TypeScript 中的類建構函式型別
Shuvayan Ghosh Dastidar
2023年1月30日
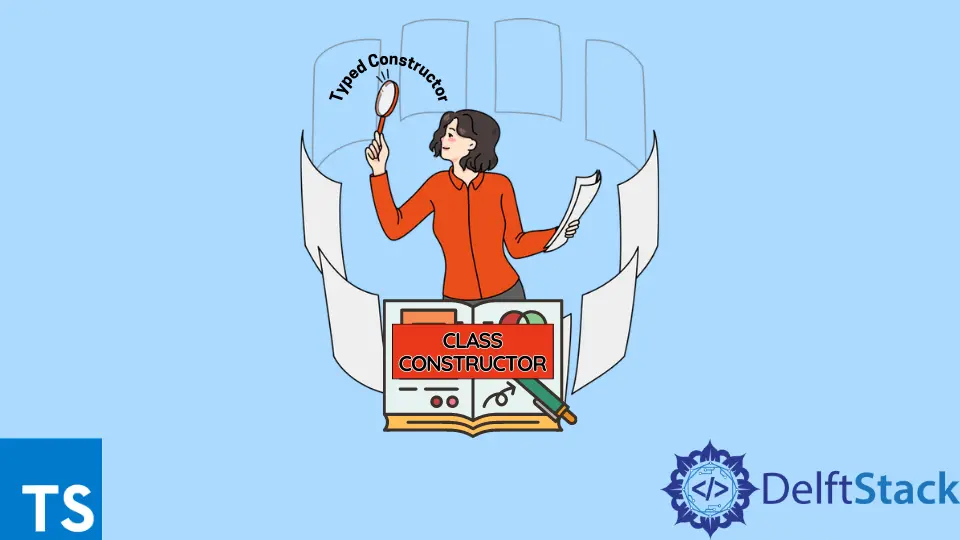
TypeScript 具有強型別語言,因此 TypeScript 中使用的每個變數和物件都有一個型別,這有助於進一步除錯和避免應用程式中的執行時錯誤。本教程將重點介紹如何在 TypeScript 中為建構函式設定型別。
TypeScript 中的型別化建構函式
通常,當我們將物件傳遞給具有型別的類的建構函式時。我們可以有多種型別,甚至使用聯合運算子。
程式碼:
interface AcademyInfo {
name : string;
country: string;
}
class Academy {
private id;
private info;
private numStudents;
constructor( info : AcademyInfo, id : string | number, numStudents? : number){
this.id = id;
this.info = info;
this.numStudents = numStudents ?? 0;
}
public getAcademyName(){
return this.info.name;
}
}
var academy = new Academy( {name : "Augustine Academy", country: "India"}, "3232dqsx23e", 1000 );
有一個建構函式支援不同型別的引數,例如 AcademyInfo
、string | number
和 number
。在建立類的例項時,所有這些變數都可以傳遞給類的建構函式。
TypeScript 中類的建構函式的型別
類的建構函式可以有自己的型別。
語法:
type ConstructorType<T> = new (...args : any[]) => T;
上面的語法使用了泛型型別,表示建構函式將返回一個物件,該物件可以是 T
型別的類例項。使用型別化建構函式,可以在 TypeScript 中實現工廠設計模式。
程式碼:
interface Animal {
speak() : void;
}
interface AnimalConstructor {
new ( speakTerm : string, name : string, legs: number ) : Animal;
}
const createAnimalFactory = (
ctor : AnimalConstructor,
name : string,
legs : number,
speakTerm : string
) => {
return new ctor(speakTerm, name, legs);
};
class Dog implements Animal{
private name;
private legs;
private speakTerm;
constructor(speakTerm : string, name : string, legs : number){
this.speakTerm = speakTerm;
this.legs = legs;
this.name = name;
}
speak(){
console.log( "Dog " + this.speakTerm + " I have " + this.legs + " legs");
}
}
class Cat implements Animal{
private name;
private legs;
private speakTerm;
constructor(speakTerm : string, name : string, legs : number){
this.speakTerm = speakTerm;
this.legs = legs;
this.name = name;
}
speak(){
console.log( "Cat " + this.speakTerm + " I have " + this.legs + " legs");
}
}
const dog = createAnimalFactory(Dog, "dog", 2, "woof");
const cat = createAnimalFactory(Cat, "cat", 2, "meow");
dog.speak();
cat.speak();
輸出:
"Dog woof I have 2 legs"
"Cat meow I have 2 legs"
在上面的示例中,我們有兩個名為 Dog
和 Cat
的類,它們都實現了相同的介面 Animal
並且具有不同的 speak
方法實現。
在 createAnimalFactory
方法中,可以只傳遞 Dog
或 Cat
類及其引數來建立該特定類的例項。
這種設計模式對於根據某些用例製作不同的類例項非常有用。