在 C# 中發出 HTTP POST Web 請求
-
使用 C# 中的
WebClient
類發出 HTTP POST Web 請求 -
使用 C# 中的
HttpWebRequest
類發出 HTTP POST Web 請求 -
使用 C# 中的
HttpClient
類發出 HTTP POST Web 請求
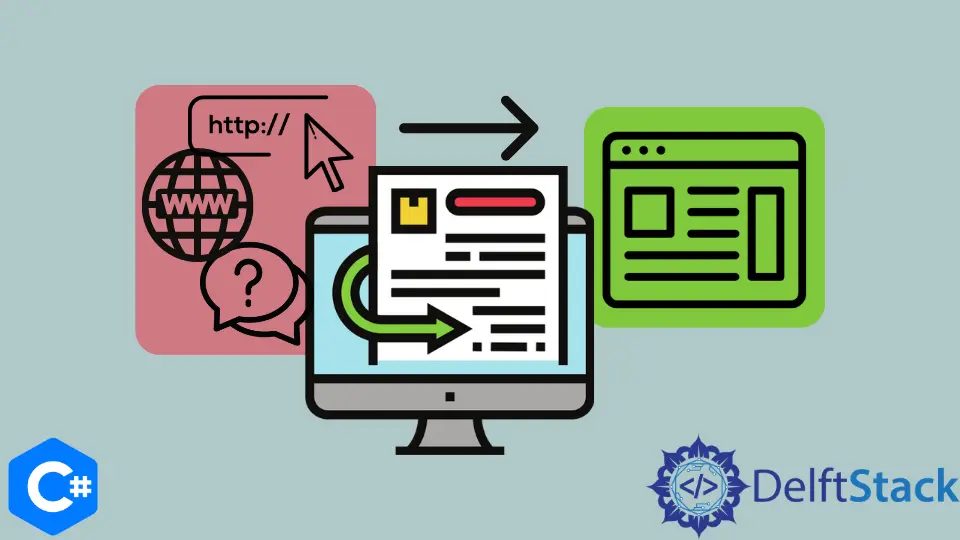
本教程將討論在 C# 中發出 HTTP POST Web 請求的方法。
使用 C# 中的 WebClient
類發出 HTTP POST Web 請求
WebClient
類在 C# 中提供了許多向 URL 傳送資料和從 URL 接收資料的方法。我們可以在 C# 中使用 WebClient.UploadValues(url, values)
函式的 WebClient
類來進行 HTTP POST 請求。以下程式碼示例向我們展示瞭如何使用 C# 中的 WebClient
類發出簡單的 HTTP POST Web 請求。
using System.Net;
using System.Collections.Specialized;
var wb = new WebClient() var data = new NameValueCollection();
string url = "www.example.com" data["username"] = "myUser";
data["password"] = "myPassword";
var response = wb.UploadValues(url, "POST", data);
在上面的程式碼中,我們建立了 Web 客戶端 wb
,用於將資料傳送到 url
。我們初始化要傳送到 URL
的 data
變數。通過在 wb.UploadValues(url, "POST", data)
函式的引數中指定 POST
,我們對 url
發出 HTTP POST Web 請求。url
的響應儲存在 reponse
變數中。
使用 C# 中的 HttpWebRequest
類發出 HTTP POST Web 請求
HttpWebRequest 類提供了在 C# 中使用 HTTP 協議與伺服器直接互動的方法。我們可以使用 HttpWebRequest.Method = "POST"
屬性來指定 HTTP Web 請求是 C# 中的 POST 請求。以下程式碼示例向我們展示瞭如何使用 C# 中的 HttpWebRequest
類發出簡單的 HTTP POST Web 請求。
using System.Net;
using System.Text;
using System.IO;
string url = "http://www.example.com" var request = (HttpWebRequest)WebRequest.Create(url);
var postData = "username=" + Uri.EscapeDataString("myUser");
postData += "&password=" + Uri.EscapeDataString("myPassword");
var data = Encoding.ASCII.GetBytes(postData);
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
request.ContentLength = data.Length;
using (var stream = request.GetRequestStream()) {
stream.Write(data, 0, data.Length);
}
var response = (HttpWebResponse)request.GetResponse();
在上面的程式碼中,我們建立了對 url
的 HTTP Web 請求 request
。我們使用 request.Method ="POST"
屬性指定 request
是 POST 請求。(https://learn.microsoft.com/zh-tw/dotnet/api/system.uri.escapedatastring?view=net-5.0/)初始化我們要傳送到 url
的資料 postData
,並將資料編碼成位元組變數 data
。我們使用 [request.ContentLength = data.Length
屬性]指定要傳送到 url
的資料的長度。最後,我們建立了一個流
並使用 stream.Write()
函式。我們使用 request.GetResponse()
函式並將其儲存在 HTTPWebResponse
類物件 response
中。
使用 C# 中的 HttpClient
類發出 HTTP POST Web 請求
HttpClient
類提供了用於傳送 HTTP 請求和接收 HTTP 響應的方法在 C# 中。我們可以使用 HttpClient.PostAsync(url, data)
函式發出 HTTP POST Web 請求,其中 url
是 URL,而 data
是我們要傳送到 url
的資料。以下程式碼示例向我們展示瞭如何使用 C# 中的 HttpClient
類發出簡單的 HTTP POST 請求。
using System.Net.Http;
readonly HttpClient client = new HttpClient();
var values =
new Dictionary<string, string> { { "username", "myUser" }, { "password", "myPassword" } };
string url = "http://www.example.com" var data = new FormUrlEncodedContent(values);
var response = await client.PostAsync(url, data);
在上面的程式碼中,我們建立了只讀 HTTP 客戶端 client
,並初始化了 url
。我們將要傳送到 url
的資料儲存在字典 values
中。然後,我們用 C# 中的 FormUrlEncodedContent()
函式將 values
轉換為 application/x-www-form-urlencoded
型別。最後,我們使用 client.PostAsync(url, data)
函式發出了 HTTP POST Web 請求,並將來自 url
的響應儲存在 response
變數中。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn