在 C# 中執行帶引數的儲存過程
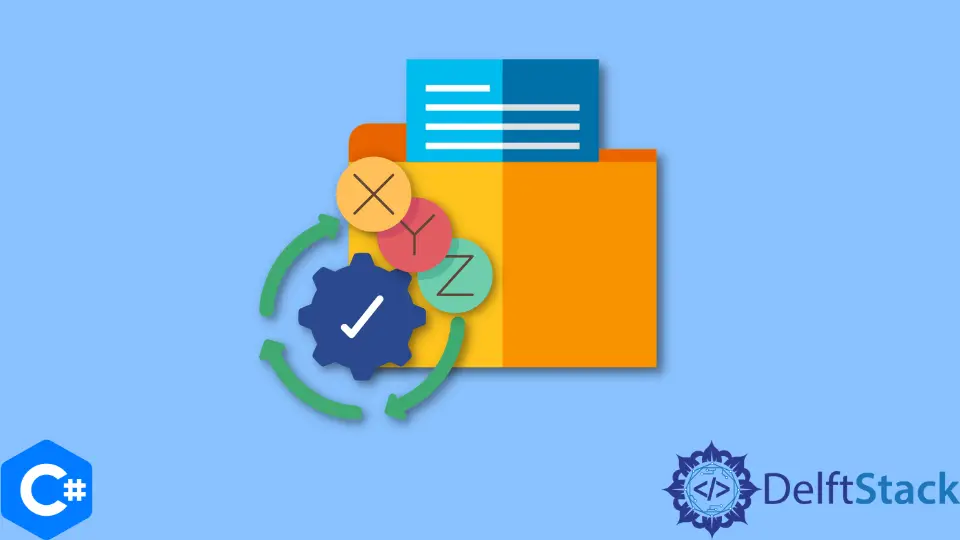
在本指南中,你將瞭解如何在 C# 中使用引數執行/呼叫儲存過程,這意味著如何在儲存過程中傳遞引數。
你將在這本緊湊的指南中從頭到尾學習所有內容。讓我們潛入吧!
在 C#
中使用引數執行儲存過程
要了解如何在 C# 中呼叫帶引數的儲存過程,你需要了解有關儲存過程的所有基礎知識。
它們是什麼,它們用於什麼目的?他們的優勢是什麼?你如何創造和改變它們?
C#
中的儲存過程
組裝成單個執行計劃的 Transact-SQL 語句的集合是一個儲存過程。資料庫伺服器使用稱為儲存過程的程式碼塊;它是一個預編譯實體,編譯一次,可以重複使用。
一系列 SQL 語句可以與儲存過程一起順序執行。我們必須使用過程引數來為過程提供資料。
儲存過程使用引數對映的概念。前端引數和過程引數的名稱、型別和方向必須匹配,前端引數長度必須小於或等於過程引數長度(只能對映引數)。
我們利用 return
語句從過程中返回任何值。
為什麼我們在 C#
中使用儲存過程
要從資料庫中訪問資料,你通常會建立 SQL 查詢,例如 select
、insert
和 update
。如果你經常使用相同的查詢,則將查詢轉換為儲存過程是有意義的。
每次編寫查詢時,資料庫都會對其進行解析。如果你為它建立了一個儲存過程,它可以在解析一次後執行 N 次。
效能也可以通過儲存的例程來提高。儲存過程,即資料庫伺服器上的單個執行塊,包含所有條件邏輯。
在 C# 中執行帶引數的儲存過程:程式碼示例
以下是在 C# 中執行帶引數儲存過程的步驟:
-
你需要做的第一件事是新增
using System.Data.SqlClient;
和使用 System.Data;
程式正上方的庫。這些將允許你在 C# 程式中建立儲存過程。 -
在下面的程式碼示例中,我們通過將資料庫的路徑傳遞給字串來開始建立儲存過程。
-
之後,我們使用
using()
方法建立Connection
物件con
並在其中傳遞路徑字串。 -
我們需要建立一個
SqlCommand
的物件。我們可以使用這個物件來利用 SQL 命令屬性,例如Connection
。 -
之後,我們需要將命令型別指定為
StoredProcedure
。 -
要建立帶引數的儲存過程,我們需要建立一個
SqlParameter
物件並使用該物件來定義我們要傳遞的引數的名稱、資料型別和值。 -
接下來我們需要做的是給它方向。我們可以將其用作輸入、輸出或兩者兼而有之。
-
現在,通過使用
AddWithValue()
,傳遞你之前建立的引數物件。 -
請記住,所有這些都需要在
try-catch
塊內完成。獲取必須在catch
塊內捕獲的資料時可能會出現錯誤。
示例程式碼:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data.SqlClient;
using System.Data;
namespace Stored_procedure {
internal class Program {
static void Main(string[] args) {
// connection with sql
try {
// string for connection information
string con_str = "pass your connection path/information";
// create connection
using (SqlConnection con = new SqlConnection(con_str)) {
// create sqlcommand object
SqlCommand cmd = new SqlCommand();
cmd.CommandText = "Write the name of your stored prcedure";
cmd.Connection = con;
// specify the command type
cmd.CommandType = CommandType.StoredProcedure;
// create object of sqlparameter class
SqlParameter param = new SqlParameter {
// set the name of parameter
ParameterName = "Write your parameter name",
// set the type of parameter
SqlDbType = SqlDbType.Int, Value = 2,
// you can specify the direction of parameter input/output/inputoutput
Direction = ParameterDirection.InputOutput
};
// adding parameter in command
cmd.Parameters.AddWithValue(param);
// open connection of db
con.Open();
// executing the query
cmd.ExecuteNonQuery();
}
} catch (Exception e) {
Console.WriteLine(e.Message);
}
}
}
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn