在 TypeScript 中獲取和設定
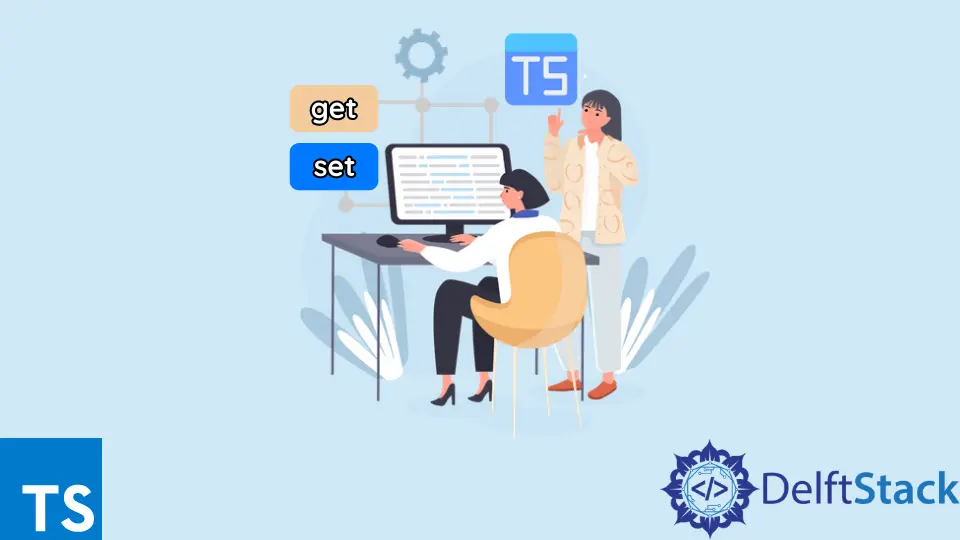
本教程將討論 TypeScript 中類的 get 和 set 屬性。
在 TypeScript 中獲取和設定
Get 和 set 是類的屬性,看起來像方法。一般而言,我們將屬性視為欄位。
Get
屬性用於獲取變數。它將有一個返回語句來返回一些東西。
Get 用於訪問變數。
Set
屬性用於設定變數。它將設定變數,這取決於使用者想要以何種方式設定變數。
class ABC {
name: string;
myName() {
console.log('My name is ' + this.name);
}
}
let abc = new ABC();
abc.name = "John Nash";
abc.myName();
輸出:
My name is John Nash
上面的例子表明,型別為 string
的欄位 name
和方法 myName()
可以在類外部訪問。這是因為,預設情況下,類中的所有內容都被認為是公開的。
現在方法 myName()
可以有一個引數來設定欄位 name
的值。下面的示例清除了這個概念。
class ABC {
name: string;
myName(setName: string) {
this.name = setName;
}
}
let abc = new ABC();
abc.myName("Jack Hudge");
console.log('My name is ' + abc.name);
輸出:
My name is Jack Hudge
myName()
方法設定欄位 name
,可在任何地方訪問。現在,讓我們將欄位的修飾符更改為私有。
class ABC {
private name: string;
}
let abc = new ABC();
abc.name = "John Hudge";
輸出:
Property 'name' is private and only accessible within class 'ABC'.
請注意,當使用者嘗試訪問類外的欄位 name
時發生錯誤。我們將使用屬性 get
和 set
來解決這個問題並將值分配給欄位 name
。
class ABC {
private Name: string;
get name() : string {
return this.Name;
}
set name(setName: string){
this.Name = setName;
}
}
let abc = new ABC();
abc.name = "Albert Thomas"; //set property
console.log('My name is ' + abc.name); //get property
輸出:
My name is Albert Thomas
在上面的例子中,欄位 Name
是私有的,現在可以使用屬性 get
和 set
訪問。get
方法返回欄位 Name
的值,set 方法將值分配給 Name
欄位。
現在讓我們再舉一個包含多個欄位的示例。
class Demo {
private x: number;
private y: number;
constructor(X: number, Y: number) {
this.x = X;
this.y = Y;
}
myResult() {
console.log('The value of x = ' + this.x + ' and The value of y = ' + this.y);
}
}
let test = new Demo(4,9);
test.myResult();
輸出:
The value of x = 4 and The value of y = 9
在上面的程式碼示例中,constructor()
方法用於初始化類的物件,它還可以設定欄位的值。每次建立一個類的新例項時,建構函式必須有這兩個數字引數,即使這些引數沒有用。
我們可以將建構函式寫為 constructor(X ?: number, Y ?: number)
。現在問號 ?
使引數可選,使用者現在可以將類例項化為 let test1 = new Demo()
。
TypeScript 允許將建構函式用於訪問修飾符並減少程式碼。以下程式碼與上面的程式碼片段相同,但程式碼和分配的語句更少。
class Demo {
constructor(private x?: number, private y?: number) {
}
myResult() {
console.log('The value of x = ' + this.x + ' and The value of y = ' + this.y);
}
}
let test = new Demo(3,7);
test.myResult();
輸出:
The value of x = 3 and The value of y = 7
上面的例子只有在欄位只被呼叫一次的情況下才有效,因為它們是私有的,所以它們不能在類外訪問。此外,如果上述程式碼保持相同的方式並重新分配欄位,則使用者必須再次例項化該類。
新例項看起來像這樣 let test1 = new Demo(4,3)
。我們將使用 get
和 set
屬性。
class Demo {
constructor(private x?: number, private y?: number) {
}
getX() {
return this.x;
}
setX(value) {
this.x = value;
}
getY() {
return this.y;
}
setY(value) {
this.y = value
}
myResult() {
console.log('The value of x = ' + this.x + ' and The value of y = ' + this.y);
}
}
let test = new Demo(3,7);
test.myResult();
test.setX(5);
console.log('The updated value of x = ' + test.getX());
test.setY(10);
console.log('The updated value of y = ' + test.getY());
test.myResult();
輸出:
The value of x = 3 and The value of y = 7
The updated value of x = 5
The updated value of y = 10
The value of x = 5 and The value of y = 10
getX()
和 getY()
方法分別用於返回欄位 x
和 y
的更新值。setX(value)
和 setY(value)
方法用於將新值分配給欄位 x
和 y
。
在以下示例中使用這些方法,而不是使用 get
和 set
屬性。get
和 set
是單獨使用的保留關鍵字。
class Demo {
constructor(private _x?: number, private _y?: number) {
}
get x(): number {
return this._x;
}
set x(value: number) {
this._x = value;
}
get y(): number {
return this._y;
}
set y(value: number) {
this._y = value
}
myResult() {
console.log('The value of x = ' + this._x + ' and The value of y = ' + this._y);
}
}
let test = new Demo(1,2);
test.myResult();
test.x = 8; //setter for x
console.log('The updated value of x = ' + test.x); //getter for x
test.y = 12; //setter for y
console.log('The updated value of y = ' + test.y); //getter for y
test.myResult();
輸出:
The value of x = 1 and The value of y = 2
The updated value of x = 8
The updated value of y = 12
The value of x = 8 and The value of y = 12
這些屬性用作分配和獲取 x
和 y
值的欄位。由於欄位和屬性使用,camel case 和具有相同的名稱,這會引發錯誤為 Duplicate Identifier
。
欄位命名為 _x
和 _y
,屬性命名時不帶下劃線。欄位 x
的 set
稱為欄位分配,x
的 get
的使用方式與欄位相同。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn