从 PHP 中的 MySQL 表中选择计数函数
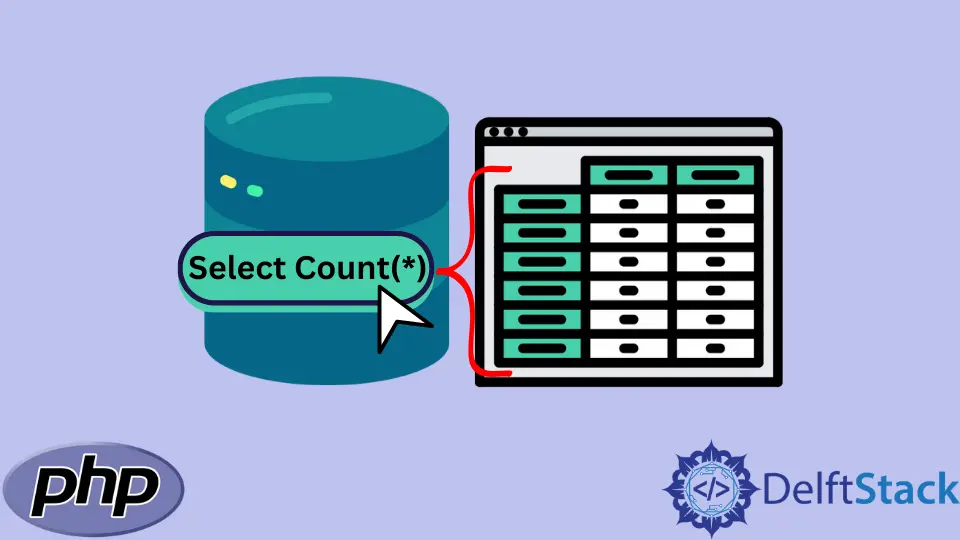
本教程将介绍 select count(*)
函数,计算行数,并从 PHP 中的 MySQL 表中获取查询返回的总记录。与教程一起工作的示例代码及其输出。
第一步是用 PHP 连接到 MySQL 表数据库。下面是我们用来连接数据库的示例代码。
示例代码 1:
<?php
$user = 'root';
$pass = '';
$db = 'sample tutorial';
//Replace 'sample tutorial' with the name of your database
$con = mysqli_connect("localhost", $user, $pass, $db);
//The code does not have an output, so we decided to print 'Database Connected'
echo "Database Connected";
?>
请记住用包含你的 MySQL 表的数据库的名称替换 sample tutorial
。
上面的代码在连接成功时通常不显示任何输出。在我们的例子中,我们决定打印 Database Connected
作为可选输出。
我们数据库中的表如下:
CarID | BrandName | OwnersName | RegistrationNumber |
---|---|---|---|
1 | Benz | John | KDD125A |
2 | Porsche | Ann | KCK345Y |
3 | Buggatti | Loy | KDA145Y |
4 | Audi | Maggie | KCA678I |
5 | Filder | Joseph | KJG998U |
6 | Tesla | Tesla | KMH786Y |
使用 PHP 中的 Select Count(*)
函数计算 MySQL 表中的行数
下面是使用 PHP 的 select count(*)
函数计算 MySQL 表中行数的示例代码。
示例代码 2:
<?php
$user = 'root';
$pass = '';
$db = 'sample tutorial';
//Replace 'sample tutorial' with the name of your database
$con = mysqli_connect("localhost", $user, $pass, $db);
$sql = "SELECT * FROM parkinglot1";
$result = mysqli_query($con, $sql);
$num_rows = mysqli_num_rows($result);
printf("Number of rows in the table : %d\n", $num_rows);
?>
输出:
Number of rows in the table : 6
使用 PHP 中的 Select Count(*)
函数在 MySQL 表中显示查询返回的总记录
select count(*)
函数可以获取数据库表中查询返回的总记录数。
下面是一个示例代码,用于显示 BrandName
类别中的总记录。
示例代码 3:
<?php
$user = 'root';
$pass = '';
$db = 'sample tutorial';
$con = mysqli_connect("localhost", $user, $pass, $db);
$sql = "SELECT COUNT(BrandName) AS total FROM parkinglot1";
$result = mysqli_query($con, $sql);
$data = mysqli_fetch_assoc($result);
echo $data['total']
?>
输出:
6
请记住用包含你的 MySQL 表的数据库的名称替换 sample tutorial
。
OwnersName
类别的另一个示例代码和输出。
示例代码 4:
<?php
$user = 'root';
$pass = '';
$db = 'sample tutorial';
$con = mysqli_connect("localhost", $user, $pass, $db);
$sql = "SELECT COUNT(OwnersName) AS total FROM parkinglot1";
$result = mysqli_query($con, $sql);
$data = mysqli_fetch_assoc($result);
echo $data['total']
?>
输出:
6
我们使用 SELECT * FROM parklot1
来计算行数,并使用 SELECT COUNT(*) AS total FROM parklot1
来显示从我们的 MySQL 表中查询返回的总记录。
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn