PHP 在 MySQL 表中的 UPDATE 查询
John Wachira
2023年1月30日
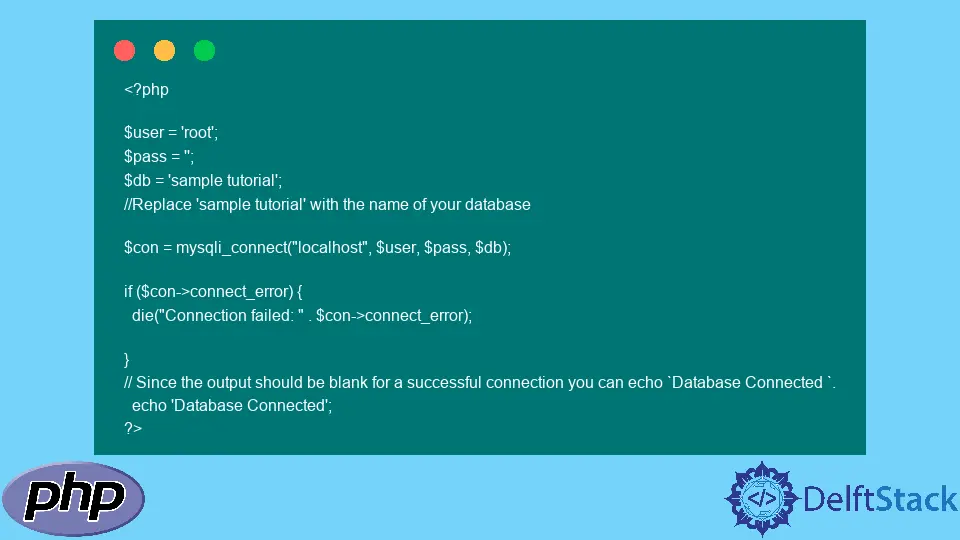
在本教程中,我们将介绍 PHP UPDATE
语句。我们将探讨如何使用此语句更新 MySQL 表中的现有记录。
在 PHP 中使用 mysqli_connect
连接到包含 MySQL 表的数据库
首先,我们需要了解如何连接到 MySQL 表数据库。参见示例:
<?php
$user = 'root';
$pass = '';
$db = 'sample tutorial';
//Replace 'sample tutorial' with the name of your database
$con = mysqli_connect("localhost", $user, $pass, $db);
if ($con->connect_error) {
die("Connection failed: " . $con->connect_error);
}
// Since the output should be blank for a successful connection you can echo `Database Connected `.
echo 'Database Connected';
?>
输出:
Database Connected
我们的数据库 sample tutorial
有一个名为 parkinglot1
的表。下面是表格的样子。
CarID | BrandName | OwnersName | RegistrationNumber |
---|---|---|---|
1 | Benz | John | KDD125A |
2 | Porsche | Ann | KCK345Y |
3 | Buggatti | Loy | KDA145Y |
4 | Audi | Maggie | KCA678I |
5 | Filder | Joseph | KJG998U |
6 | Tesla | Tesla | KMH786Y |
假设 John 的汽车的 RegistrationNumber
发生了变化。我们会将注册号从 KDD125A
更改为 KBK639F
。
使用 Update
查询更新 Mysql 表中的现有记录
<?php
$user = 'root';
$pass = '';
$db = 'sample tutorial';
//Replace 'sample tutorial' with the name of your database
$con = mysqli_connect("localhost", $user, $pass, $db);
if ($con->connect_error) {
die("Connection failed: " . $con->connect_error);
}
// Since the output should be blank for a sucessful connection you can echo `Database Connected `.
echo 'Database Connected';
echo '<br>';
//Update
$sql = "UPDATE parkinglot1 SET RegistrationNumber = 'KBK639F' WHERE CarID = 1";
// Check if the update was successful.
if ($con->query($sql) === TRUE){
echo 'Registration Number Updated Successfully';
} else{
echo 'Error Updating the Registration Number:' . $con->error;
}
?>
输出:
Database Connected
Registration Number Updated Successfully
这是更新后我们的表格的样子:
CarID | BrandName | OwnersName | RegistrationNumber |
---|---|---|---|
1 | Benz | John | KBK639F |
2 | Porsche | Ann | KCK345Y |
3 | Buggatti | Loy | KDA145Y |
4 | Audi | Maggie | KCA678I |
5 | Filder | Joseph | KJG998U |
6 | Tesla | Tesla | KMH786Y |
注意
你应该使用
WHERE
语句指定在何处进行更改,如我们的代码所示;否则,你最终可能会更新所有内容。作者: John Wachira
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn