PHP에서 사용되지 않는 Mysql_connect에 대한 솔루션
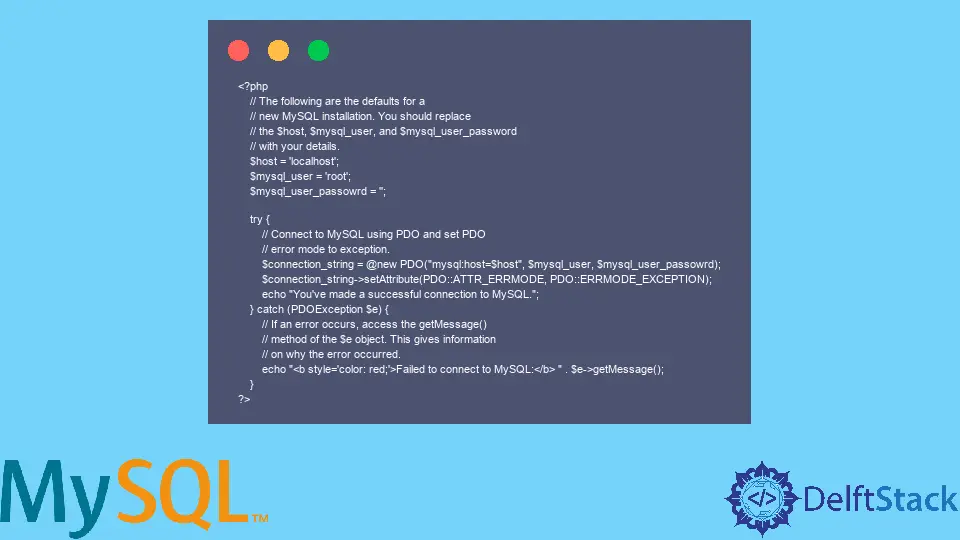
이 기사는 PHP에서 더 이상 사용되지 않는 mysql_connect
에 대한 솔루션을 알려줍니다. 이러한 솔루션에는 mysqli_connect
(절차 및 OOP) 및 PHP 데이터 개체(PDO)가 포함됩니다.
절차 Mysqli_connect
를 사용하여 MySQL에 연결
‘mysqli_connect’를 사용하면 절차적 프로그래밍을 사용하여 MySQL 데이터베이스에 연결할 수 있습니다. 결과적으로 다음 알고리즘을 사용할 수 있습니다.
-
데이터베이스 연결 세부 정보를 정의합니다.
-
mysqli_connect
로 연결하십시오. -
연결에 성공하면 성공 메시지를 표시합니다.
-
연결에 실패한 경우 오류 메시지를 표시합니다.
이 알고리즘은 2단계에서 오류가 발생할 때까지 제대로 작동합니다. 이러한 오류는 잘못된 데이터베이스 세부 정보일 수 있습니다.
PHP가 이러한 잘못된 세부 정보를 발견하면 치명적인 오류
예외가 발생합니다. 따라서 코드는 오류 메시지가 표시되는 3단계 또는 4단계로 이동하지 않습니다.
이 문제를 해결하려면 MySQL 오류 보고를 끄고 경고를 표시하지 않아야 합니다. 이렇게 하면 오류가 발생했을 때 사용자가 민감한 세부 정보를 볼 수 없도록 하는 동시에 사용자 지정 오류 메시지를 표시할 수 있습니다.
즉, 다음은 수정된 알고리즘입니다.
-
데이터베이스 연결 세부 정보를 정의합니다.
-
MySQL 오류 보고를 끕니다.
-
mysqli_connect
로 연결하고 경고를 표시하지 마십시오. -
연결에 성공하면 성공 메시지를 표시합니다.
-
연결에 실패한 경우 오류 메시지를 표시합니다.
다음은 이 알고리즘의 구현입니다.
<?php
// The following are the defaults for a
// new MySQL installation. You should replace
// the $host, $mysql_user, and $mysql_user_password
// with your details.
$host = 'localhost';
$mysql_user = 'root';
$mysql_user_passowrd = '';
// Turn off error reports like "Fatal Errors".
// Such reports can contain too much sensitive
// information that no one should see. Also,
// turning off error reports allows us to handle
// connection error in an if/else statement.
mysqli_report(MYSQLI_REPORT_OFF);
// If there is an error in the connection string,
// PHP will produce a Warning message. For security
// and private reasons, it's best to suppress the
// warnings using the '@' sign
$connect_to_mysql = @mysqli_connect($host, $mysql_user, $mysql_user_passowrd);
if (!$connect_to_mysql) {
echo "<b style='color: red;'>Failed to connect to MySQL.</b>";
} else {
echo "You've made a successful connection to MySQL.";
}
?>
성공적인 연결에 대한 출력:
You've made a successful connection to MySQL.
실패한 연결에 대한 출력:
<b style='color: red;'>Failed to connect to MySQL.</b>
객체 지향 프로그래밍을 사용하여 MySQL에 연결
OOP와 mysqli_connect
를 사용하면 new mysqli()
를 사용하여 데이터베이스 연결을 생성할 수 있습니다. 절차적 기술과 마찬가지로 연결 세부 정보를 new mysqli()
에 전달해야 합니다.
그러나 이러한 세부 정보에 오류가 있을 수 있으므로 오류 처리를 위해 try...catch
블록을 사용합니다. 먼저 try
블록에 연결 세부 정보를 배치하고 오류가 발생하면 catch
블록에서 이를 포착합니다.
다음에서 MySQL에 대한 연결은 mysqli_connect
의 OOP 버전을 사용합니다. 또한 연결 문자열에서 오류가 억제되었음을 알 수 있습니다.
<?php
// The following are the defaults for a
// new MySQL installation. You should replace
// the $host, $mysql_user, and $mysql_user_password
// with your details.
$host = 'localhost';
$mysql_user = 'root';
$mysql_user_passowrd = '';
try {
// Connect to the database using the OOP style
// of mysqli.
$connection_string = @new mysqli($host, $mysql_user, $mysql_user_passowrd);
echo "You've made a successful connection to MySQL.";
} catch (Exception $e) {
// If an error occurs, access the getMessage()
// method of the $e object. This gives information
// on why the error occurred.
echo "<b style='color: red;'>Failed to connect to MySQL :</b> " . $e->getMessage();
}
?>
성공적인 연결에 대한 출력:
You've made a successful connection to MySQL.
실패한 연결에 대한 출력:
<b style='color: red;'>Failed to connect to MySQL :</b> Access denied for user ''@'localhost' (using password: NO)
PDO를 사용하여 MySQL에 연결
PDO를 사용하여 MySQL에 연결할 수 있으며 try... catch
블록을 사용하여 오류를 잡아야 합니다. 후자는 OOP 섹션에서 배운 것과 동일한 방식으로 작동합니다.
또한 사용자는 오류가 발생할 때 민감한 오류 메시지를 볼 수 없습니다. 이제 다음은 MySQL에 연결하기 위한 PDO 버전입니다.
<?php
// The following are the defaults for a
// new MySQL installation. You should replace
// the $host, $mysql_user, and $mysql_user_password
// with your details.
$host = 'localhost';
$mysql_user = 'root';
$mysql_user_passowrd = '';
try {
// Connect to MySQL using PDO and set PDO
// error mode to exception.
$connection_string = @new PDO("mysql:host=$host", $mysql_user, $mysql_user_passowrd);
$connection_string->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "You've made a successful connection to MySQL.";
} catch (PDOException $e) {
// If an error occurs, access the getMessage()
// method of the $e object. This gives information
// on why the error occurred.
echo "<b style='color: red;'>Failed to connect to MySQL:</b> " . $e->getMessage();
}
?>
성공적인 연결에 대한 출력:
You've made a successful connection to MySQL.
실패한 연결에 대한 출력:
Failed to connect to MySQL: SQLSTATE[HY000] [1045] Access denied for user 'root'@'localhost' (using password: YES)
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn