在 C# 中执行带参数的存储过程
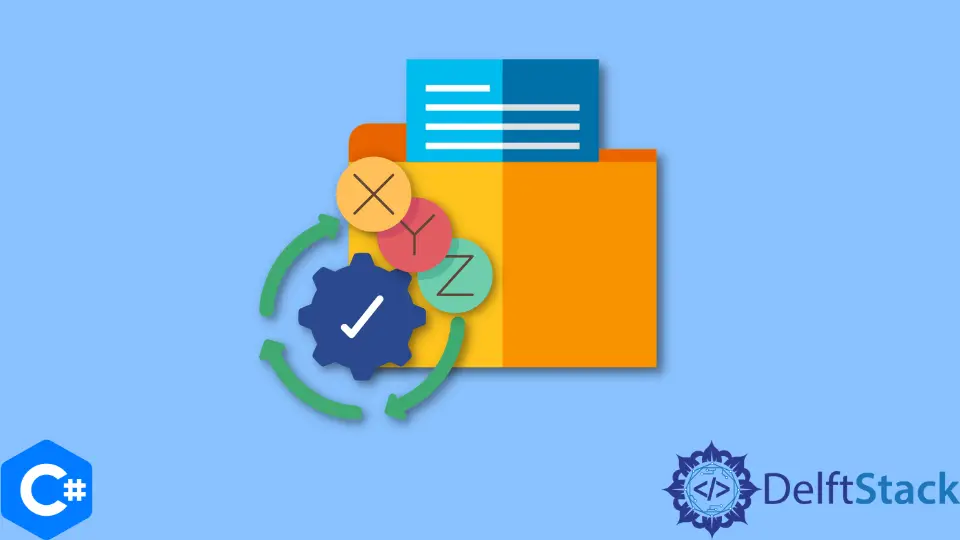
在本指南中,你将了解如何在 C# 中使用参数执行/调用存储过程,这意味着如何在存储过程中传递参数。
你将在这本紧凑的指南中从头到尾学习所有内容。让我们潜入吧!
在 C#
中使用参数执行存储过程
要了解如何在 C# 中调用带参数的存储过程,你需要了解有关存储过程的所有基础知识。
它们是什么,它们用于什么目的?他们的优势是什么?你如何创造和改变它们?
C#
中的存储过程
组装成单个执行计划的 Transact-SQL 语句的集合是一个存储过程。数据库服务器使用称为存储过程的代码块;它是一个预编译实体,编译一次,可以重复使用。
一系列 SQL 语句可以与存储过程一起顺序运行。我们必须使用过程参数来为过程提供数据。
存储过程使用参数映射的概念。前端参数和过程参数的名称、类型和方向必须匹配,前端参数长度必须小于或等于过程参数长度(只能映射参数)。
我们利用 return
语句从过程中返回任何值。
为什么我们在 C#
中使用存储过程
要从数据库中访问数据,你通常会创建 SQL 查询,例如 select
、insert
和 update
。如果你经常使用相同的查询,则将查询转换为存储过程是有意义的。
每次编写查询时,数据库都会对其进行解析。如果你为它创建了一个存储过程,它可以在解析一次后执行 N 次。
性能也可以通过存储的例程来提高。存储过程,即数据库服务器上的单个执行块,包含所有条件逻辑。
在 C# 中执行带参数的存储过程:代码示例
以下是在 C# 中执行带参数存储过程的步骤:
-
你需要做的第一件事是添加
using System.Data.SqlClient;
和使用 System.Data;
程序正上方的库。这些将允许你在 C# 程序中创建存储过程。 -
在下面的代码示例中,我们通过将数据库的路径传递给字符串来开始创建存储过程。
-
之后,我们使用
using()
方法创建Connection
对象con
并在其中传递路径字符串。 -
我们需要创建一个
SqlCommand
的对象。我们可以使用这个对象来利用 SQL 命令属性,例如Connection
。 -
之后,我们需要将命令类型指定为
StoredProcedure
。 -
要创建带参数的存储过程,我们需要创建一个
SqlParameter
对象并使用该对象来定义我们要传递的参数的名称、数据类型和值。 -
接下来我们需要做的是给它方向。我们可以将其用作输入、输出或两者兼而有之。
-
现在,通过使用
AddWithValue()
,传递你之前创建的参数对象。 -
请记住,所有这些都需要在
try-catch
块内完成。获取必须在catch
块内捕获的数据时可能会出现错误。
示例代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data.SqlClient;
using System.Data;
namespace Stored_procedure {
internal class Program {
static void Main(string[] args) {
// connection with sql
try {
// string for connection information
string con_str = "pass your connection path/information";
// create connection
using (SqlConnection con = new SqlConnection(con_str)) {
// create sqlcommand object
SqlCommand cmd = new SqlCommand();
cmd.CommandText = "Write the name of your stored prcedure";
cmd.Connection = con;
// specify the command type
cmd.CommandType = CommandType.StoredProcedure;
// create object of sqlparameter class
SqlParameter param = new SqlParameter {
// set the name of parameter
ParameterName = "Write your parameter name",
// set the type of parameter
SqlDbType = SqlDbType.Int, Value = 2,
// you can specify the direction of parameter input/output/inputoutput
Direction = ParameterDirection.InputOutput
};
// adding parameter in command
cmd.Parameters.AddWithValue(param);
// open connection of db
con.Open();
// executing the query
cmd.ExecuteNonQuery();
}
} catch (Exception e) {
Console.WriteLine(e.Message);
}
}
}
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn