TypeScript 中的字符串格式化
Shuvayan Ghosh Dastidar
2023年1月30日
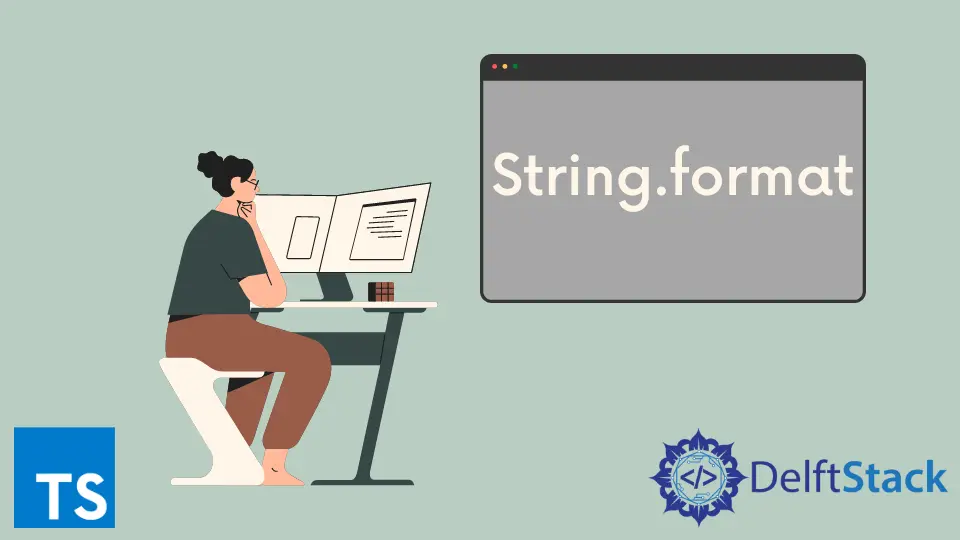
字符串是一组 16 位无符号整数值(UTF-16 代码单元)。TypeScript 中的字符串是不可变的,即特定索引处的值不能更改,但可以在字符串末尾附加字符串或字符值。
但是,由于字符串的只读属性,可以读取特定索引处的字符。
在 TypeScript 中附加字符串以形成新字符串
在 TypeScript 中,可以附加字符串以形成新的字符串。 +
或连接运算符连接两个字符串以形成最终字符串。
// string literal
var name : string = 'Geralt';
var nameString = 'The Witcher is of age 95 and his name is ' + name ;
console.log(nameString);
输出:
"The Witcher is of age 95, and his name is Geralt"
然而,这在大字符串的情况下变得不方便,因此在 TypeScript 中引入了模板
字符串。
在 TypeScript 中使用 Template
字符串形成字符串
Template
字符串是允许嵌入表达式的字符串文字。他们通过 ${``}
运算符使用字符串插值,因此可以嵌入任何表达式。
var name : string = "Geralt";
var age : number = 95;
var formedString = `The Witcher is of age ${age} and his name is ${name}`;
console.log(formedString);
输出:
"The Witcher is of age 95, and his name is Geralt"
请注意,在字符串的情况下,而不是一般的 ""
或 ''
。因此,模板字符串允许以一种更简洁的方式来表示字符串。
此外,使用模板字符串,动态处理变得非常方便。
var name : string = "Geralt";
var age : number = 95;
var enemiesKilledPerYear = 3;
var formedString = `The Witcher is of age ${age} and his name is ${name} and has killed over ${age * enemiesKilledPerYear} enemies`;
console.log(formedString);
输出:
"The Witcher is of age 95, and his name is Geralt and has killed over 4750 enemies"
此外,字符串模板可以使用 (``)
生成多行字符串。
var formedString = `Today is a nice day
to learn new things about TypeScript`;
console.log(formedString);
输出:
"Today is a nice day
to learn new things about TypeScript"