TypeScript 中的类构造函数类型
Shuvayan Ghosh Dastidar
2023年1月30日
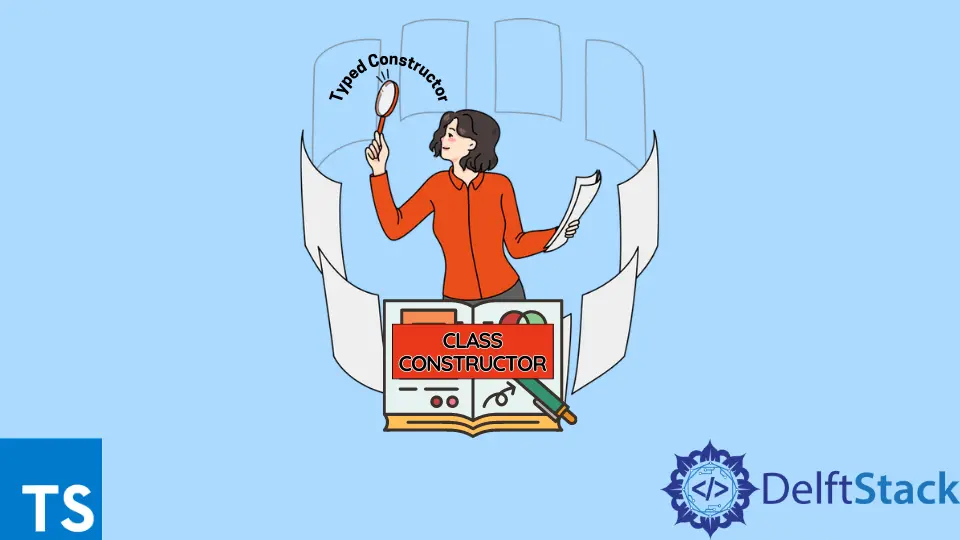
TypeScript 具有强类型语言,因此 TypeScript 中使用的每个变量和对象都有一个类型,这有助于进一步调试和避免应用程序中的运行时错误。本教程将重点介绍如何在 TypeScript 中为构造函数设置类型。
TypeScript 中的类型化构造函数
通常,当我们将对象传递给具有类型的类的构造函数时。我们可以有多种类型,甚至使用联合运算符。
代码:
interface AcademyInfo {
name : string;
country: string;
}
class Academy {
private id;
private info;
private numStudents;
constructor( info : AcademyInfo, id : string | number, numStudents? : number){
this.id = id;
this.info = info;
this.numStudents = numStudents ?? 0;
}
public getAcademyName(){
return this.info.name;
}
}
var academy = new Academy( {name : "Augustine Academy", country: "India"}, "3232dqsx23e", 1000 );
有一个构造函数支持不同类型的参数,例如 AcademyInfo
、string | number
和 number
。在创建类的实例时,所有这些变量都可以传递给类的构造函数。
TypeScript 中类的构造函数的类型
类的构造函数可以有自己的类型。
语法:
type ConstructorType<T> = new (...args : any[]) => T;
上面的语法使用了泛型类型,表示构造函数将返回一个对象,该对象可以是 T
类型的类实例。使用类型化构造函数,可以在 TypeScript 中实现工厂设计模式。
代码:
interface Animal {
speak() : void;
}
interface AnimalConstructor {
new ( speakTerm : string, name : string, legs: number ) : Animal;
}
const createAnimalFactory = (
ctor : AnimalConstructor,
name : string,
legs : number,
speakTerm : string
) => {
return new ctor(speakTerm, name, legs);
};
class Dog implements Animal{
private name;
private legs;
private speakTerm;
constructor(speakTerm : string, name : string, legs : number){
this.speakTerm = speakTerm;
this.legs = legs;
this.name = name;
}
speak(){
console.log( "Dog " + this.speakTerm + " I have " + this.legs + " legs");
}
}
class Cat implements Animal{
private name;
private legs;
private speakTerm;
constructor(speakTerm : string, name : string, legs : number){
this.speakTerm = speakTerm;
this.legs = legs;
this.name = name;
}
speak(){
console.log( "Cat " + this.speakTerm + " I have " + this.legs + " legs");
}
}
const dog = createAnimalFactory(Dog, "dog", 2, "woof");
const cat = createAnimalFactory(Cat, "cat", 2, "meow");
dog.speak();
cat.speak();
输出:
"Dog woof I have 2 legs"
"Cat meow I have 2 legs"
在上面的示例中,我们有两个名为 Dog
和 Cat
的类,它们都实现了相同的接口 Animal
并且具有不同的 speak
方法实现。
在 createAnimalFactory
方法中,可以只传递 Dog
或 Cat
类及其参数来创建该特定类的实例。
这种设计模式对于根据某些用例制作不同的类实例非常有用。