Python 中 NumPy 数组的一位有效编码
- 在 Python 中使用 NumPy 模块对 NumPy 数组执行一位有效编码
-
在 Python 中使用
sklearn
模块的 NumPy 数组上执行一位有效编码 -
在 Python 中使用
pandas
模块的 NumPy 数组上执行一位有效编码 -
在 Python 中使用
keras
模块的 NumPy 数组上执行一位有效编码
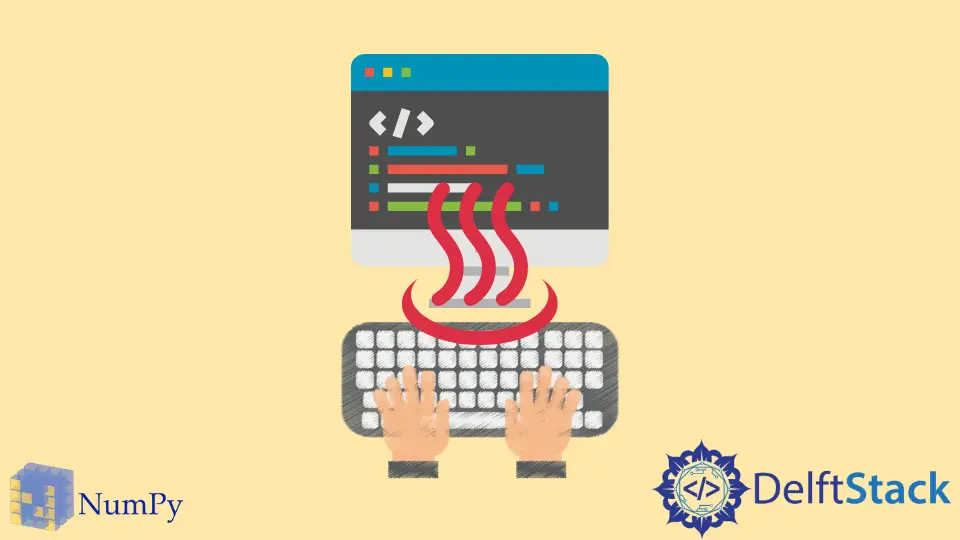
Python 具有可用于机器学习的庞大框架。我们可以轻松地训练和测试模型。但是,当涉及分类数据时,某些算法无法使用此类数据标签,并且需要数字值。
因此,一位有效编码是在将数据用于算法之前对其进行编码的一种高度使用的技术。
在本教程中,我们将学习如何对 numpy 数组执行一位有效编码。
在 Python 中使用 NumPy 模块对 NumPy 数组执行一位有效编码
在这种方法中,我们将生成一个包含编码数据的新数组。我们将使用 numpy.zeros()
函数创建所需大小的 0 数组。然后,使用 numpy.arange()
函数在对应的位置将 0 替换为 1。
例如,
import numpy as np
a = np.array([1, 0, 3])
b = np.zeros((a.size, a.max() + 1))
b[np.arange(a.size), a] = 1
print(b)
输出:
[[0. 1. 0. 0.]
[1. 0. 0. 0.]
[0. 0. 0. 1.]]
我们还可以使用 eye()
函数对数组执行一位有效编码。默认情况下,它返回一个二维,在主对角线处为 1,在其他位置为 0。我们可以使用此方法并指定我们希望 1 的位置,如下所示。
import numpy as np
values = [1, 0, 3]
n_values = np.max(values) + 1
print(np.eye(n_values)[values])
输出:
[[0. 1. 0. 0.]
[1. 0. 0. 0.]
[0. 0. 0. 1.]]
在 Python 中使用 sklearn
模块的 NumPy 数组上执行一位有效编码
sklearn.preprocessing.LabelBinarizer
是 Python 中可用的类,可以有效地执行此编码。它用于通过将多标签转换为数字形式来进行二进制化。我们将使用 transform()
函数使用此类的对象来转换数据。
以下代码对此进行了说明。
import sklearn.preprocessing
import numpy as np
a = np.array([1, 0, 3])
label_binarizer = sklearn.preprocessing.LabelBinarizer()
label_binarizer.fit(range(max(a) + 1))
b = label_binarizer.transform(a)
print(b)
输出:
[[0 1 0 0]
[1 0 0 0]
[0 0 0 1]]
在 Python 中使用 pandas
模块的 NumPy 数组上执行一位有效编码
机器学习算法的数据集通常采用 pandas
DataFrame 的形式。因此,pandas
模块可以很好地执行数据编码。get_dummies()
可用于将分类数据集转换为数字指示符,从而执行一位有效编码。最终结果是一个 DataFrame。
例如,
import pandas as pd
import numpy as np
a = np.array([1, 0, 3])
b = pd.get_dummies(a)
print(b)
输出:
0 1 3
0 0 1 0
1 1 0 0
2 0 0 1
在 Python 中使用 keras
模块的 NumPy 数组上执行一位有效编码
keras
模块被广泛用于 Python 的机器学习。该模块中的 to_categorical()
函数可以对数据执行一位有效编码。
下面的代码段显示了如何。
from keras.utils.np_utils import to_categorical
import numpy as np
a = np.array([1, 0, 3])
b = to_categorical(a, num_classes=(len(a) + 1))
print(b)
输出:
[[0. 1. 0. 0.]
[1. 0. 0. 0.]
[0. 0. 0. 1.]]
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn