Python 中的 sigmoid 函数
-
在 Python 中使用
math
模块实现 Sigmoid 函数 -
在 Python 中使用
numpy.exp()
方法实现 Sigmoid 函数 -
在 Python 中使用
SciPy
库实现 Sigmoid 函数
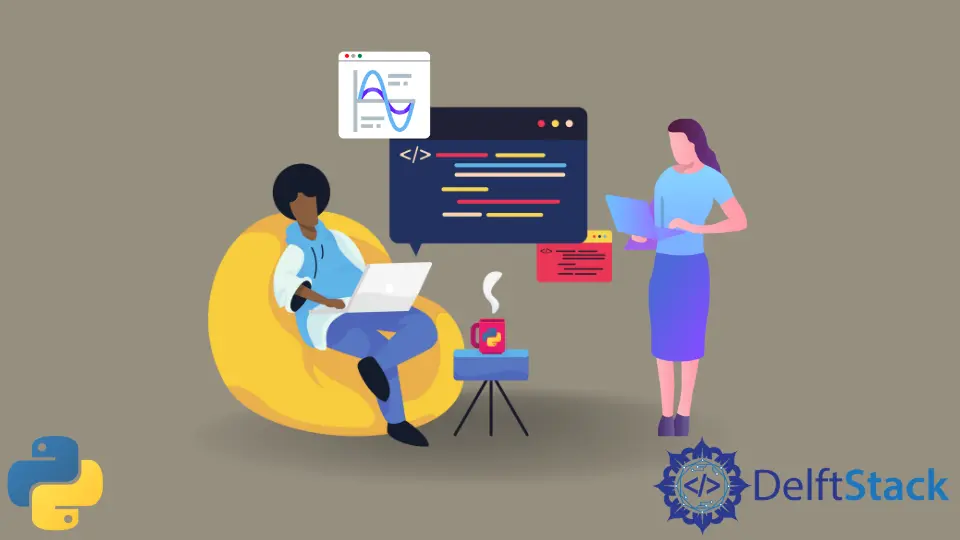
在本教程中,我们将研究在 Python 中使用 Sigmoid 函数的各种方法。sigmoid 函数是数学逻辑函数。它通常用于统计,音频信号处理,生物化学以及人工神经元的激活功能。S 形函数的公式为 F(x) = 1/(1 + e^(-x))
。
在 Python 中使用 math
模块实现 Sigmoid 函数
我们可以使用 math
模块在 Python 中实现自己的 Sigmoid 函数。我们需要 math
模块中的 math.exp()
方法来实现 Sigmoid 函数。
下面的示例代码演示了如何在 Python 中使用 Sigmoid 函数。
import math
def sigmoid(x):
sig = 1 / (1 + math.exp(-x))
return sig
该实现方式的问题在于它在数值上不稳定,并且可能发生溢出。
下面给出了 Python 中 Sigmoid 函数的数值稳定实现的示例代码。
import math
def stable_sigmoid(x):
if x >= 0:
z = math.exp(-x)
sig = 1 / (1 + z)
return sig
else:
z = math.exp(x)
sig = z / (1 + z)
return sig
在 Python 中使用 numpy.exp()
方法实现 Sigmoid 函数
我们还可以使用 Python 中的 numpy.exp()
方法来实现 Sigmoid 函数。像使用 math.exp()
方法实现 sigmoid 函数一样,我们也可以使用 numpy.exp()
方法实现 sigmoid 函数。
numpy.exp()
方法优于 math.exp()
的优点是,除了整数或浮点数之外,它还可以处理数组形状的输入。
以下是在 Python 中使用 numpy.exp()
方法的常规 sigmoid 函数的实现。
import numpy as np
def sigmoid(x):
z = np.exp(-x)
sig = 1 / (1 + z)
return sig
对于 Sigmoid 函数的数值稳定实现,我们首先需要检查输入数组的每个值的值,然后传递 Sigmoid 的值。为此,我们可以使用 np.where()
方法,如下面的示例代码所示。
import numpy as np
def stable_sigmoid(x):
sig = np.where(x < 0, np.exp(x) / (1 + np.exp(x)), 1 / (1 + np.exp(-x)))
return sig
在 Python 中使用 SciPy
库实现 Sigmoid 函数
我们还可以通过在 SciPy
库中简单地导入名为 expit
的 Sigmoid 函数来使用 Python 的 Sigmoid 函数的 SciPy
版本。
下面的示例代码演示了如何使用 SciPy
库使用 Sigmoid 函数:
from scipy.special import expit
x = 0.25
sig = expit(x)
expit()
方法比上面的实现要慢。expit()
方法的优点是它可以自动处理各种类型的输入,例如列表和数组等。
from scipy.special import expit
sig = expit(np.array([0.25, 0.5, 0.6, 0.7, 0.4]))
print(sig)
输出:
[0.5621765 0.62245933 0.64565631 0.66818777 0.59868766]