如何更改 Tkinter 按钮的大小
Jinku Hu
2024年2月15日
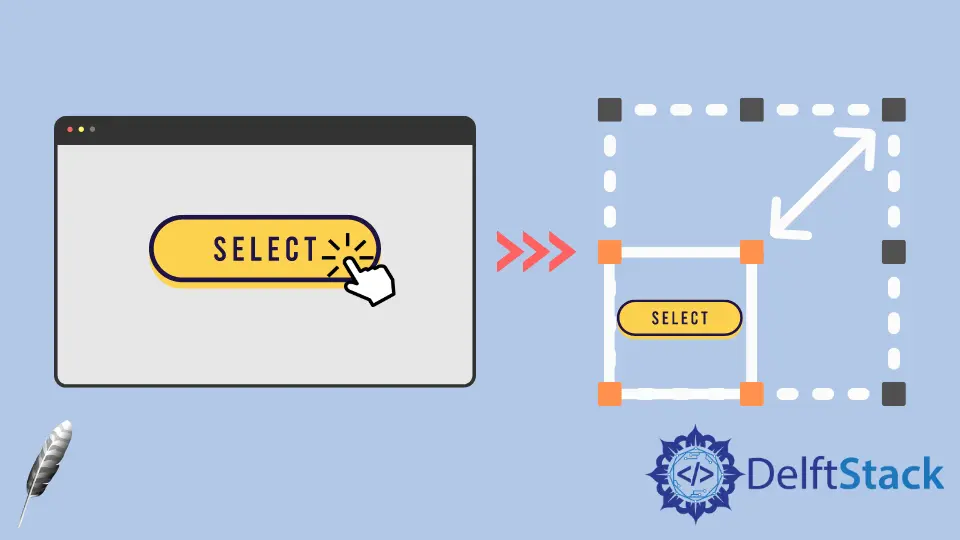
Tkinter 按钮 Button
控件的 height
和 width
选项指定初始化期间创建的按钮的大小。初始化之后,我们仍然可以使用 configure
方法来配置 height
和 width
选项,以编程方式更改 Tkinter 按钮 Button
控件的大小。
指定 height
和 width
选项以设置按钮大小
tk.Button(self, text="", height=20, width=20)
以文本单位为单位,将 height
和 width
设置为 20
。在默认的系统字体中,水平文本单位等于字符 0
的宽度,垂直文本单位等于 0
的高度。
注意
Tkinter 使用文本单位而不是英寸或像素来测量宽度和高度的原因是,该文本单位可确保 Tkinter 在不同平台上的一致行为。
完整的工作代码
import tkinter as tk
import tkinter.font as tkFont
app = tk.Tk()
app.geometry("400x200")
buttonExample1 = tk.Button(app, text="Button 1", width=10, height=10)
buttonExample2 = tk.Button(app, text="Button 2", width=10, height=10)
buttonExample1.pack(side=tk.LEFT)
buttonExample2.pack(side=tk.RIGHT)
app.mainloop()
如上所示,按钮的高度和宽度在像素中是不同的,尽管其 height
和 width
都设置为 10
。
以像素为单位设置 Tkinter 按钮 Button
的宽度和高度
如果需要以像素 pixel
为单位指定 Tkinter 按钮控件的宽度和/或高度,则可以向按钮
中添加虚拟的不可见的 1x1
像素图像。然后,将以像素为单位测量宽度和高度。
tk.Button(app, text="Button 1", image=pixelVirtual, width=100, height=100, compound="c")
如果不可见的图像和文本应该在按钮的中央,我们还需要将 compound
选项设置为 c
或等效的 tk.CENTER
。如果未配置 compound
,则 text
将不会显示在按钮中。
import tkinter as tk
import tkinter.font as tkFont
app = tk.Tk()
app.geometry("300x100")
fontStyle = tkFont.Font(family="Lucida Grande", size=20)
labelExample = tk.Label(app, text="20", font=fontStyle)
pixelVirtual = tk.PhotoImage(width=1, height=1)
buttonExample1 = tk.Button(
app, text="Increase", image=pixelVirtual, width=100, height=100, compound="c"
)
buttonExample2 = tk.Button(
app, text="Decrease", image=pixelVirtual, width=100, height=100, compound="c"
)
buttonExample1.pack(side=tk.LEFT)
buttonExample2.pack(side=tk.RIGHT)
app.mainloop()
初始化后更改 Tkinter 按钮 Button
大小
在创建按钮控件之后,configure
方法可以设置 width
和/或 height
选项来更改按钮的大小。
buttonExample1.configure(height=100, width=100)
它将 buttonExample1
的高度和宽度设置为 100。
初始化后更改按钮 Button
大小的完整工作示例
import tkinter as tk
import tkinter.font as tkFont
app = tk.Tk()
app.geometry("600x500")
def decreaseSize():
buttonExample1.configure(height=100, width=100)
def increaseSize():
buttonExample2.configure(height=400, width=400)
pixelVirtual = tk.PhotoImage(width=1, height=1)
buttonExample1 = tk.Button(
app,
text="Decrease Size",
image=pixelVirtual,
width=200,
height=200,
compound="c",
command=decreaseSize,
)
buttonExample2 = tk.Button(
app,
text="Increase Size",
image=pixelVirtual,
width=200,
height=200,
compound=tk.CENTER,
command=increaseSize,
)
buttonExample1.pack(side=tk.LEFT)
buttonExample2.pack(side=tk.RIGHT)
app.mainloop()
作者: Jinku Hu