在 JavaScript 中过滤数组多个值
-
JavaScript 中使用
filter()
方法通过检查多个值来过滤数组 -
JavaScript 中使用
filter()
方法通过检查多个值来过滤对象数组 -
在 JavaScript 中使用
filter()
方法动态过滤对象数组
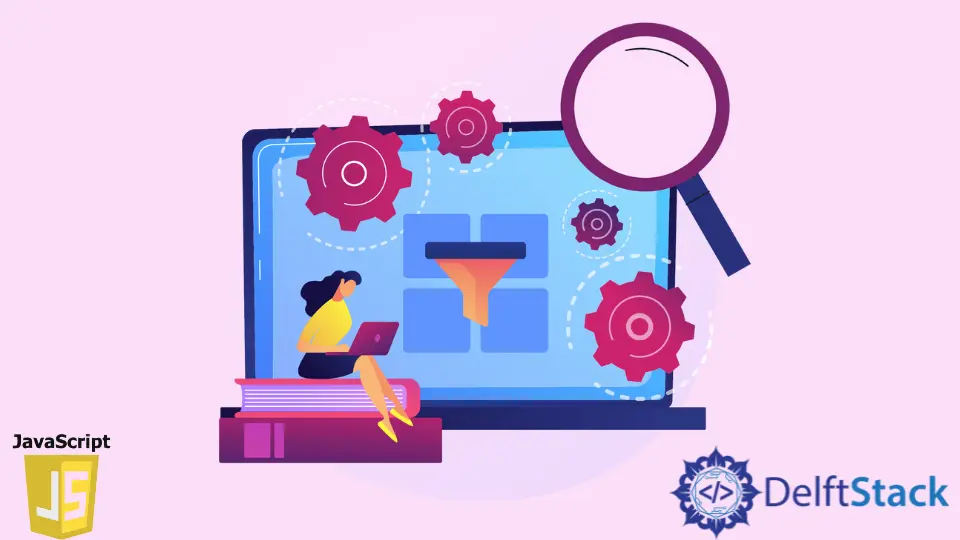
filter()
方法使用已通过测试函数的元素创建一个全新的数组。
JavaScript 中使用 filter()
方法通过检查多个值来过滤数组
例如,我们将检索姓氏以 M
开头并且至少参加了 3 门课程的学生的记录。
filter()
函数不会修改/更新原始数组并针对空元素运行。
var array = [1, 3, 4, 2, 6, 7, 5, 9, 10, 8];
var result = array.filter(array => array < 10 && array >= 2);
console.log(result);
输出:
[3, 4, 2, 6, 7, 5, 9, 8]
在这里,我们在 filter()
方法中使用箭头函数。
请记住,我们还可以传递函数名称并单独编写该函数。对数组中的每个元素执行此数组函数,并检查是否满足指定的条件。
然后该元素将保存在 result
数组中,否则,移动到数组的下一个元素。
JavaScript 中使用 filter()
方法通过检查多个值来过滤对象数组
在这里,我们有一个包含 4 个对象的 cities
数组。
每个对象都有两个属性,city_name
和 city_population
。
let cities = [
{city_name: 'Los Angeles', city_population: 3992631},
{city_name: 'New York', city_population: 8185433},
{city_name: 'Chicago', city_population: 2655568},
{city_name: 'China', city_population: 2039471},
];
let bigCitiesAndPopulation = cities.filter(function(e) {
return e.city_population > 2000000 && e.city_name.startsWith('Chi');
});
console.log(bigCitiesAndPopulation);
输出:
[{
city_name: "Chicago",
city_population: 2655568
}, {
city_name: "China",
city_population: 2039471
}]
filter()
方法返回数组,因为我们将它与数组一起使用。现在,我们将它与对象数组一起使用,filter()
函数返回一个对象数组。
或者,我们可以使用 filter()
方法来获得准确的结果。
let bigCitiesAndPopulation = cities.filter(
city => city.city_population > 2000000 && city.city_name.startsWith('Chi'));
console.log(bigCitiesAndPopulation);
输出:
[{
city_name: "Chicago",
city_population: 2655568
}, {
city_name: "China",
city_population: 2039471
}]
不使用内置方法的过滤器方法的实现。
let bigCitiesAndPopulation = [];
for (let i = 0; i < cities.length; i++) {
if (cities[i].city_population > 2000000 &&
cities[i].city_name.startsWith('Chi')) {
bigCitiesAndPopulation.push(cities[i]);
}
}
console.log(bigCitiesAndPopulation);
输出:
[{
city_name: "Chicago",
city_population: 2655568
}, {
city_name: "China",
city_population: 2039471
}]
我们使用一个一直运行到 cities.length-1
的 for
循环。在 for-loop
中,我们检查 city_population
是否大于 2000000
并且 city_name
是否以 Chi
开头。
如果两个条件都满足,那么只有这个城市才会被推入数组 bigCitiesAndPopulation
。
在上面的示例中,我们应该只使用两个值来过滤对象数组。
假设我们有许多值将被检查以过滤对象数组。在这种情况下,我们必须将这些值保存在单独的数组中。
例如,我们在 filtersArray
中有 4 个值 Biology
、Physics
、Chemistry
和 Arts
。我们的目标是获取那些具有 filtersArray
的对象。
var studentCourses = [
{id: 210, courses: 'Biology Physics Math'},
{id: 211, courses: 'History Physics ComputerScience'},
{id: 212, courses: 'Arts Language Biology Chemistry Physics'},
{id: 213, courses: 'Chemistry Statistics Math'},
{id: 214, courses: 'Biology Chemistry Physics Arts'},
];
var filtersArray = ['Biology', 'Physics', 'Chemistry', 'Arts'];
var filteredArray = studentCourses.filter(function(element) {
var courses = element.courses.split(' ');
return courses
.filter(function(course) {
return filtersArray.indexOf(course) > -1;
})
.length === filtersArray.length;
});
console.log(filteredArray);
输出:
[{
courses: "Arts Language Biology Chemistry Physics",
id: 212
}, {
courses: "Biology Chemistry Physics Arts",
id: 214
}]
我们得到那些包含所有 filtersArray
元素的对象。请记住,对象可以有额外的课程,但它们必须包含 filterArray
的元素才能被过滤。
在 JavaScript 中使用 filter()
方法动态过滤对象数组
动态意味着一切都将在运行时决定。
'use strict';
Array.prototype.flexFilter =
function(criteria) {
// set variables
var matchFilters, matches = [], counter;
// helper function to iterate over the criteria (filter criteria)
matchFilters =
function(item) {
counter = 0
for (var n = 0; n < criteria.length; n++) {
if (criteria[n]['Values'].indexOf(item[criteria[n]['Field']]) > -1) {
counter++;
}
}
// The array's current items satisfies all the filter criteria, if it is
// true
return counter == criteria.length;
}
// loop through every item of the array
// and checks if the item satisfies the filter criteria
for (var i = 0; i < this.length; i++) {
if (matchFilters(this[i])) {
matches.push(this[i]);
}
}
// returns a new array holding the objects that fulfill the filter criteria
return matches;
}
var personData = [
{id: 1, name: 'John', month: 'January', gender: 'M'},
{id: 2, name: 'Thomas', month: 'March', gender: 'M'},
{id: 3, name: 'Saira', month: 'April', gender: 'F'},
{id: 4, name: 'Daniel', month: 'November', gender: 'M'},
{id: 5, name: 'Leonardo', month: 'March', gender: 'M'},
{id: 6, name: 'Jamaima', month: 'April', gender: 'F'},
{id: 7, name: 'Tina', month: 'December', gender: 'F'},
{id: 8, name: 'Mehvish', month: 'March', gender: 'F'}
];
var filter_criteria = [
{Field: 'month', Values: ['March']}, {Field: 'gender', Values: ['F', 'M']}
];
var filtered = personData.flexFilter(filter_criteria);
console.log(filtered);
我们使用一个函数来遍历 filter_criteria
来检索匹配的值。
每个过滤条件都将被视为 AND
,而多个过滤器值在过滤字段中被视为 OR
。
输出:
[{
gender: "M",
id: 2,
month: "March",
name: "Thomas"
}, {
gender: "M",
id: 5,
month: "March",
name: "Leonardo"
}, {
gender: "F",
id: 8,
month: "March",
name: "Mehvish"
}]
检查 filter_criteria
后,我们遍历每个数组元素并评估它是否满足过滤条件。
我们 push
进入 matches
数组以满足过滤条件。否则不行。
循环结束后,我们打印满足 filter_criteria
的新数组。