在 C# 中將換行符新增到字串
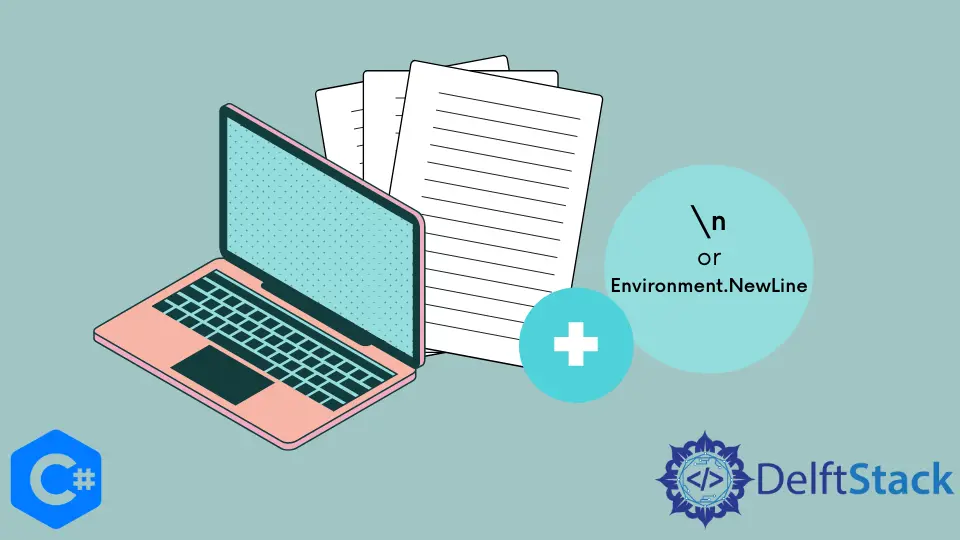
本教程將介紹在 C# 中向字串變數新增新行的方法。
C# 中使用\n
轉義字元將新行新增到字串中
Mac 中的\n
或\r
轉義符用於在 C# 中向控制檯新增新行。由於我使用的是 Windows 計算機,因此在本教程中將使用\n
轉義符來換行。換行符還可以用於向字串變數新增多行。無論何時要開始新行,都必須在字串中寫入\n
。以下程式碼示例向我們展示瞭如何在 C# 中使用\n
轉義字元向字串變數新增新行。
using System;
namespace add_newline_to_string {
class Program {
static void Main(string[] args) {
string s = "This is the first line.\nThis is the second line.";
Console.WriteLine(s);
}
}
}
輸出:
This is the first line.
This is the second line.
我們用 C# 中的\n
轉義字元在字串變數 s
中新增了新行。該方法的唯一缺點是我們必須在字串變數 s
的初始化期間編寫\n
。上面的程式碼可以用 String.Replace()
函式來修改,在字串變數 s
初始化後增加一個換行符。String.Replace(string x, y)
返回一個字串,其中將字串 x
替換為字串 y
。下面的程式碼示例向我們展示瞭如何在 C# 中使用 String.Replace()
函式初始化後新增\n
。
using System;
namespace add_newline_to_string {
class Program {
static void Main(string[] args) {
string s = "This is the first line.This is the second line.";
s = s.Replace(".", ".\n");
Console.WriteLine(s);
}
}
}
輸出:
This is the first line.
This is the second line.
在 C# 中使用 String.Replace()
函式初始化後,我們在字串變數 s
中新增了新行。這種向字串新增新行的方法不是最佳方法,因為\n
轉義字元取決於環境。我們需要知道我們的程式碼執行的環境,以便通過這種方法將新行正確地新增到字串變數中。
使用 C# 中的 Environment.NewLine
屬性將新行新增到字串中
如果我們想在程式碼中新增新行,但是對程式碼將在其中執行的環境一無所知,則可以使用 C# 中的 Environment.NewLine
屬性。Environment.NewLine
屬性獲取適合我們環境的換行符。以下程式碼示例向我們展示瞭如何使用 C# 中的 Environment.NewLine
屬性向字串新增新行。
using System;
namespace add_newline_to_string {
class Program {
static void Main(string[] args) {
string s = "This is the first line.This is the second line.";
s = s.Replace(".", "." + Environment.NewLine);
Console.WriteLine(s);
}
}
}
輸出:
This is the first line.
This is the second line.
我們初始化了一個字串變數 s
,並在使用 C# 中的 Environment.NewLine
屬性和 String.Replace()
函式初始化後,在字串變數 s
中新增了新行。此方法優於其他方法,因為在此方法中,我們不必擔心程式碼將在其中執行的環境。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn