在 C# 中发出 HTTP POST Web 请求
-
使用 C# 中的
WebClient
类发出 HTTP POST Web 请求 -
使用 C# 中的
HttpWebRequest
类发出 HTTP POST Web 请求 -
使用 C# 中的
HttpClient
类发出 HTTP POST Web 请求
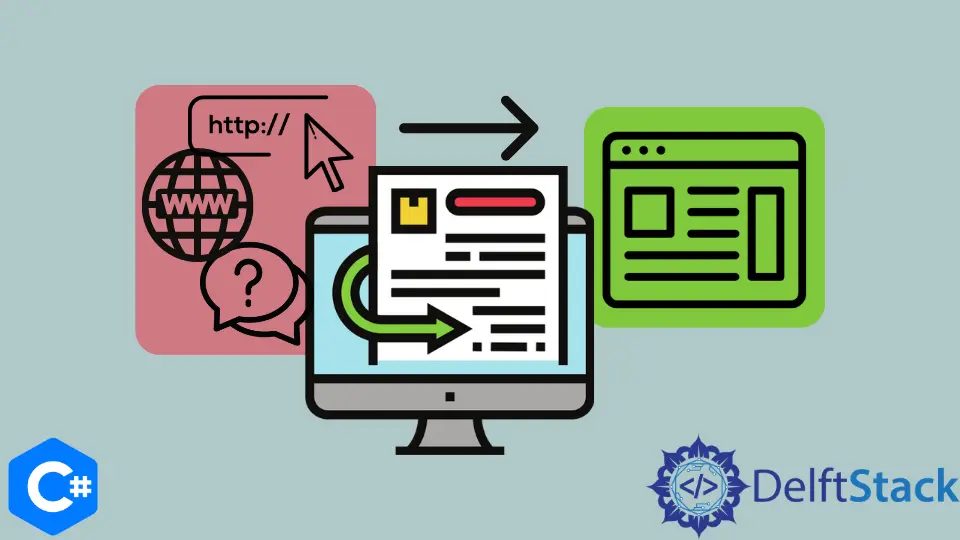
本教程将讨论在 C# 中发出 HTTP POST Web 请求的方法。
使用 C# 中的 WebClient
类发出 HTTP POST Web 请求
WebClient
类在 C# 中提供了许多向 URL 发送数据和从 URL 接收数据的方法。我们可以在 C# 中使用 WebClient.UploadValues(url, values)
函数的 WebClient
类来进行 HTTP POST 请求。以下代码示例向我们展示了如何使用 C# 中的 WebClient
类发出简单的 HTTP POST Web 请求。
using System.Net;
using System.Collections.Specialized;
var wb = new WebClient() var data = new NameValueCollection();
string url = "www.example.com" data["username"] = "myUser";
data["password"] = "myPassword";
var response = wb.UploadValues(url, "POST", data);
在上面的代码中,我们创建了 Web 客户端 wb
,用于将数据发送到 url
。我们初始化要发送到 URL
的 data
变量。通过在 wb.UploadValues(url, "POST", data)
函数的参数中指定 POST
,我们对 url
发出 HTTP POST Web 请求。url
的响应保存在 reponse
变量中。
使用 C# 中的 HttpWebRequest
类发出 HTTP POST Web 请求
HttpWebRequest 类提供了在 C# 中使用 HTTP 协议与服务器直接交互的方法。我们可以使用 HttpWebRequest.Method = "POST"
属性来指定 HTTP Web 请求是 C# 中的 POST 请求。以下代码示例向我们展示了如何使用 C# 中的 HttpWebRequest
类发出简单的 HTTP POST Web 请求。
using System.Net;
using System.Text;
using System.IO;
string url = "http://www.example.com" var request = (HttpWebRequest)WebRequest.Create(url);
var postData = "username=" + Uri.EscapeDataString("myUser");
postData += "&password=" + Uri.EscapeDataString("myPassword");
var data = Encoding.ASCII.GetBytes(postData);
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
request.ContentLength = data.Length;
using (var stream = request.GetRequestStream()) {
stream.Write(data, 0, data.Length);
}
var response = (HttpWebResponse)request.GetResponse();
在上面的代码中,我们创建了对 url
的 HTTP Web 请求 request
。我们使用 request.Method ="POST"
属性指定 request
是 POST 请求。(https://learn.microsoft.com/zh-cn/dotnet/api/system.uri.escapedatastring?view=net-8.0&viewFallbackFrom=net-5.0%2F)初始化我们要发送到 url
的数据 postData
,并将数据编码成字节变量 data
。我们使用 [request.ContentLength = data.Length
属性]指定要发送到 url
的数据的长度。最后,我们创建了一个流
并使用 stream.Write()
函数。我们使用 request.GetResponse()
函数并将其存储在 HTTPWebResponse
类对象 response
中。
使用 C# 中的 HttpClient
类发出 HTTP POST Web 请求
HttpClient
类提供了用于发送 HTTP 请求和接收 HTTP 响应的方法在 C# 中。我们可以使用 HttpClient.PostAsync(url, data)
函数发出 HTTP POST Web 请求,其中 url
是 URL,而 data
是我们要发送到 url
的数据。以下代码示例向我们展示了如何使用 C# 中的 HttpClient
类发出简单的 HTTP POST 请求。
using System.Net.Http;
readonly HttpClient client = new HttpClient();
var values =
new Dictionary<string, string> { { "username", "myUser" }, { "password", "myPassword" } };
string url = "http://www.example.com" var data = new FormUrlEncodedContent(values);
var response = await client.PostAsync(url, data);
在上面的代码中,我们创建了只读 HTTP 客户端 client
,并初始化了 url
。我们将要发送到 url
的数据存储在字典 values
中。然后,我们用 C# 中的 FormUrlEncodedContent()
函数将 values
转换为 application/x-www-form-urlencoded
类型。最后,我们使用 client.PostAsync(url, data)
函数发出了 HTTP POST Web 请求,并将来自 url
的响应存储在 response
变量中。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn