在 C# 中将换行符添加到字符串
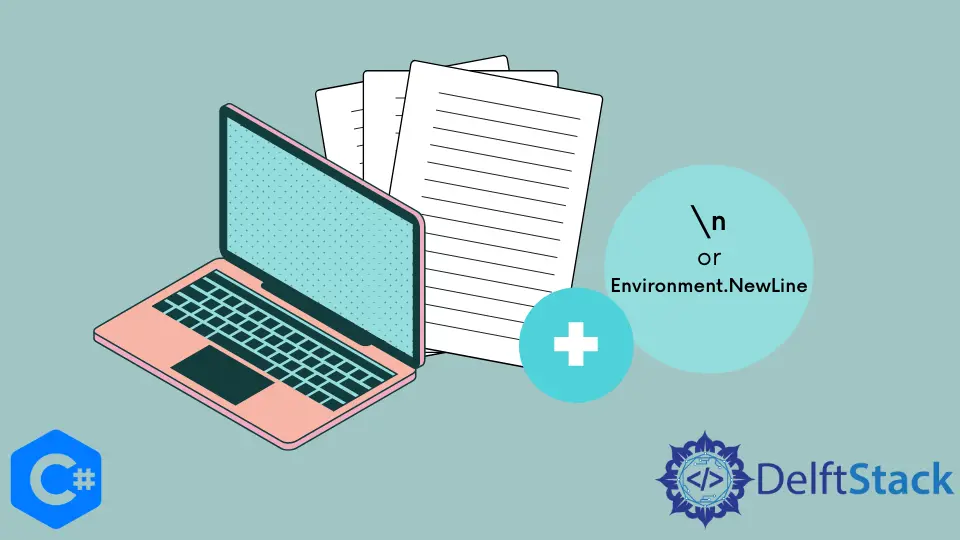
本教程将介绍在 C# 中向字符串变量添加新行的方法。
C# 中使用\n
转义字符将新行添加到字符串中
Mac 中的\n
或\r
转义符用于在 C# 中向控制台添加新行。由于我使用的是 Windows 计算机,因此在本教程中将使用\n
转义符来换行。换行符还可以用于向字符串变量添加多行。无论何时要开始新行,都必须在字符串中写入\n
。以下代码示例向我们展示了如何在 C# 中使用\n
转义字符向字符串变量添加新行。
using System;
namespace add_newline_to_string {
class Program {
static void Main(string[] args) {
string s = "This is the first line.\nThis is the second line.";
Console.WriteLine(s);
}
}
}
输出:
This is the first line.
This is the second line.
我们用 C# 中的\n
转义字符在字符串变量 s
中添加了新行。该方法的唯一缺点是我们必须在字符串变量 s
的初始化期间编写\n
。上面的代码可以用 String.Replace()
函数来修改,在字符串变量 s
初始化后增加一个换行符。String.Replace(string x, y)
返回一个字符串,其中将字符串 x
替换为字符串 y
。下面的代码示例向我们展示了如何在 C# 中使用 String.Replace()
函数初始化后添加\n
。
using System;
namespace add_newline_to_string {
class Program {
static void Main(string[] args) {
string s = "This is the first line.This is the second line.";
s = s.Replace(".", ".\n");
Console.WriteLine(s);
}
}
}
输出:
This is the first line.
This is the second line.
在 C# 中使用 String.Replace()
函数初始化后,我们在字符串变量 s
中添加了新行。这种向字符串添加新行的方法不是最佳方法,因为\n
转义字符取决于环境。我们需要知道我们的代码运行的环境,以便通过这种方法将新行正确地添加到字符串变量中。
使用 C# 中的 Environment.NewLine
属性将新行添加到字符串中
如果我们想在代码中添加新行,但是对代码将在其中运行的环境一无所知,则可以使用 C# 中的 Environment.NewLine
属性。Environment.NewLine
属性获取适合我们环境的换行符。以下代码示例向我们展示了如何使用 C# 中的 Environment.NewLine
属性向字符串添加新行。
using System;
namespace add_newline_to_string {
class Program {
static void Main(string[] args) {
string s = "This is the first line.This is the second line.";
s = s.Replace(".", "." + Environment.NewLine);
Console.WriteLine(s);
}
}
}
输出:
This is the first line.
This is the second line.
我们初始化了一个字符串变量 s
,并在使用 C# 中的 Environment.NewLine
属性和 String.Replace()
函数初始化后,在字符串变量 s
中添加了新行。此方法优于其他方法,因为在此方法中,我们不必担心代码将在其中执行的环境。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn