在 C# 中清除文本框
Muhammad Maisam Abbas
2024年2月16日
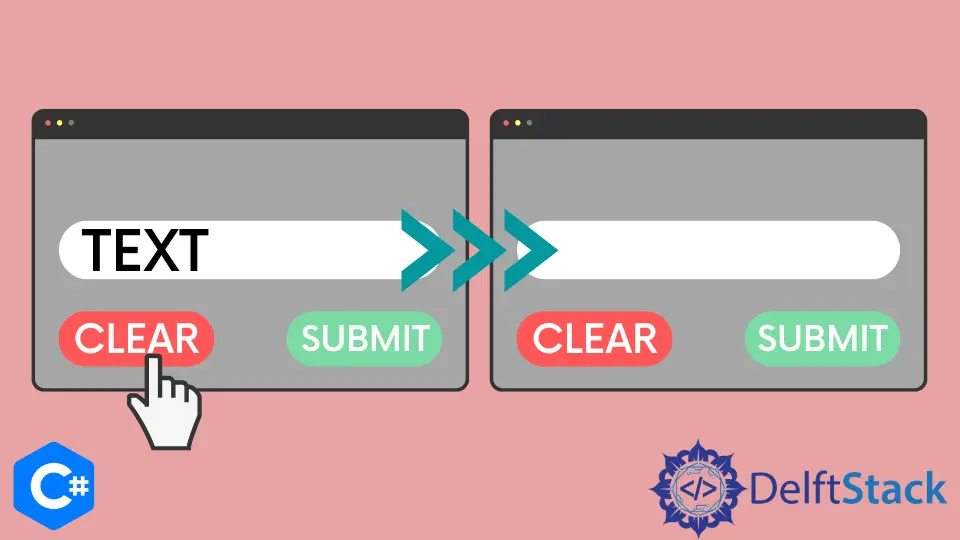
本教程将讨论清除 C# 中文本框的方法。
在 C# 中使用 String.Empty
属性清除文本框
C# 中 String.Empty
属性表示空字符串。它等效于 C# 中的""
。我们可以将 TextBox
的文本设置为等于 String.Empty
来清除文本框。下面的代码示例向我们展示了如何清除 C# 中带有 String.Empty
属性的文本框。
using System;
using System.Windows.Forms;
namespace check_if_textbox_is_empty {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
textBox1.Text = String.Empty;
}
}
}
在上面的代码中,我们通过使它等于 C# 中的 String.Empty
,清除了 textBox1
文本框中的所有文本。
在 C# 中使用 TextBox.Text=""
方法清除文本框
在以前的方法中,String.Empty
属性表示一个空字符串,并且等于""
。我们也可以通过使 TextBox.Text
属性等于""
来清除文本框。下面的代码示例向我们展示了如何使用 C# 中的 TextBox.Text
方法清除文本框。
using System;
using System.Windows.Forms;
namespace check_if_textbox_is_empty {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
textBox1.Text = "";
}
}
}
在上面的代码中,我们通过使它等于 C# 中的""
,清除了 textBox1
文本框中的所有文本。
使用 C# 中的 TextBox.Clear()
函数清除文本框
TextBox.Clear()
函数用于清除 C# 文本框中的所有文本。下面的代码示例向我们展示了如何使用 C# 中的 TextBox.Clear()
函数清除文本框。
using System;
using System.Windows.Forms;
namespace check_if_textbox_is_empty {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
textBox1.Clear();
}
}
}
在上面的代码中,我们使用 C# 中的 textBox1.Clear()
函数清除了 textBox1
文本框中的所有文本。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn