TypeScript 中的字符串插值
- TypeScript 中单个表达式的字符串插值
- TypeScript 中多个表达式的字符串插值
- TypeScript 中算术表达式的字符串插值
- TypeScript 中条件表达式的字符串插值
- TypeScript 中字符串内置方法/函数的字符串插值
- TypeScript 中自定义方法/函数的字符串插值
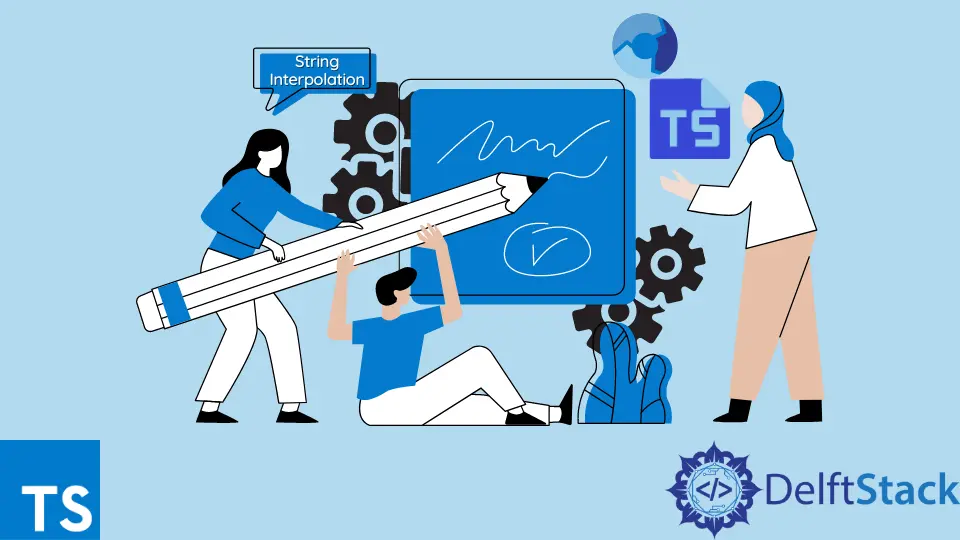
在本教程中,我们将讨论 TypeScript 中的字符串插值,以涵盖基本表达式和复杂表达式的概念。字符串插值意味着调用静态字符串中的变量。
TypeScript 使用反引号进行字符串插值,表示字符串的起点和终点。
在这些反引号内调用字符串,变量括在花括号/花括号中作为 {}
,以美元符号作为 $
开始。
TypeScript 中字符串插值的语法如下所示。
TypeScript 中单个表达式的字符串插值
TypeScript 可以处理字符串插值中的表达式。让我们用一个表达式来开始这个概念。
让我们举个例子:
let name1: string = "John Nash";
console.log(`My name is ${name1}.`);
输出:
My name is John Nash.
在本例中,变量 name1
的值为 John Nash
。变量 name1
具有类型 string
。
现在控制台语句具有静态字符串和变量。该字符串在反引号之间调用,以及变量如何用占位符括起来作为 ${name1}
。
输出语句使用变量 name1
值。这个概念被称为字符串插值。
让我们再举一个例子:
const age: number = 21;
console.log(`John is ${age} years old.`);
输出:
John is 21 years old.
该字符串使用类型为 number
的变量 age
。在输出语句中使用变量值 21
。
TypeScript 中多个表达式的字符串插值
字符串插值也可以处理多个表达式。
例子:
let name1: string = "Smith";
let age: number = 20;
console.log(`My name is ${name1} and I am ${age} years old.`);
输出:
My name is Smith and I am 20 years old.
多重表达式处理是字符串插值。
请注意,类型为 string
和 number
的两个变量 name1
和 age
包含在占位符 ${}
中。
两个以上表达式的另一个示例:
let name1: string = "Jack";
let age: number = 21;
let city: string = "New York";
let country: string = "America";
console.log(`Hi there, my name is ${name1}. I'm ${age} years old and I live in ${city}, ${country}.`);
输出:
Hi there, my name is Jack. I'm 21 years old and I live in New York, America.
TypeScript 中算术表达式的字符串插值
TypeScript 允许在字符串插值中处理算术逻辑表达式。
字符串中的逻辑表达式示例:
const x: number = 10;
const y: number = 7;
console.log(`The sum of two numbers ${x} and ${y} is = ${x+y}.`);
输出:
The sum of two numbers 10 and 7 is = 17.
类型为 number
的变量 x
和 y
用于字符串中的其他表达式。占位符 ${x+y}
计算 x
和 y
的加法。
我们可以计算操作并将其存储在另一个变量中并使用字符串中的变量,而不是计算字符串中的操作。
看看下面的代码来理解这个概念:
const x: number = 7;
const y: number = 11;
const sum: number = x+y;
console.log(`The sum of two numbers ${x} and ${y} is = ${sum}.`);
输出:
The sum of two numbers 7 and 11 is 18.
另一个具有多个算术表达式的示例:
const x: number = 10;
const y: number = 5;
const addition: number = x+y;
const subtraction: number = x-y;
const multiplication: number = x*y;
const division: number = x/y;
console.log(`The two numbers are ${x} and ${y}. The Addition = ${addition} Subtraction= ${subtraction} Multiplication = ${multiplication} Division = ${division} of the two numbers is computed.`);
输出:
The two numbers are 10 and 5. The Addition = 15 Subtraction= 5 Multiplication = 50 Division = 2 of the two numbers is computed.
上面的例子显示了这两个数字的基本算术计算。执行一系列操作,并使用字符串插值在单个字符串中使用结果值。
static
字符串现在使用变量。用户现在可以更改变量的值来更新语句,而不是再次为不同的变量值重写整个代码来打印结果输出语句。
TypeScript 中条件表达式的字符串插值
条件表达式有两个条件,true
或 false
。条件语句以这种方式作为(条件)?真假
。
首先是我们要计算的表达式。在问号 ?
之后有两个条件,第一个是 true
,冒号:
后面的另一个是 false
。
字符串中的条件表达式示例:
const x: number = 12;
const y: number = 13;
const sum: number = a+b;
console.log(`The sum-total of ${a} and ${b} is = ${(sum==25) ? 'Twenty Five' : 'Addition Error'}.`);
输出:
The sum-total of 12 and 13 is = Twenty Five.
有类型为 number
的 a
和 b
变量。在字符串中,我们有一个条件运算符来检查总和是否等于 25。
让我们举一个条件为 false
的例子:
const x: number = 12;
console.log(`The number is x = ${x}, which is ${(x==13) ? 'equal to 13' : 'not equal to 13'}.`);
输出:
The number is x = 12, which is not equal to 13.
条件结果为 false
。因此,它被打印为不等于 13
。
TypeScript 中字符串内置方法/函数的字符串插值
TypeScript 允许在字符串插值中处理内置的字符串方法/函数。
使用字符串的单个内置函数 length 的示例:
let name1: string = "John";
console.log(`The name ${name1} has ${name1.length} alphabets in his name.`);
输出:
The name John has 4 alphabets in his name.
length
函数计算字符串的字符数。
让我们再举一个例子:
let name1: string = "John Nash";
console.log(`The name ${name1} has ${name1.length} characters.`);
输出:
The name John Nash has 9 characters.
上面的输出显示变量 name
有九个字符。任何写在字符串中的东西都被认为是一个字符,包括空格' '
。
使用内置函数 slice() 的示例:
let name1: string = "John Nash";
console.log(`The first name is ${name1.slice(0,4)} and the last name is ${name1.slice(5,9)}.`);
输出:
The first name is John and the last name is Nash.
slice()
函数需要两个参数
。第一个是起点,另一个是终点。
现在让我们以字符串的多个内置函数为例:
let name1: string = "John Nash";
let firstname: string = name1.slice(0,4);
let lastname: string = name1.slice(5,9);
console.log(`The first name ${firstname.toUpperCase()} is in capital alphabets and the last name ${lastname.toLowerCase()} is in small alphabets.`);
输出:
The first name `JOHN` is in capital alphabets, and the last name `nash` is in small alphabets.
有两个函数 toUpperCase() 和 toLowerCase() 分别将字符串更改为大写字母和小写字母。
TypeScript 中自定义方法/函数的字符串插值
TypeScript 允许对自定义函数进行字符串插值。自定义函数是用户创建的函数。
简单自定义函数示例:
function sum(x: number, y: number): number {
return x+y;
};
console.log(`The sum of two numbers is = ${sum(5,7)}.`);
输出:
The sum of two numbers is = 12;
上面的例子显示了一个自定义函数 sum()
,它带有两个参数 x
和 y
类型 number
在一个字符串中使用。
示例 2:
function areaOfCircle(radius: number): number {
let area: number = 3.14 * radius * radius;
return area;
};
console.log(`The area of circle is = ${areaOfCircle(4)}.`);
输出:
The area of circle is = 50.24.
自定义函数是 areaOfCircle()
,它需要一个参数 radius
。该函数返回变量 area
。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn