运算符重载
Jinku Hu
2020年6月25日
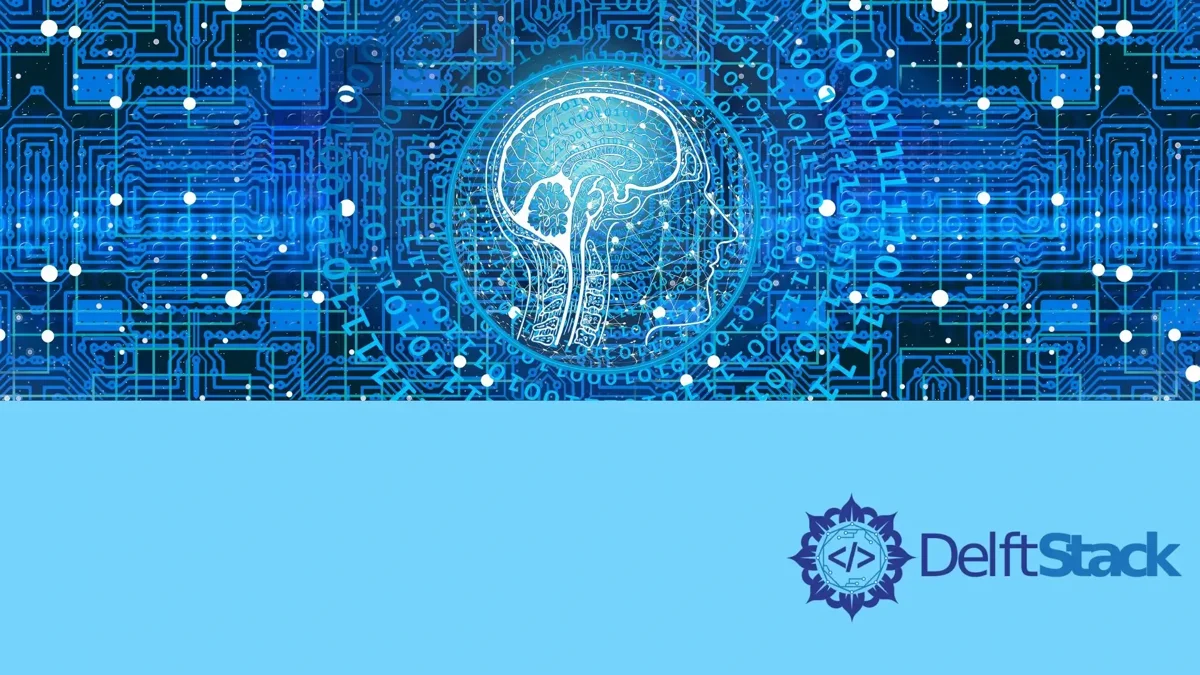
运算符重载
Python 中的一切都是对象,每个对象都有一些特殊的内部方法,用于与其他对象进行交互。通常,这些方法遵循 __action__
命名约定。
你可以重载这些方法中的任何一种。这通常用于 Python 中的运算符重载。下面是使用 Python 数据模型进行运算符重载的示例。在 Vector
类创建有两个变量的简单矢量。我们将使用运算符重载来支持两个向量的数学运算。
class Vector(object):
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, v):
# Addition with another vector.
return Vector(self.x + v.x, self.y + v.y)
def __sub__(self, v):
# Subtraction with another vector.
return Vector(self.x - v.x, self.y - v.y)
def __mul__(self, s):
# Multiplication with a scalar.
return Vector(self.x * s, self.y * s)
def __div__(self, s):
# Division with a scalar.
float_s = float(s)
return Vector(self.x / float_s, self.y / float_s)
def __floordiv__(self, s):
# Division with a scalar (value floored).
return Vector(self.x // s, self.y // s)
def __repr__(self):
# Print friendly representation of Vector class. Else, it would
# show up like, <__main__.Vector instance at 0x01DDDDC8>.
return "<Vector (%f, %f)>" % (
self.x,
self.y,
)
a = Vector(3, 5)
b = Vector(2, 7)
print a + b # Output: <Vector (5.000000, 12.000000)>
print b - a # Output: <Vector (-1.000000, 2.000000)>
print b * 1.3 # Output: <Vector (2.600000, 9.100000)>
print a // 17 # Output: <Vector (0.000000, 0.000000)>
print a / 17 # Output: <Vector (0.176471, 0.294118)>
通过运算符重载,我们可以让类进行一些数学运算,假如这些运算经常被调用到的话,这样就省去了通过函数来实现的步骤。
具体的可支持重载的运算符列表可以参见官方手册。