在 Java 中计算对数
Sheeraz Gul
2023年10月12日
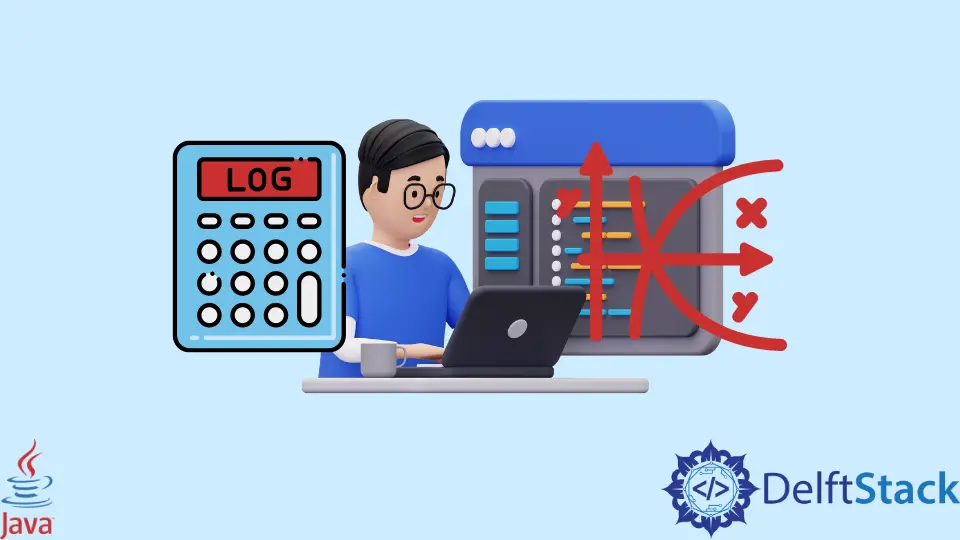
本教程将演示如何使用 Math.log
函数在 Java 中计算对数。
在 Java 中使用 Math.log
计算给定值的对数
在 Java 中,java.lang.Math
库具有函数 Math.log()
来计算给定值的对数。输入值可以是双精度、整数或浮点数,并返回双精度值。
我们必须确保数字不是负数、零或无穷大; 否则,输出将不是双精度数据类型。
下面的例子展示了 Java 中 Math.log
的使用。
import java.lang.Math;
class Java_Log {
public static void main(String args[]) {
double p = -4.3;
double q = 6.0 / 0;
double r = 0;
double s = 130.333;
double u = 130.333 / 30;
int v = 5;
float w = 34;
// The negative double in the Math.log function will output: NaN
System.out.println("The Output for Negative Integer:");
System.out.println(Math.log(p));
// The positive infinity in the Math.log function will output: Infinity
System.out.println("The Output for Positive Infinity:");
System.out.println(Math.log(q));
// The positive zero in the Math.log function will output: - Infinity
System.out.println("The Output for Zero:");
System.out.println(Math.log(r));
// The positive double argument in the Math.log function will output: logarithm answer
System.out.println("The Output for positive double:");
System.out.println(Math.log(s));
// The positive double argument in the Math.log function will output: logarithm answer
System.out.println("The Output for Positive double in division form:");
System.out.println(Math.log(u));
// The positive integer argument in the Math.log function will output: logarithm answer
System.out.println("The Output for Positive Integer:");
System.out.println(Math.log(v));
// The positive integer float argument in the Math.log function will output: logarithm answer
System.out.println("The Output for Positive float:");
System.out.println(Math.log(w));
}
}
输出:
The Output for Negative Integer:
NaN
The Output for Positive Infinity:
Infinity
The Output for Zero:
-Infinity
The Output for positive double:
4.870092713769228
The Output for Positive double in division form:
1.468895332107073
The Output for Positive Integer:
1.6094379124341003
The Output for Positive float:
3.5263605246161616
上面的代码计算每种数据类型的对数,并返回双精度、整数、浮点数、无穷大和零输入值的输出值。
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook