如何將 Pandas Dataframe 轉換為 NumPy 陣列
Asad Riaz
2023年1月30日
Pandas
Pandas DataFrame
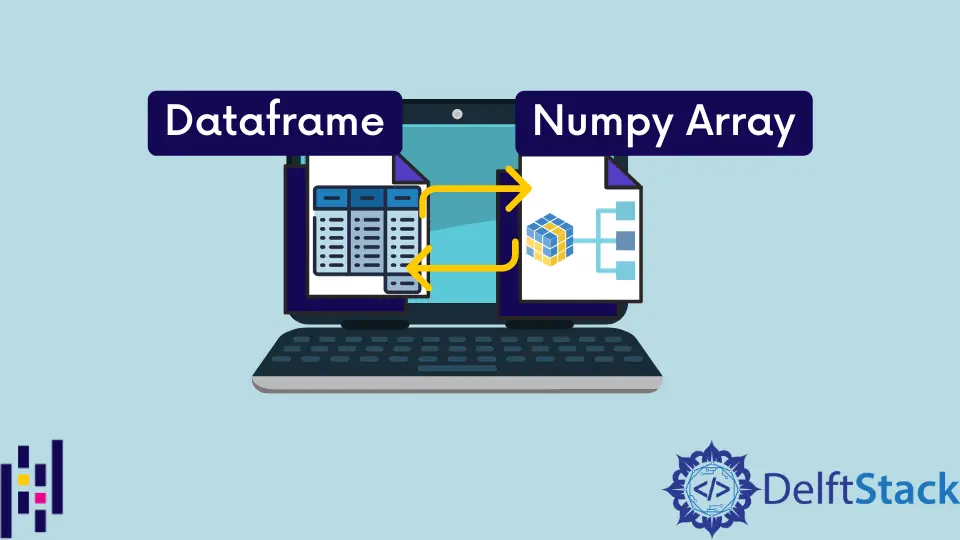
我們將來介紹 to_numpy()
方法將 pandas.Dataframe
轉換為 NumPy
陣列,這是從 pandas v0.24.0 引入的,替換了舊的 .values
方法。我們可以在 Index
,Series
和 DataFrame
物件上定義 to_numpy
。
舊的 DataFrame.values
具有不一致的行為,根據 PandasAPI 文件,我們不建議使用它。但是,如果你使用的是舊版本,我們將研究此方法的例子。
另外一個不推薦使用的舊方法是 DataFrame.as_matrix()
,請不要使用它!
我們還將介紹另一種使用 DataFrame.to_records()
方法將給定的 DataFrame
轉換為 NumPy
記錄陣列的方法。
to_numpy
方法將 DataFrame
轉換為 NumPy
陣列
pandas.Dataframe
是具有行和列的二維表格資料結構。可以使用 to_numpy
方法將該資料結構轉換為 NumPy
陣列:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
nmp = df.to_numpy()
print(nmp)
print(type(nmp))
輸出:
[[5 5 1 3]
[1 6 6 0]
[9 1 2 0]
[9 3 5 3]
[7 9 4 9]
[8 1 8 9]]
<class 'numpy.ndarray'>
可以通過以下方法使用 Dataframe.values
方法來實現:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
nmp = df.values
print(nmp)
print(type(nmp))
輸出:
[[8 8 5 0]
[1 7 7 5]
[0 2 4 2]
[6 8 0 7]
[6 4 5 1]
[1 8 4 7]]
<class 'numpy.ndarray'>
如果我們想在 NumPy
陣列中包含 indexes
,則需要對 Dataframe.values
應用 reset_index()
:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
nmp = df.reset_index().values
print(nmp)
print(type(nmp))
輸出:
[[0 1 0 3 7]
[1 8 2 5 1]
[2 2 2 7 3]
[3 3 4 3 7]
[4 5 4 4 3]
[5 2 9 7 6]]
<class 'numpy.ndarray'>
to_records()
方法將 DataFrame
轉換為 NumPy
記錄陣列
如果你需要 dtypes
,則 to_records()
是最好的選擇。在效能方面,to_numpy()
和 to_records()
幾乎相同:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
nmp = df.to_records()
print(nmp)
print(type(nmp))
輸出:
[(0, 0, 4, 6, 1)
(1, 3, 1, 7, 1)
(2, 9, 1, 6, 4)
(3, 1, 4, 6, 9)
(4, 9, 1, 3, 9)
(5, 2, 5, 7, 9)]
<class 'numpy.recarray'>
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe