Python 中的 sigmoid 函式
-
在 Python 中使用
math
模組實現 Sigmoid 函式 -
在 Python 中使用
numpy.exp()
方法實現 Sigmoid 函式 -
在 Python 中使用
SciPy
庫實現 Sigmoid 函式
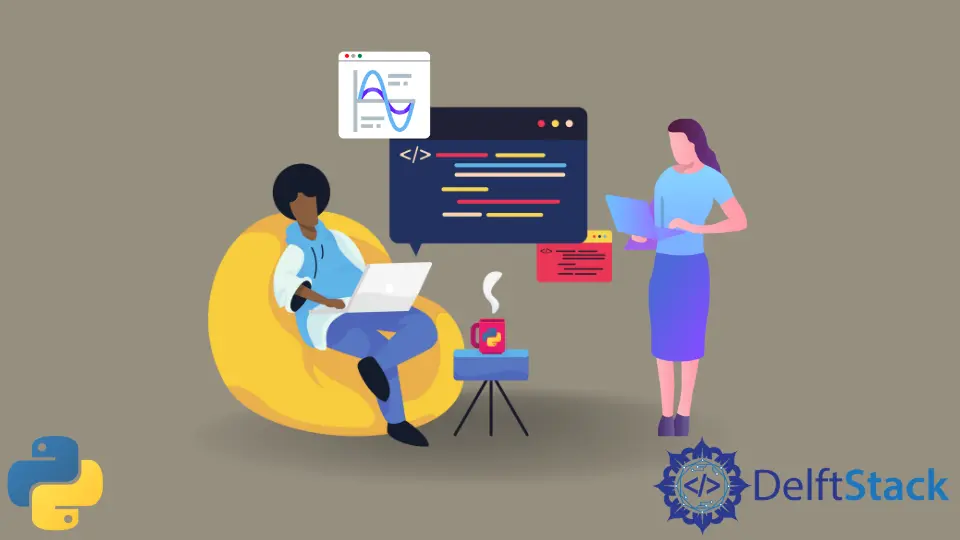
在本教程中,我們將研究在 Python 中使用 Sigmoid 函式的各種方法。sigmoid 函式是數學邏輯函式。它通常用於統計,音訊訊號處理,生物化學以及人工神經元的啟用功能。S 形函式的公式為 F(x) = 1/(1 + e^(-x))
。
在 Python 中使用 math
模組實現 Sigmoid 函式
我們可以使用 math
模組在 Python 中實現自己的 Sigmoid 函式。我們需要 math
模組中的 math.exp()
方法來實現 Sigmoid 函式。
下面的示例程式碼演示瞭如何在 Python 中使用 Sigmoid 函式。
import math
def sigmoid(x):
sig = 1 / (1 + math.exp(-x))
return sig
該實現方式的問題在於它在數值上不穩定,並且可能發生溢位。
下面給出了 Python 中 Sigmoid 函式的數值穩定實現的示例程式碼。
import math
def stable_sigmoid(x):
if x >= 0:
z = math.exp(-x)
sig = 1 / (1 + z)
return sig
else:
z = math.exp(x)
sig = z / (1 + z)
return sig
在 Python 中使用 numpy.exp()
方法實現 Sigmoid 函式
我們還可以使用 Python 中的 numpy.exp()
方法來實現 Sigmoid 函式。像使用 math.exp()
方法實現 sigmoid 函式一樣,我們也可以使用 numpy.exp()
方法實現 sigmoid 函式。
numpy.exp()
方法優於 math.exp()
的優點是,除了整數或浮點數之外,它還可以處理陣列形狀的輸入。
以下是在 Python 中使用 numpy.exp()
方法的常規 sigmoid 函式的實現。
import numpy as np
def sigmoid(x):
z = np.exp(-x)
sig = 1 / (1 + z)
return sig
對於 Sigmoid 函式的數值穩定實現,我們首先需要檢查輸入陣列的每個值的值,然後傳遞 Sigmoid 的值。為此,我們可以使用 np.where()
方法,如下面的示例程式碼所示。
import numpy as np
def stable_sigmoid(x):
sig = np.where(x < 0, np.exp(x) / (1 + np.exp(x)), 1 / (1 + np.exp(-x)))
return sig
在 Python 中使用 SciPy
庫實現 Sigmoid 函式
我們還可以通過在 SciPy
庫中簡單地匯入名為 expit
的 Sigmoid 函式來使用 Python 的 Sigmoid 函式的 SciPy
版本。
下面的示例程式碼演示瞭如何使用 SciPy
庫使用 Sigmoid 函式:
from scipy.special import expit
x = 0.25
sig = expit(x)
expit()
方法比上面的實現要慢。expit()
方法的優點是它可以自動處理各種型別的輸入,例如列表和陣列等。
from scipy.special import expit
sig = expit(np.array([0.25, 0.5, 0.6, 0.7, 0.4]))
print(sig)
輸出:
[0.5621765 0.62245933 0.64565631 0.66818777 0.59868766]