如何在 Java 中检查字符串是否为空
Hassan Saeed
2023年10月12日
Java
Java String
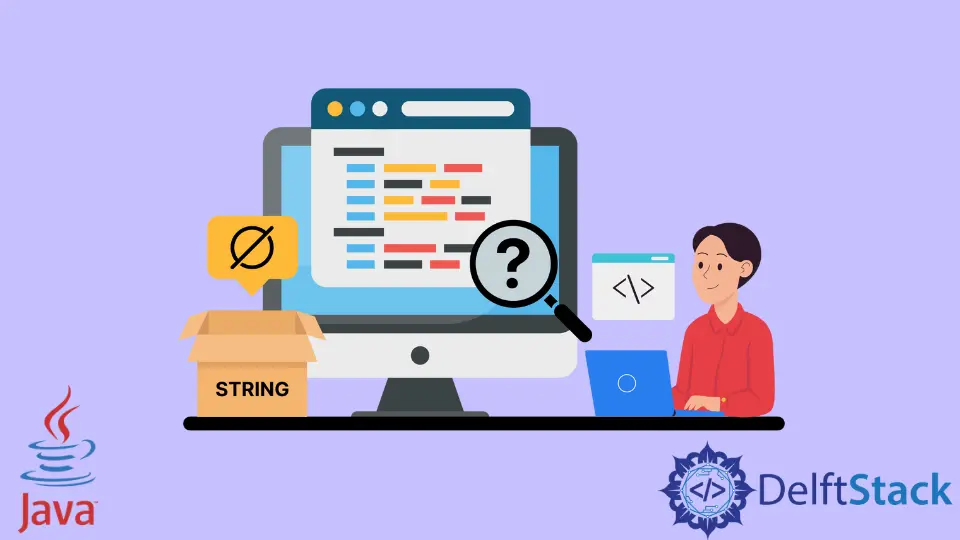
本教程讨论了在 Java 中检查一个字符串是否为空的方法。
在 Java 中使用 str == null
检查一个字符串是否为 null
在 Java 中检查一个给定的字符串是否为 null
的最简单方法是使用 str == null
将其与 null
进行比较。下面的例子说明了这一点。
public class MyClass {
public static void main(String args[]) {
String str1 = null;
String str2 = "Some text";
if (str1 == null)
System.out.println("str1 is a null string");
else
System.out.println("str1 is not a null string");
if (str2 == null)
System.out.println("str2 is a null string");
else
System.out.println("str2 is not a null string");
}
}
输出:
str1 is a null string
str2 is not a null string
在 Java 中使用 str.isEmpty()
检查一个字符串是否为空
在 Java 中检查一个给定的字符串是否为空的最简单方法是使用 String
类的内置方法- isEmpty()
。下面的例子说明了这一点。
public class MyClass {
public static void main(String args[]) {
String str1 = "";
String str2 = "Some text";
if (str1.isEmpty())
System.out.println("str1 is an empty string");
else
System.out.println("str1 is not an empty string");
if (str2.isEmpty())
System.out.println("str2 is an empty string");
else
System.out.println("str2 is not an empty string");
}
}
输出:
str1 is an empty string
str2 is not an empty string
如果我们有兴趣同时检查这两个条件,我们可以通过使用逻辑或运算符-||
来实现。下面的例子说明了这一点。
public class MyClass {
public static void main(String args[]) {
String str1 = "";
if (str1.isEmpty() || str1 == null)
System.out.println("This is an empty or null string");
else
System.out.println("This is neither empty nor null string");
}
}
输出:
This is an empty or null string
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe