R 数据框的选定列的总和
-
使用 Base R 的
rowSums()
函数计算数据框选定列的总和 -
使用 Base R 的
apply()
函数计算数据框选定列的总和 - 使用 Tidyverse 函数计算 R 中数据框选定列的总和
- 结论
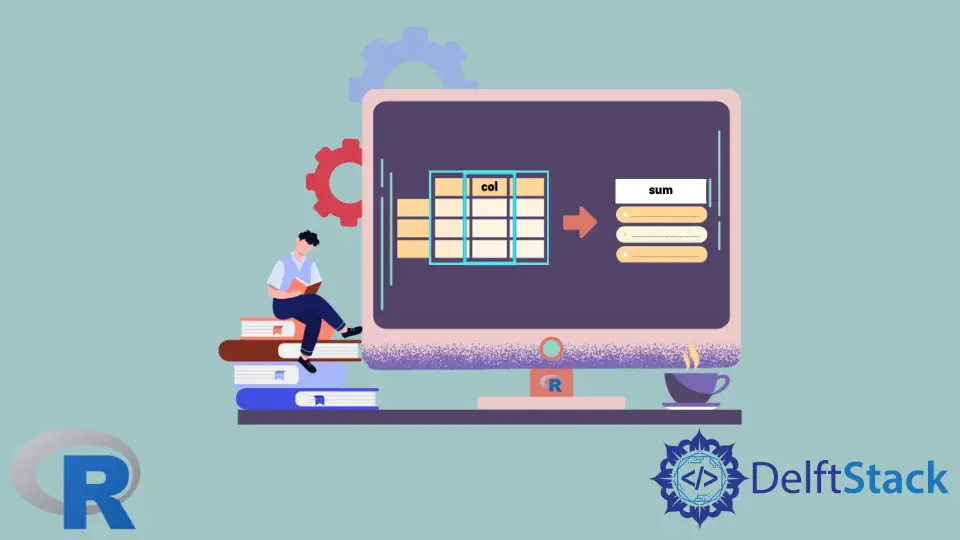
在很多情况下,我们想使用其他列的值创建一个新列。本文将教授如何创建一个新列来计算 R 中所选数据框列的总和。
我们将学习创建新列的三种方法:使用基础 R 中的 rowSums()
和 apply()
以及 Tidyverse 中的一组函数。
我们可以根据使用的函数以不同的方式指定要添加的列:直接在函数中、使用字符串向量或模式匹配函数。
我们将首先创建一个数据框。该数据框没有行名,也没有缺失值。
示例代码:
# Create five variables.
Student = c("Student A", "Student B", "Student C")
Hobby = c("Music", "Sports", "Cycling")
Maths = c(40, 35, 30)
Statistics = c(30, 35, 20)
Programming = c(25, 20, 35)
# Create a data frame from the variables.
df_students = data.frame(Student, Hobby, Maths, Statistics, Programming)
# View the data frame.
df_students
使用 Base R 的 rowSums()
函数计算数据框选定列的总和
我们将使用 data_frame$new_column
语法创建一个新列,并使用 rowSums()
函数为其赋值。要添加的列将使用子集语法直接在函数中给出。
示例代码:
# This adds the new column to the data frame.
df_students$myRowSums = rowSums(df_students[,c("Maths", "Statistics", "Programming")])
# View the data frame with the added column.
df_students
# We can also give a vector of column positions.
# df_students$myRowSums = rowSums(df_students[,c(3:5)])
输出:
> # View the data frame with the added column.
> df_students
Student Hobby Maths Statistics Programming myRowSums
1 Student A Music 40 30 25 95
2 Student B Sports 35 35 20 90
3 Student C Cycling 30 20 35 85
我们还可以保存列的名称以添加为字符串向量。我们可以将此向量传递给 rowSums()
函数。
示例代码:
# Save the list of columns as a vector of strings.
col_list = c("Maths", "Statistics", "Programming")
# Pass the vector of strings to the subsetting square brackets.
df_students$myRowSums = rowSums(df_students[,col_list])
# View the data frame with the added column.
df_students
文档指出,rowSums()
函数等效于带有 FUN = sum
的 apply()
函数,但要快得多。
它指出 rowSums()
函数模糊了一些 NaN
或 NA
的细微之处。
使用 Base R 的 apply()
函数计算数据框选定列的总和
我们将这三个参数传递给 apply()
函数。
- 数据框所需的列。
- 要保留的数据框的维度。
1
表示行。 - 我们要计算的函数,
sum
。
示例代码:
# We will recreate the data frame from the variables.
df_students = data.frame(Student, Hobby, Maths, Statistics, Programming)
# In base R, we can delete a column by setting its name to NULL.
# df_students$myRowSums = NULL
# A new column gets created.
df_students$myApplySums = apply(df_students[,col_list], 1, sum)
# View the data frame with the added column.
df_students
我们还可以将要添加的列作为字符串或位置的向量直接添加到方括号中。
示例代码:
# Names of columns as a vector of strings.
df_students$myApplySums = apply(df_students[,c("Maths", "Statistics", "Programming")], 1, sum)
# Vector of columns positions.
df_students$myApplySums = apply(df_students[,c(3, 4, 5)], 1, sum)
使用 Tidyverse 函数计算 R 中数据框选定列的总和
我们可以将 dplyr
的 mutate()
函数与 Tidyverse 中的其他函数结合使用来创建总和列。
在使用 Tidyverse 方法时,我们需要了解一些细节。Tibbles 删除行名称,并且对有效数字、尾随零和尾随小数具有不同的默认值。
首先,我们需要加载 dplyr
包并创建一个 tibble。
请注意我们如何在代码中使用以下内容。
- 管道运算符,
%>%
,以避免嵌套某些函数。 rowwise()
使其他函数在行上工作。mutate()
添加列。sum()
用于加法。c_across()
旨在与rowwise()
一起使用。all_of()
从字符向量中选择值。
rowwise()
是一种分组类型。使用后,我们可能需要使用 ungroup(data_frame_name)
并将未分组的版本保存为对象。
示例代码:
# We will recreate the data frame from the variables.
df_students = data.frame(Student, Hobby, Maths, Statistics, Programming)
# Load the dplyr package.
library(dplyr)
# Create a tibble from the data frame.
# This could have been done with the next step but obscured the main point.
tb_students = as_tibble(df_students)
# We have to assign the RHS to an object to save the column to the object.
# It can be the same as the original tibble.
tb_students = tb_students %>% rowwise() %>% mutate(myTidySum = sum(c_across(all_of(col_list))))
# View the rowwise tibble with the added column.
tb_students
输出:
> # View the rowwise tibble with the added column.
> tb_students
# A tibble: 3 x 6
# Rowwise:
Student Hobby Maths Statistics Programming myTidySum
<chr> <chr> <dbl> <dbl> <dbl> <dbl>
1 Student A Music 40 30 25 95
2 Student B Sports 35 35 20 90
3 Student C Cycling 30 20 35 85
上面的示例使用了四个函数。它比前几节的方法更复杂。
但是 Tidyverse 方法的一大优势是它提供了许多指定列的方法。
根据我们的需要,我们可以使用选择辅助函数,例如 starts_with()
、contains()
、where()
,使用联合和交集组合选择,指定我们不想选择的列,等等在。
下面的示例代码提供了五种等效方法来实现相同的结果。由于这种灵活性,我们需要熟悉这种方法。
示例代码:
tb_students = as_tibble(df_students)
# Take the union of the column names.
tb_students = tb_students %>% rowwise() %>% mutate(myTidySum = sum(c_across(Maths | Statistics | Programming)))
tb_students = as_tibble(df_students)
# Give a range of columns as a range of names.
tb_students = tb_students %>% rowwise() %>% mutate(myTidySum = sum(c_across(Maths:Programming)))
tb_students = as_tibble(df_students)
# Give a range of columns as a range of column positions.
tb_students = tb_students %>% rowwise() %>% mutate(myTidySum = sum(c_across(3:5)))
tb_students = as_tibble(df_students)
# Select all columns having 'at' or 'am'
tb_students = tb_students %>% rowwise() %>% mutate(myTidySum = sum(c_across(contains('at') | contains('am'))))
tb_students = as_tibble(df_students)
# Select all columns except Student and Hobby.
# Make sure the tibble only has the required columns before running the next line.
tb_students = tb_students %>% rowwise() %>% mutate(myTidySum = sum(c_across(!c(Student, Hobby))))
参考和帮助
请参阅 R for Data Science 中的章节以了解管道运算符。
有关 rowwise()
和 c_across()
的帮助,请参阅 Tidyverse 函数参考。
有关 tidyselect 辅助函数,请参阅 tidyselect 选择语言。
在 R Studio 中,有关 rowSums()
或 apply()
的帮助,请单击 Help > Search R Help
并在搜索框中键入不带括号的函数名称。或者,在 R 控制台的命令提示符处键入一个问号,后跟函数名称。
结论
rowSums()
和 apply()
函数使用简单。要添加的列可以使用名称或列位置直接在函数中指定,也可以作为字符向量提供。
Tidyverse 方法虽然有点复杂,但提供了许多替代方法来指定要添加的列。
我们可以选择最适合我们需求的方法。