在 C# 中将字符串转换为双精度
-
在 C# 中使用
Convert.ToDouble()
函数将字符串转换为双精度数 -
在 C# 中使用
Double.Parse()
方法将字符串转换为双精度数 -
在 C# 中使用
Double.TryParse()
方法测试字符串并将其转换为 Double
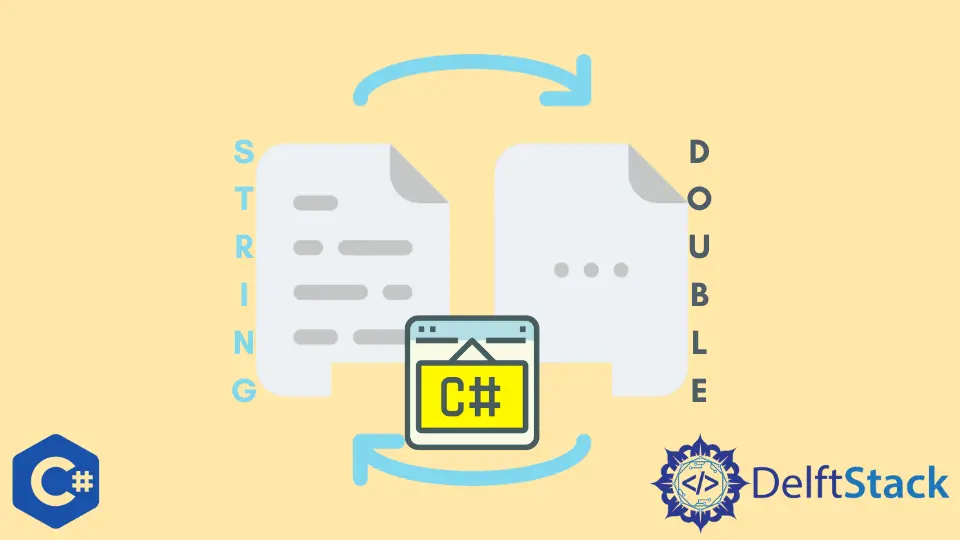
字符串用于存储文本,在 C# 中,字符串不仅仅是文本片段。它们是可以具有多种不同操作的对象。
Double 是具有 15 位十进制值的浮点数。它在 Float(另一种浮点数据类型)之后使用,但仅限于 7 个十进制数字。
通常,字符串可能包含文本中的数字,可能需要用作数字数据类型,例如: Int、Float、Double 等,用于算术运算。
本文将演示如何将字符串转换为双精度数。
在 C# 中使用 Convert.ToDouble()
函数将字符串转换为双精度数
我们在这里声明一个 String 变量,然后从 Console 类中调用 ToDouble()
函数将 String 类型转换为 Double
。
String word = "123.987324234234";
Console.WriteLine(Convert.ToDouble(word));
如果上述转换在极少数情况下产生错误,你可以尝试将字符串中的 .
改为 ,
。
例如,123.98732
将转换为 123,98732
。但是,发生这种情况的机会很少,如果出现错误,你最好尝试下面给出的不同选项。
在 C# 中使用 Double.Parse()
方法将字符串转换为双精度数
该方法继承自类 Double
,并作为 Console
类提供的方法的绝佳替代品。它解析字符串中的数字并提供 Double 数据类型结果。
String word = "123.9873242342342342342342342341";
Double number = Double.Parse(word);
Console.WriteLine(number);
在 C# 中使用 Double.TryParse()
方法测试字符串并将其转换为 Double
Parse()
方法附带一个类似的函数,称为 TryParse()
。一种方法,它还允许检查字符串是否为有效的 Double,以及返回已解析的 Double 数据类型。
TryParse()
是一个布尔方法,如果字符串是有效的 Double 则返回 True
,否则返回 False
。它还接受通过引用传递的第二个参数,以使用从字符串中解析的 Double 修改其值。
String word = "123.9873242342342342342342342341";
Double get_number;
if (Double.TryParse(word, out get_number)) {
Console.WriteLine("Valid Double. Number is : " + get_number);
} else {
Console.WriteLine("Invalid Double");
}
请记住始终使用 out
关键字并传递第二个参数。为什么?
C# 中的 out
关键字通过引用而不是值传递参数,允许函数修改其值并测试其有效性。
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub