How to Initialize a Map Containing Arrays in TypeScript
-
Initialize a Map Containing an Array Using the
Array
Class in TypeScript -
Initialize a Map Containing an Array Using Square Brackets
[]
in TypeScript
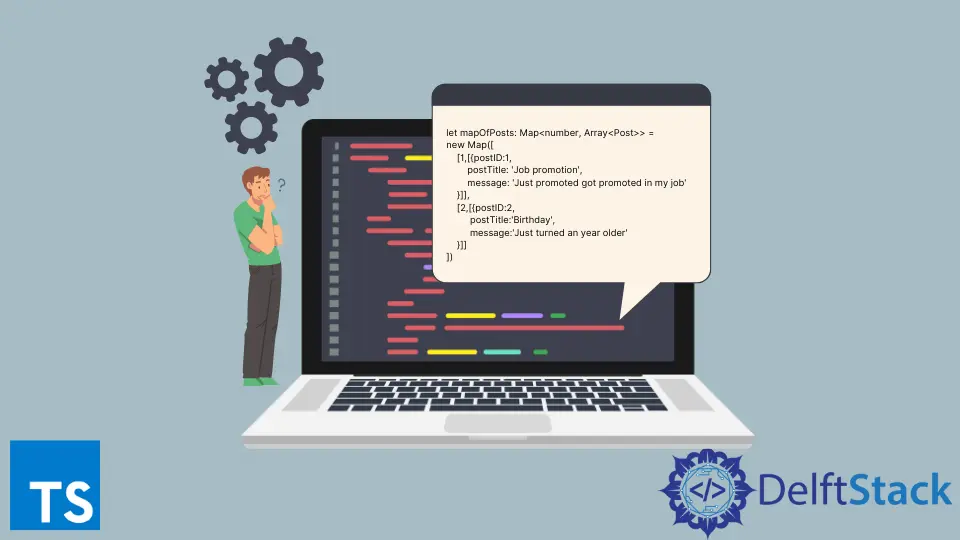
A Map is a data structure that keeps data in the form of the key-value pairs. You can use any data type you prefer for both the keys and the values.
In this tutorial, we will learn to create a Map that uses numbers as keys and an array of custom types as values.
Initialize a Map Containing an Array Using the Array
Class in TypeScript
Go to Visual Studio Code and create a folder named map-initialization
or use any name preferred. Create a file named array-class-map.ts
under the folder.
Copy and paste the following code into the file.
type Post = {
postID: number
postTitle: string
message: string
}
let mapOfPosts: Map<number, Array<Post>> =
new Map([
[1,[{postID:1,
postTitle: 'Job promotion',
message: 'Just promoted got promoted in my job'
}]],
[2,[{postID:2,
postTitle:'Birthday',
message:'Just turned an year older'
}]]
])
mapOfPosts.forEach((post) => console.log(post))
In the above code, we have defined our custom object named Post
that we will use in our Map as values.
Since Map is generic, the first parameter of the angle brackets indicates that the key is of type number
. The second parameter uses another type called Array<Post>
to indicate that the value is an array of posts.
The Array
is a generic class used to create an array of elements by specifying the type of elements in the angle brackets. In our case, we have passed Post
as the type of element in the angle brackets.
ForEach()
is a functional method that iterates through the array of elements in the Map behind the scenes and prints them to the console.
To execute the above example, go to the terminal and cd
to the location of this folder. Generate a tsconfig.json
file using the following command.
david@david-HP-ProBook-6470b:~/Documents/map-initialization$ tsc --init
Ensure the tsconfig.json
file has the following configuration.
{
"compilerOptions":{
"target": "es5"
"noEmitOnError": true,
}
}
The Map API was introduced in es5
, and thus we added the library to prevent any compilation errors in our code.
Use the following command to transpile the TypeScript code to a JavaScript code that can be executed using node
.
david@david-HP-ProBook-6470b:~/Documents/map-initialization$ tsc
Once the code has been compiled, a file named array-class-map.js
will be generated. Use the following command to execute the JavaScript file.
david@david-HP-ProBook-6470b:~/Documents/map-initialization$ node array-class-map.js
Output:
[
{
postID: 1,
postTitle: 'Job promotion',
message: 'Just promoted got promoted in my job'
}
]
[
{
postID: 2,
postTitle: 'Birthday',
message: 'Just turned an year older'
}
]
Initialize a Map Containing an Array Using Square Brackets []
in TypeScript
In this example, we will use the basic method known by most developers, even those with a basic understanding of arrays.
Create a file named maps.ts
under the same folder. Copy and paste the following code into the file.
type Post = {
postID: number
postTitle: string
message: string
}
let mapOfPosts: Map<number, Post[]> =
new Map([
[1,[{postID:1,
postTitle: 'Job promotion',
message: 'Just promoted got promoted in my job'
}]],
[2,[{postID:2,
postTitle:'Birthday',
message:'Just turned an year older'
}]]
])
mapOfPosts.forEach((post) => console.log(post))
In the above code, we have defined a Map that accepts keys of type number
and an array of Post
as values.
The basic way of creating an array is by defining the type followed by square brackets as indicated by Post[]
. The Map has been initialized on one line using the new
keyword, and two concrete elements have been added to the Map.
This example works the same way as the previous example, apart from the fact that it creates the array of posts for the Map values using square brackets.
To execute this example, use the same approach as the previous example to get the following output.
Output:
[
{
postID: 1,
postTitle: 'Job promotion',
message: 'Just promoted got promoted in my job'
}
]
[
{
postID: 2,
postTitle: 'Birthday',
message: 'Just turned an year older'
}
]
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHubRelated Article - TypeScript Map
- How to Enforce Types for Indexed Members in TypeScript Objects
- How to Declare Map or List Type in TypeScript
- How to Declare an ES6 Map in TypeScript