PHP 中已弃用的 Mysql_connect 的解决方案
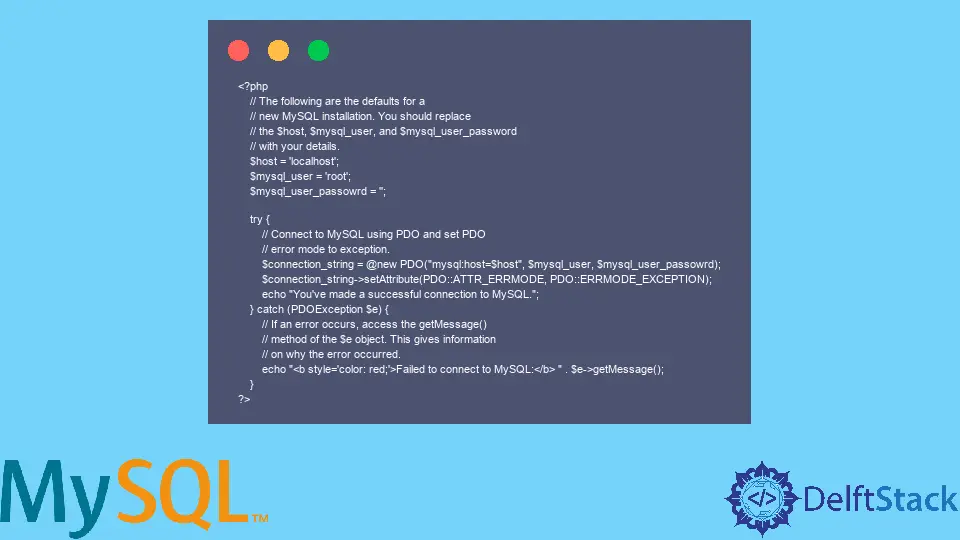
本文将教你解决 PHP 中已弃用的 mysql_connect
的解决方案。这些解决方案包括 mysqli_connect
(过程和 OOP)和 PHP 数据对象 (PDO)。
使用程序 Mysqli_connect
连接到 MySQL
mysqli_connect
允许你使用过程编程连接到你的 MySQL 数据库。因此,你可以使用以下算法:
-
定义数据库连接详细信息。
-
使用
mysqli_connect
连接。 -
如果连接成功,则显示成功消息。
-
如果连接失败,则显示错误消息。
在步骤 2 中发生错误之前,此算法工作正常。此类错误可能是不正确的数据库详细信息。
当 PHP 遇到这些不正确的细节时,它会抛出一个 Fatal Error
异常。因此,代码不会进入第 3 步或第 4 步,你将在其中显示错误消息。
为了解决这个问题,我们必须关闭 MySQL 错误报告并禁止警告。通过这样做,当发生错误时,你将阻止用户看到敏感细节,同时,你可以显示自定义错误消息。
话虽如此,以下是修改后的算法:
-
定义数据库连接详细信息。
-
关闭 MySQL 错误报告。
-
使用
mysqli_connect
连接并抑制警告。 -
如果连接成功,则显示成功消息。
-
如果连接失败,则显示错误消息。
下面是这个算法的实现:
<?php
// The following are the defaults for a
// new MySQL installation. You should replace
// the $host, $mysql_user, and $mysql_user_password
// with your details.
$host = 'localhost';
$mysql_user = 'root';
$mysql_user_passowrd = '';
// Turn off error reports like "Fatal Errors".
// Such reports can contain too much sensitive
// information that no one should see. Also,
// turning off error reports allows us to handle
// connection error in an if/else statement.
mysqli_report(MYSQLI_REPORT_OFF);
// If there is an error in the connection string,
// PHP will produce a Warning message. For security
// and private reasons, it's best to suppress the
// warnings using the '@' sign
$connect_to_mysql = @mysqli_connect($host, $mysql_user, $mysql_user_passowrd);
if (!$connect_to_mysql) {
echo "<b style='color: red;'>Failed to connect to MySQL.</b>";
} else {
echo "You've made a successful connection to MySQL.";
}
?>
成功连接的输出:
You've made a successful connection to MySQL.
连接失败的输出:
<b style='color: red;'>Failed to connect to MySQL.</b>
使用面向对象编程连接到 MySQL
使用 OOP 和 mysqli_connect
,你可以使用 new mysqli()
创建数据库连接。与程序技术一样,你必须将连接详细信息传递给 new mysqli()
。
但是,这些细节中可能存在错误,因此我们将使用 try...catch
块进行错误处理。首先,我们将连接详细信息放在 try
块中,如果发生错误,我们将在 catch
块中捕获它们。
在下文中,与 MySQL 的连接使用 OOP 版本的 mysqli_connect
。你还会注意到我们已经抑制了连接字符串中的错误。
<?php
// The following are the defaults for a
// new MySQL installation. You should replace
// the $host, $mysql_user, and $mysql_user_password
// with your details.
$host = 'localhost';
$mysql_user = 'root';
$mysql_user_passowrd = '';
try {
// Connect to the database using the OOP style
// of mysqli.
$connection_string = @new mysqli($host, $mysql_user, $mysql_user_passowrd);
echo "You've made a successful connection to MySQL.";
} catch (Exception $e) {
// If an error occurs, access the getMessage()
// method of the $e object. This gives information
// on why the error occurred.
echo "<b style='color: red;'>Failed to connect to MySQL :</b> " . $e->getMessage();
}
?>
成功连接的输出:
You've made a successful connection to MySQL.
连接失败的输出:
<b style='color: red;'>Failed to connect to MySQL :</b> Access denied for user ''@'localhost' (using password: NO)
使用 PDO 连接到 MySQL
你可以使用 PDO 连接到 MySQL,并且你应该使用 try...catch
块捕获错误。后者的工作方式与你在 OOP 部分中学习的方式相同。
此外,当错误发生时,你的用户不会看到敏感的错误消息。现在,下面是连接 MySQL 的 PDO 版本:
<?php
// The following are the defaults for a
// new MySQL installation. You should replace
// the $host, $mysql_user, and $mysql_user_password
// with your details.
$host = 'localhost';
$mysql_user = 'root';
$mysql_user_passowrd = '';
try {
// Connect to MySQL using PDO and set PDO
// error mode to exception.
$connection_string = @new PDO("mysql:host=$host", $mysql_user, $mysql_user_passowrd);
$connection_string->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "You've made a successful connection to MySQL.";
} catch (PDOException $e) {
// If an error occurs, access the getMessage()
// method of the $e object. This gives information
// on why the error occurred.
echo "<b style='color: red;'>Failed to connect to MySQL:</b> " . $e->getMessage();
}
?>
连接成功的输出:
You've made a successful connection to MySQL.
连接失败的输出:
Failed to connect to MySQL: SQLSTATE[HY000] [1045] Access denied for user 'root'@'localhost' (using password: YES)
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn