코드를 다음으로 바꿉니다.
예제 코드(App.js
):
import 'react-native-gesture-handler';
import {NavigationContainer} from '@react-navigation/native';
import {createStackNavigator} from '@react-navigation/stack';
import React from 'react';
import FirstPage from './pages/FirstPage';
import SecondPage from './pages/SecondPage';
const Stack = createStackNavigator();
function App() {
return (
<NavigationContainer>
<Stack.Navigator initialRouteName='FirstPage'>
<Stack.Screen
name = 'FirstPage'
component={FirstPage}
options={
{
title: 'First Page', headerStyle: {
backgroundColor: '#f4511e',
},
headerTintColor: '#fff', headerTitleStyle: {
fontWeight: 'bold',
},
}}
/>
<Stack.Screen
name="SecondPage"
component={SecondPage}
options={{
title: "Second Page",
headerStyle: {
backgroundColor: "#f4511e",
},
headerTintColor: "#fff",
headerTitleStyle: {
fontWeight: "bold",
},
}}
/>
</Stack.Navigator>
</NavigationContainer>
);
}
export default App;
여기서 사용되는 Navigator
는 컴포넌트를 생성하고 다양한 컴포넌트를 탐색할 수 있도록 도와줍니다. 제목, 색상, 헤더 스타일, 글꼴, 글꼴 두께 등과 같은 속성이 있는 ‘첫 페이지’라는 화면 요소가 있는 것처럼 화면 사이를 탐색하는 데 도움이 됩니다.
마찬가지로 두 번째 화면은 제목, 색상, 헤더 스타일, 글꼴, 글꼴 두께 등과 같은 속성을 가진 두 번째 페이지
라고 합니다.
코드 편집기에서 FirstPage.js
의 코드를 다음 코드로 바꿉니다.
import React from 'react';
import {Button, SafeAreaView, Text, View} from 'react-native';
const FirstPage = ({navigation}) => {
global.MyVar = 'Welcome To Upwork';
return (
<SafeAreaView style={{ flex: 1 }}>
<View style={{ flex: 1, padding: 16 }}>
<View
style={{
flex: 1,
alignItems: 'center',
justifyContent: 'center',
}}
>
<Text
style={{
fontSize: 25,
textAlign: 'center',
marginBottom: 16,
}}
>
Here is the First Page of the App
</Text>
<Text
style={{
fontSize: 18,
textAlign: "center",
marginBottom: 16,
color: "red",
}}
>
Value of Global Variable is: {global.MyVar}
</Text>
<Button
onPress={() => navigation.navigate('SecondPage')}
title='Go to Second Page.'
/>
</View>
</View>
</SafeAreaView>
);
};
export default FirstPage;
전역 변수는 global
키워드를 사용하여 선언됩니다. global
키워드를 사용한 후에는 해당 변수가 전역 값을 저장하도록 해야 합니다.
보시다시피 MyVar
변수는 Welcome To Upwork
값을 저장하는 전역적으로 선언됩니다. 한 번만 초기화하면 됩니다.
이제 필요할 때 다른 페이지에서 해당 변수를 사용할 수 있습니다.
코드 편집기에서 SecondPage.js
를 열고 코드를 다음으로 바꿉니다.
import React from 'react';
import {SafeAreaView, Text, View} from 'react-native';
const SecondPage = () => {
return (
<SafeAreaView style={{ flex: 1 }}>
<View style={{ flex: 1, padding: 16 }}>
<View
style={{
flex: 1,
alignItems: 'center',
justifyContent: 'center',
}}
>
<Text
style={{
fontSize: 25,
textAlign: 'center',
marginBottom: 16,
}}
>
Here is the Second Page of the App
</Text>
<Text
style={{
fontSize: 18,
textAlign: "center",
marginBottom: 16,
color: "red",
}}
>
Value of Global Variable is: {global.MyVar}
</Text>
</View>
</View>
</SafeAreaView>
);
};
export default SecondPage;
출력:
스크린샷에서 변수 값과 GO TO SECOND PAGE
라는 이름의 버튼을 볼 수 있습니다. 네비게이터의 도움으로 첫 번째 페이지를 두 번째 페이지로 렌더링합니다.
여기 스크린샷에서 전역 변수도 사용되는 것을 볼 수 있습니다.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn