如何将 Pandas Dataframe 转换为 NumPy 数组
Asad Riaz
2023年1月30日
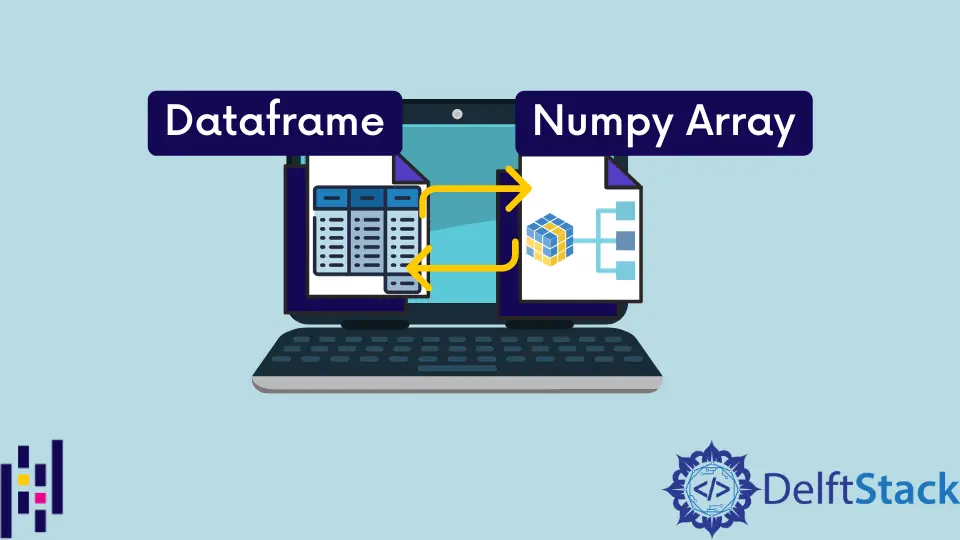
我们将来介绍 to_numpy()
方法将 pandas.Dataframe
转换为 NumPy
数组,这是从 pandas v0.24.0 引入的,替换了旧的 .values
方法。我们可以在 Index
,Series
和 DataFrame
对象上定义 to_numpy
。
旧的 DataFrame.values
具有不一致的行为,根据 PandasAPI 文档,我们不建议使用它。但是,如果你使用的是旧版本,我们将研究此方法的例子。
另外一个不推荐使用的旧方法是 DataFrame.as_matrix()
,请不要使用它!
我们还将介绍另一种使用 DataFrame.to_records()
方法将给定的 DataFrame
转换为 NumPy
记录数组的方法。
to_numpy
方法将 DataFrame
转换为 NumPy
数组
pandas.Dataframe
是具有行和列的二维表格数据结构。可以使用 to_numpy
方法将该数据结构转换为 NumPy
数组:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
nmp = df.to_numpy()
print(nmp)
print(type(nmp))
输出:
[[5 5 1 3]
[1 6 6 0]
[9 1 2 0]
[9 3 5 3]
[7 9 4 9]
[8 1 8 9]]
<class 'numpy.ndarray'>
可以通过以下方法使用 Dataframe.values
方法来实现:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
nmp = df.values
print(nmp)
print(type(nmp))
输出:
[[8 8 5 0]
[1 7 7 5]
[0 2 4 2]
[6 8 0 7]
[6 4 5 1]
[1 8 4 7]]
<class 'numpy.ndarray'>
如果我们想在 NumPy
数组中包含 indexes
,则需要对 Dataframe.values
应用 reset_index()
:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
nmp = df.reset_index().values
print(nmp)
print(type(nmp))
输出:
[[0 1 0 3 7]
[1 8 2 5 1]
[2 2 2 7 3]
[3 3 4 3 7]
[4 5 4 4 3]
[5 2 9 7 6]]
<class 'numpy.ndarray'>
to_records()
方法将 DataFrame
转换为 NumPy
记录数组
如果你需要 dtypes
,则 to_records()
是最好的选择。在性能方面,to_numpy()
和 to_records()
几乎相同:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
nmp = df.to_records()
print(nmp)
print(type(nmp))
输出:
[(0, 0, 4, 6, 1)
(1, 3, 1, 7, 1)
(2, 9, 1, 6, 4)
(3, 1, 4, 6, 9)
(4, 9, 1, 3, 9)
(5, 2, 5, 7, 9)]
<class 'numpy.recarray'>