在 Java 中将 Stream 转换为列表
Mohammad Irfan
2023年10月12日
Java
Java Stream
Java List
-
在 Java 中使用
collect()
方法将流转换为列表 -
在 Java 中使用
toCollection()
方法将流转换为列表 -
在 Java 中使用
forEach()
方法将流转换为列表 -
在 Java 中使用
toArray()
方法将流转换为列表
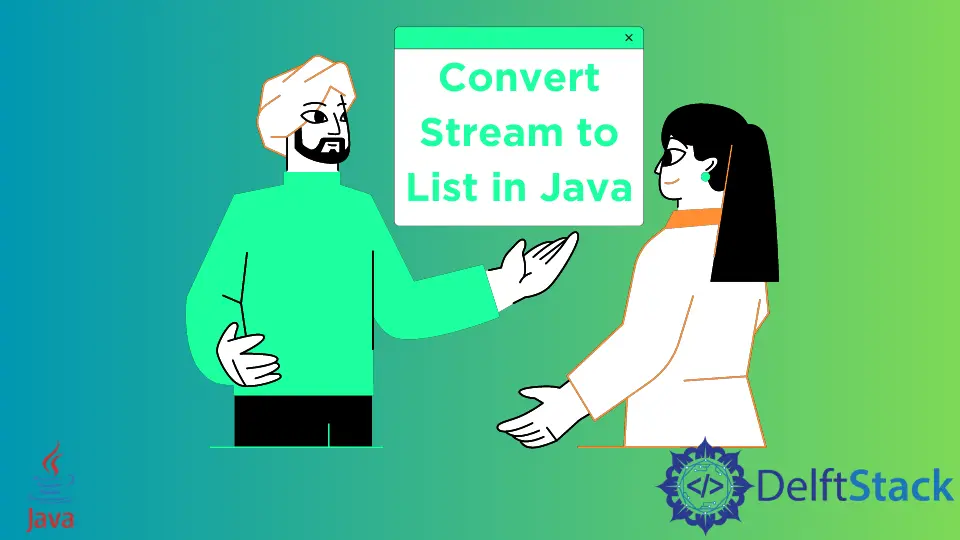
本教程介绍了 Java 中 Stream
到 List 的转换。
Stream 是对象的集合。Stream 不存储任何数据,因此它不是数据结构。
Stream
被添加到 Java 8 版本中,而 List 是一个存储有序集合的接口。在本教程中,我们将研究将 Stream 转换为 List。
Java 有以下方法可以用来完成我们的任务:
- 使用
collect()
方法进行转换 - 使用
toCollection()
方法进行转换 - 使用
forEach()
方法进行转换 - 使用
toArray()
方法进行转换
在 Java 中使用 collect()
方法将流转换为列表
Stream collect()
操作是一个终端操作。终端操作意味着 Stream 已被消费并且在此操作之后无法进一步使用。
这个方法的语法是:
collect(Collector<? super T, A, R> collector)
此方法将 Collector
对象作为参数。
Collector 类用于将 Stream 的元素收集到一个集合中。此类具有 toList()
方法,可将 Stream 转换为 List。
现在让我们看一个代码示例:
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class SimpleTesting {
public static void main(String args[]) {
// declaring a stream
Stream<String> furnitures =
Stream.of("chair", "dinning table", "study table", "coffee table", "sofa");
// changing stream to list
List<String> furniture_list = furnitures.collect(Collectors.toList());
// printing the list
for (String furniture : furniture_list) {
System.out.println(furniture);
}
}
}
输出:
chair
dinning table
study table
coffee table
sofa
在 Java 中使用 toCollection()
方法将流转换为列表
此示例与上一个示例类似,只是我们使用了 Collector.toList()
方法而不是 Collectors.toCollection()
方法。
Collector
类有一个名为 toCollection()
的方法,该方法返回一个 Collector
,它将输入项按照遇到的顺序收集到一个新的 Collection 中。看下面的示例代码:
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class SimpleTesting {
public static void main(String args[]) {
// declaring a stream
Stream<String> furnitures =
Stream.of("chair", "dinning table", "study table", "coffee table", "sofa");
// changing stream to list
List<String> furniture_list = furnitures.collect(Collectors.toCollection(ArrayList::new));
// printing the list
for (String furniture : furniture_list) {
System.out.println(furniture);
}
}
}
输出:
chair
dinning table
study table
coffee table
sofa
在 Java 中使用 forEach()
方法将流转换为列表
在这个例子中,我们首先创建了一个空的 ArrayList,然后使用 forEach()
方法将每个 Stream 元素一个一个添加到 List 中。Stream
有一个名为 forEach()
的方法,它对输入 Stream 的所有元素执行。
看下面的示例代码:
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class SimpleTesting {
public static void main(String args[]) {
// declaring a stream
Stream<String> furnitures =
Stream.of("chair", "dinning table", "study table", "coffee table", "sofa");
// declaring an empty arraylist
ArrayList<String> furniture_list = new ArrayList<>();
// adding elements one by one
furnitures.forEach(furniture_list::add);
// printing the list
for (String furniture : furniture_list) {
System.out.println(furniture);
}
}
}
输出:
chair
dinning table
study table
coffee table
sofa
在 Java 中使用 toArray()
方法将流转换为列表
在这个例子中,我们首先使用 toArray()
方法将 Stream
转换为一个数组。之后,我们使用 asList()
方法将新创建的数组转换为 List 以获取列表。
看下面的代码:
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class SimpleTesting {
public static void main(String args[]) {
// declaring a stream
Stream<String> furnitures =
Stream.of("chair", "dinning table", "study table", "coffee table", "sofa");
// converting stream to array
String[] furniture_array = furnitures.toArray(String[] ::new);
// converting array to list
List<String> furniture_list = Arrays.asList(furniture_array);
// printing the list
for (String furniture : furniture_list) {
System.out.println(furniture);
}
}
}
输出:
chair
dinning table
study table
coffee table
sofa
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
相关文章 - Java Stream
- Java 中的 Stream 的 reduce 操作
- Java 中的流过滤器
- Java 中的 findFirst 流方法
- 在 Java 中将 Stream 元素转换为映射
- Java 中的 flatMap
- 在 Java 中把数组转换为流