How to Get and Set in TypeScript
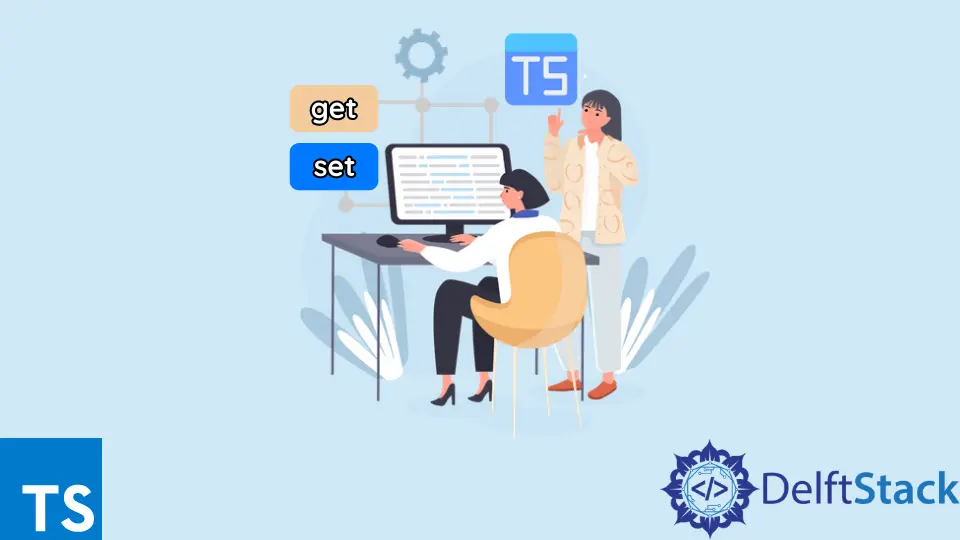
This tutorial will discuss the get and set properties for a class in TypeScript.
Get and Set in TypeScript
Get and set are the properties of the class that look like methods. In general terms, we consider properties as fields.
Get
property is used to get the variable. It will have a return statement to return something.
Get is used to access the variable.
Set
property is used to set the variable. It will set the variable, and it is up to the user in which way he wants to set the variable.
class ABC {
name: string;
myName() {
console.log('My name is ' + this.name);
}
}
let abc = new ABC();
abc.name = "John Nash";
abc.myName();
Output:
My name is John Nash
The above example shows that the field name
of type string
and the method myName()
are accessible outside the class. It is because, by default, everything inside the class is considered public.
Now the method myName()
can have a parameter to set the value of field name
. The below example clears this concept.
class ABC {
name: string;
myName(setName: string) {
this.name = setName;
}
}
let abc = new ABC();
abc.myName("Jack Hudge");
console.log('My name is ' + abc.name);
Output:
My name is Jack Hudge
The method myName()
sets the field name
, accessible everywhere. Now, let’s change the modifier for the field to private.
class ABC {
private name: string;
}
let abc = new ABC();
abc.name = "John Hudge";
Output:
Property 'name' is private and only accessible within class 'ABC'.
Notice the error occurred when the user tried to access the field name
outside the class. We will use the properties get
and set
to solve this problem and assign value to the field name
.
class ABC {
private Name: string;
get name() : string {
return this.Name;
}
set name(setName: string){
this.Name = setName;
}
}
let abc = new ABC();
abc.name = "Albert Thomas"; //set property
console.log('My name is ' + abc.name); //get property
Output:
My name is Albert Thomas
In the above example, the field Name
being private is now accessible using the properties get
and set
. The get
method returns the field Name
value, and the set method assigns value to the Name
field.
Now let’s take another example with more than one field.
class Demo {
private x: number;
private y: number;
constructor(X: number, Y: number) {
this.x = X;
this.y = Y;
}
myResult() {
console.log('The value of x = ' + this.x + ' and The value of y = ' + this.y);
}
}
let test = new Demo(4,9);
test.myResult();
Output:
The value of x = 4 and The value of y = 9
In the above code example, the constructor()
method is used to initialize the object of the class, and it can also set the value of the fields. Each time a new instance of a class is created, the constructor must have these two number parameters even if the parameters are of no use.
We can write the constructor as constructor(X ?: number, Y ?: number)
. Now the question mark ?
makes the parameters optional, and the user can now instantiate the class as let test1 = new Demo()
.
TypeScript allows the use of the constructor for access modifiers and reduces the code. The following code works the same as the snippet above but with less code and assigned statements.
class Demo {
constructor(private x?: number, private y?: number) {
}
myResult() {
console.log('The value of x = ' + this.x + ' and The value of y = ' + this.y);
}
}
let test = new Demo(3,7);
test.myResult();
Output:
The value of x = 3 and The value of y = 7
The above examples are only valid if the fields are called only once because they are private, so they can’t be accessed outside the class. Moreover, if the above code is kept the same way and reassigns the fields, the user must instantiate the class again.
The new instance looks like this let test1 = new Demo(4,3)
. We will use the get
and set
properties.
class Demo {
constructor(private x?: number, private y?: number) {
}
getX() {
return this.x;
}
setX(value) {
this.x = value;
}
getY() {
return this.y;
}
setY(value) {
this.y = value
}
myResult() {
console.log('The value of x = ' + this.x + ' and The value of y = ' + this.y);
}
}
let test = new Demo(3,7);
test.myResult();
test.setX(5);
console.log('The updated value of x = ' + test.getX());
test.setY(10);
console.log('The updated value of y = ' + test.getY());
test.myResult();
Output:
The value of x = 3 and The value of y = 7
The updated value of x = 5
The updated value of y = 10
The value of x = 5 and The value of y = 10
The methods getX()
and getY()
are used to return the updated values of the fields x
and y
respectively. The methods setX(value)
and setY(value)
are used to assign the new value to the fields x
and y
.
The methods are used in the following example instead of using the get
and set
properties. The get
and set
are reserved keywords used separately.
class Demo {
constructor(private _x?: number, private _y?: number) {
}
get x(): number {
return this._x;
}
set x(value: number) {
this._x = value;
}
get y(): number {
return this._y;
}
set y(value: number) {
this._y = value
}
myResult() {
console.log('The value of x = ' + this._x + ' and The value of y = ' + this._y);
}
}
let test = new Demo(1,2);
test.myResult();
test.x = 8; //setter for x
console.log('The updated value of x = ' + test.x); //getter for x
test.y = 12; //setter for y
console.log('The updated value of y = ' + test.y); //getter for y
test.myResult();
Output:
The value of x = 1 and The value of y = 2
The updated value of x = 8
The updated value of y = 12
The value of x = 8 and The value of y = 12
The properties are used as fields to assign and get the values of x
and y
. Since the field and property use, camel casing and have the same name, which raises an error as Duplicate Identifier
.
The fields are named _x
and _y
, and the properties are named without underscore. The set
for field x
is called a field assignment, and the get
for x
is used in the same way as for the field.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn