在 TypeScript 中获取和设置
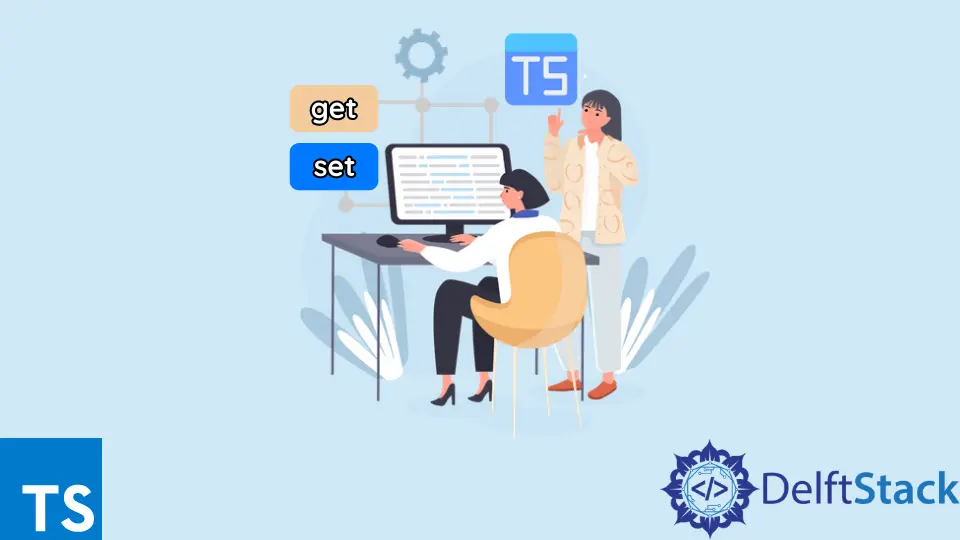
本教程将讨论 TypeScript 中类的 get 和 set 属性。
在 TypeScript 中获取和设置
Get 和 set 是类的属性,看起来像方法。一般而言,我们将属性视为字段。
Get
属性用于获取变量。它将有一个返回语句来返回一些东西。
Get 用于访问变量。
Set
属性用于设置变量。它将设置变量,这取决于用户想要以何种方式设置变量。
class ABC {
name: string;
myName() {
console.log('My name is ' + this.name);
}
}
let abc = new ABC();
abc.name = "John Nash";
abc.myName();
输出:
My name is John Nash
上面的例子表明,类型为 string
的字段 name
和方法 myName()
可以在类外部访问。这是因为,默认情况下,类中的所有内容都被认为是公开的。
现在方法 myName()
可以有一个参数来设置字段 name
的值。下面的示例清除了这个概念。
class ABC {
name: string;
myName(setName: string) {
this.name = setName;
}
}
let abc = new ABC();
abc.myName("Jack Hudge");
console.log('My name is ' + abc.name);
输出:
My name is Jack Hudge
myName()
方法设置字段 name
,可在任何地方访问。现在,让我们将字段的修饰符更改为私有。
class ABC {
private name: string;
}
let abc = new ABC();
abc.name = "John Hudge";
输出:
Property 'name' is private and only accessible within class 'ABC'.
请注意,当用户尝试访问类外的字段 name
时发生错误。我们将使用属性 get
和 set
来解决这个问题并将值分配给字段 name
。
class ABC {
private Name: string;
get name() : string {
return this.Name;
}
set name(setName: string){
this.Name = setName;
}
}
let abc = new ABC();
abc.name = "Albert Thomas"; //set property
console.log('My name is ' + abc.name); //get property
输出:
My name is Albert Thomas
在上面的例子中,字段 Name
是私有的,现在可以使用属性 get
和 set
访问。get
方法返回字段 Name
的值,set 方法将值分配给 Name
字段。
现在让我们再举一个包含多个字段的示例。
class Demo {
private x: number;
private y: number;
constructor(X: number, Y: number) {
this.x = X;
this.y = Y;
}
myResult() {
console.log('The value of x = ' + this.x + ' and The value of y = ' + this.y);
}
}
let test = new Demo(4,9);
test.myResult();
输出:
The value of x = 4 and The value of y = 9
在上面的代码示例中,constructor()
方法用于初始化类的对象,它还可以设置字段的值。每次创建一个类的新实例时,构造函数必须有这两个数字参数,即使这些参数没有用。
我们可以将构造函数写为 constructor(X ?: number, Y ?: number)
。现在问号 ?
使参数可选,用户现在可以将类实例化为 let test1 = new Demo()
。
TypeScript 允许将构造函数用于访问修饰符并减少代码。以下代码与上面的代码片段相同,但代码和分配的语句更少。
class Demo {
constructor(private x?: number, private y?: number) {
}
myResult() {
console.log('The value of x = ' + this.x + ' and The value of y = ' + this.y);
}
}
let test = new Demo(3,7);
test.myResult();
输出:
The value of x = 3 and The value of y = 7
上面的例子只有在字段只被调用一次的情况下才有效,因为它们是私有的,所以它们不能在类外访问。此外,如果上述代码保持相同的方式并重新分配字段,则用户必须再次实例化该类。
新实例看起来像这样 let test1 = new Demo(4,3)
。我们将使用 get
和 set
属性。
class Demo {
constructor(private x?: number, private y?: number) {
}
getX() {
return this.x;
}
setX(value) {
this.x = value;
}
getY() {
return this.y;
}
setY(value) {
this.y = value
}
myResult() {
console.log('The value of x = ' + this.x + ' and The value of y = ' + this.y);
}
}
let test = new Demo(3,7);
test.myResult();
test.setX(5);
console.log('The updated value of x = ' + test.getX());
test.setY(10);
console.log('The updated value of y = ' + test.getY());
test.myResult();
输出:
The value of x = 3 and The value of y = 7
The updated value of x = 5
The updated value of y = 10
The value of x = 5 and The value of y = 10
getX()
和 getY()
方法分别用于返回字段 x
和 y
的更新值。setX(value)
和 setY(value)
方法用于将新值分配给字段 x
和 y
。
在以下示例中使用这些方法,而不是使用 get
和 set
属性。get
和 set
是单独使用的保留关键字。
class Demo {
constructor(private _x?: number, private _y?: number) {
}
get x(): number {
return this._x;
}
set x(value: number) {
this._x = value;
}
get y(): number {
return this._y;
}
set y(value: number) {
this._y = value
}
myResult() {
console.log('The value of x = ' + this._x + ' and The value of y = ' + this._y);
}
}
let test = new Demo(1,2);
test.myResult();
test.x = 8; //setter for x
console.log('The updated value of x = ' + test.x); //getter for x
test.y = 12; //setter for y
console.log('The updated value of y = ' + test.y); //getter for y
test.myResult();
输出:
The value of x = 1 and The value of y = 2
The updated value of x = 8
The updated value of y = 12
The value of x = 8 and The value of y = 12
这些属性用作分配和获取 x
和 y
值的字段。由于字段和属性使用,camel case 和具有相同的名称,这会引发错误为 Duplicate Identifier
。
字段命名为 _x
和 _y
,属性命名时不带下划线。字段 x
的 set
称为字段分配,x
的 get
的使用方式与字段相同。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn