在 C# 中复制一个列表
Muhammad Maisam Abbas
2024年2月16日
Csharp
Csharp List
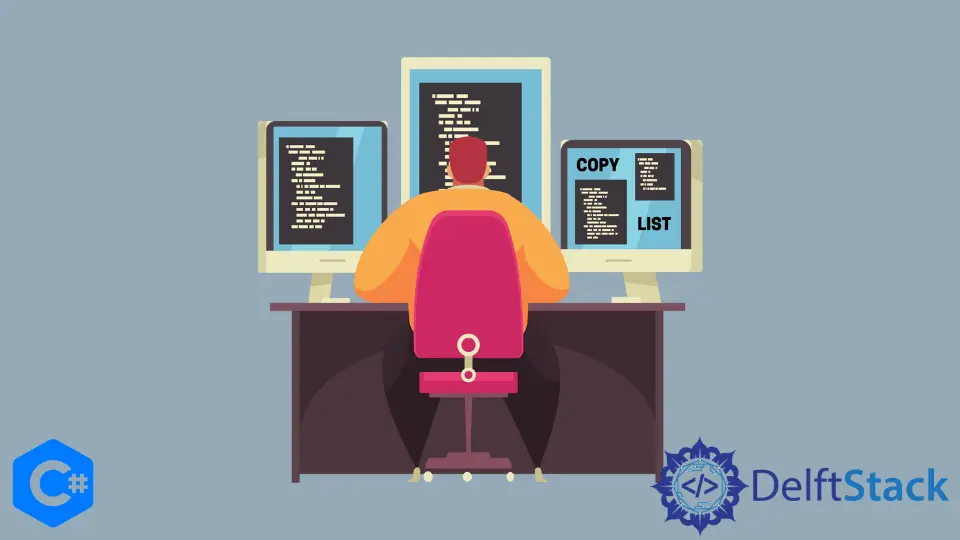
本教程将介绍在 C# 中复制列表的方法。
在 C# 中使用 Linq 复制列表
Linq 可以对 C# 中的数据结构执行类似 SQL 的查询。我们可以使用 Linq 与预定义的 item.Clone(/)
方法来创建一个列表的副本。请参见以下示例。
using System;
using System.Collections.Generic;
using System.Linq;
namespace copy_a_list {
static class Extensions {
public static List<T> Clone<T>(this List<T> listToClone)
where T : ICloneable {
return listToClone.Select(item => (T)item.Clone()).ToList();
}
}
class Program {
static void Main(string[] args) {
List<string> slist = new List<string> { "ABC", "DEF", "GHI" };
List<string> tlist = slist.Clone();
foreach (var t in tlist) {
Console.WriteLine(t);
}
}
}
}
输出:
ABC
DEF
GHI
我们创建了扩展功能 Clone()
以与通用列表一起使用。Clone()
函数使用 item.Clone()
函数为列表中的每个元素创建一个单独的副本,然后使用 C# 中的 ToList()
函数以列表的形式返回结果。在主函数中,我们初始化了字符串 slist
的列表,并将其克隆到另一个字符串 tlist
的列表中。我们可以将这种方法与值列表和引用列表一起使用。
使用 C# 中的列表构造器复制列表
创建列表副本的另一种更简单的方法是在 C# 中使用列表构造函数。我们可以将先前的列表传递给新列表的构造函数,以创建先前列表的副本。以下代码示例向我们展示了如何使用 C# 中的列表构造函数创建列表的单独副本。
using System;
using System.Collections.Generic;
using System.Linq;
namespace copy_a_list {
class Program {
static void method2() {}
static void Main(string[] args) {
List<string> slist = new List<string> { "ABC", "DEF", "GHI" };
List<string> tlist = new List<string>(slist);
foreach (var t in tlist) {
Console.WriteLine(t);
}
}
}
}
输出:
ABC
DEF
GHI
与以前的方法相比,此代码更简单,更易于理解。在上面的代码中,我们通过将 slist
作为 tlist
构造函数的参数传递,将列表 slist
单独创建到 tlist
中。我们只能将这种方法与值列表一起使用。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn